Regular expression to return text between parenthesis
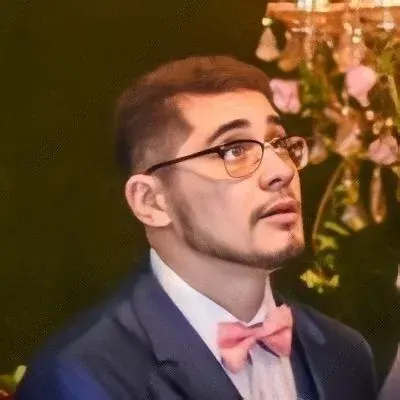
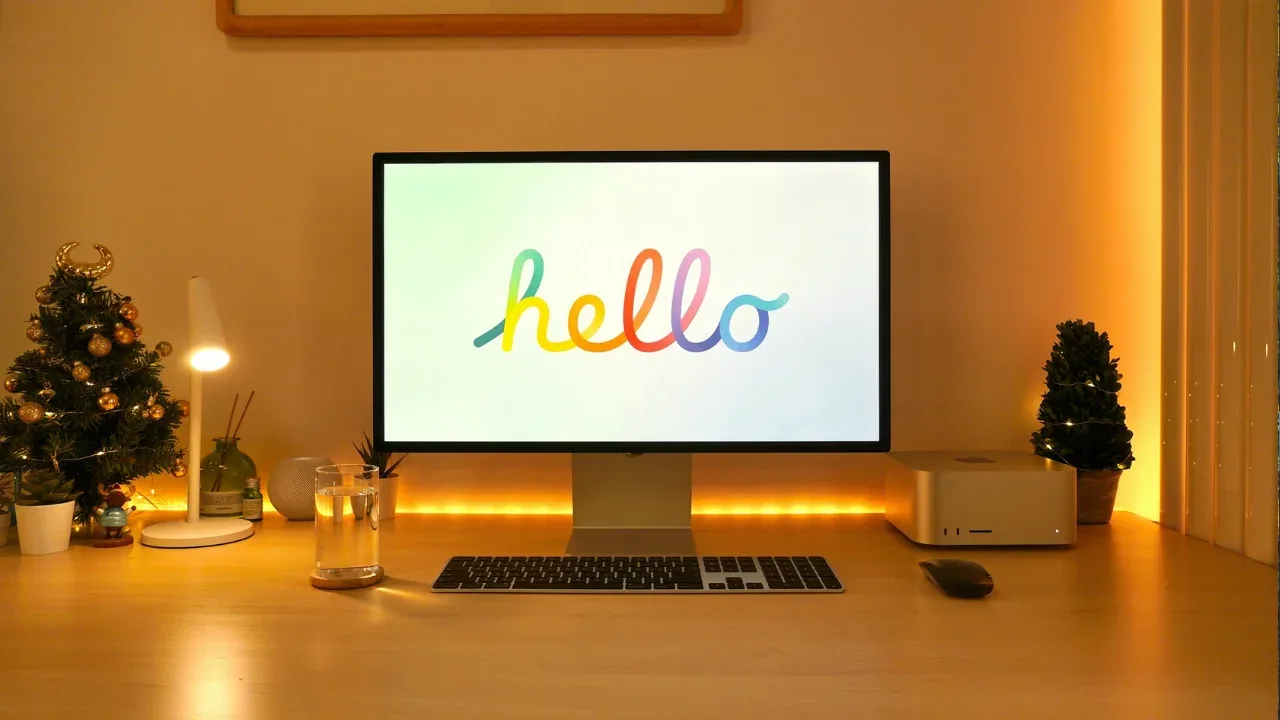
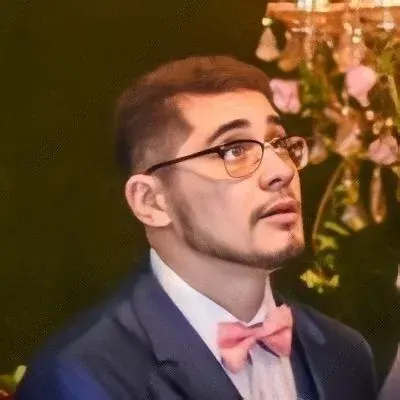
Tech Blog: Unleashing the Magic of Regular Expressions š©āØ
š Hey there tech enthusiasts! Today, we're diving into the world of regular expressions, unlocking a secret technique that will empower you to retrieve text between parentheses in an instant. Whether you're a beginner or a seasoned coder, this guide will equip you with the knowledge and tools you need to conquer this common challenge. Let's get started! š
The Challenge: Extracting Text between Parentheses
š§© Imagine you have a string that looks like this:
u'abcde(date=\'2/xc2/xb2\',time=\'/case/test.png\')'
š Your mission is to extract the contents within the parentheses and return it. In this case, we want to extract:
date='2/xc2/xb2',time='/case/test.png'
The Superpower: Regular Expressions
ā” Regular expressions, or regex, are a powerful tool for finding and manipulating text. With a bit of regex magic, you can easily solve this challenge.
ā Before we proceed, let's clarify that different programming languages might have slightly different regex syntax, but the core principles we'll discuss here remain the same.
The Solution: Mastering Regular Expressions
āļø To extract the text between parentheses, we can use the following regex expression:
\((.*?)\)
š Now let's break it down:
\(
: Matches the opening parenthesis "("(.*?)
: Matches any character (represented by "."), zero or more times (represented by "*?"), in a non-greedy manner (to capture the smallest possible match)\)
: Matches the closing parenthesis ")"
Putting it together, this expression allows us to extract the desired text between the parentheses.
Python Implementation: Code Like a Ninja šš¤
š» Here's an example of how you can use this regex expression in Python:
import re
input_string = u'abcde(date=\'2/xc2/xb2\',time=\'/case/test.png\')'
matches = re.findall(r'\((.*?)\)', input_string)
if matches:
extracted_text = matches[0]
print(extracted_text)
š Running this code will yield the following output:
date='2/xc2/xb2',time='/case/test.png'
Recap and Beyond: Expand Your Regex Toolkit š§°
š” Regular expressions are an essential tool for every coder. Armed with the knowledge we've shared today, you'll be able to handle similar challenges confidently and efficiently. Remember, practice makes perfect!
š Keep exploring and experimenting with different regex expressions to extract specific patterns that suit your needs. Regular expressions can be used to search for text, validate user input, perform substitutions, and so much more!
ā” If you're hungry for further regex knowledge, take a look at the official documentation of your programming language or check out some online regex tester tools such as RegExr or Regex101.
š Have a question, comment, or want to share your own regex tips and tricks? Don't be shy! Let's start a conversation in the comments below. Happy regex-ing! š¬šŖ
š„š Ready to take your coding skills to the next level? Join our newsletter to receive regular updates, coding challenges, and exclusive tutorials. Don't miss out on the tech adventure! Subscribe now! š©ā¤ļø
We hope you enjoyed this regex adventure! Feel free to share this article with your fellow developers and spread the regex happiness! š«āØ
Disclaimer: The examples in this article are specific to Python, but the regex principles can be applied across different programming languages.