Reading Excel File using Python, how do I get the values of a specific column with indicated column name?
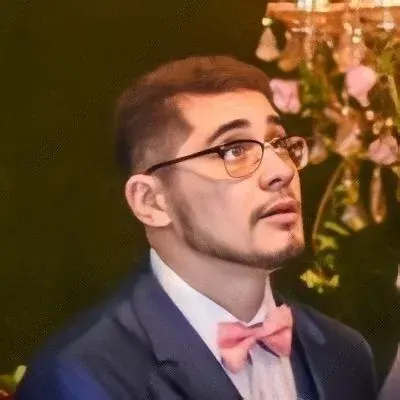
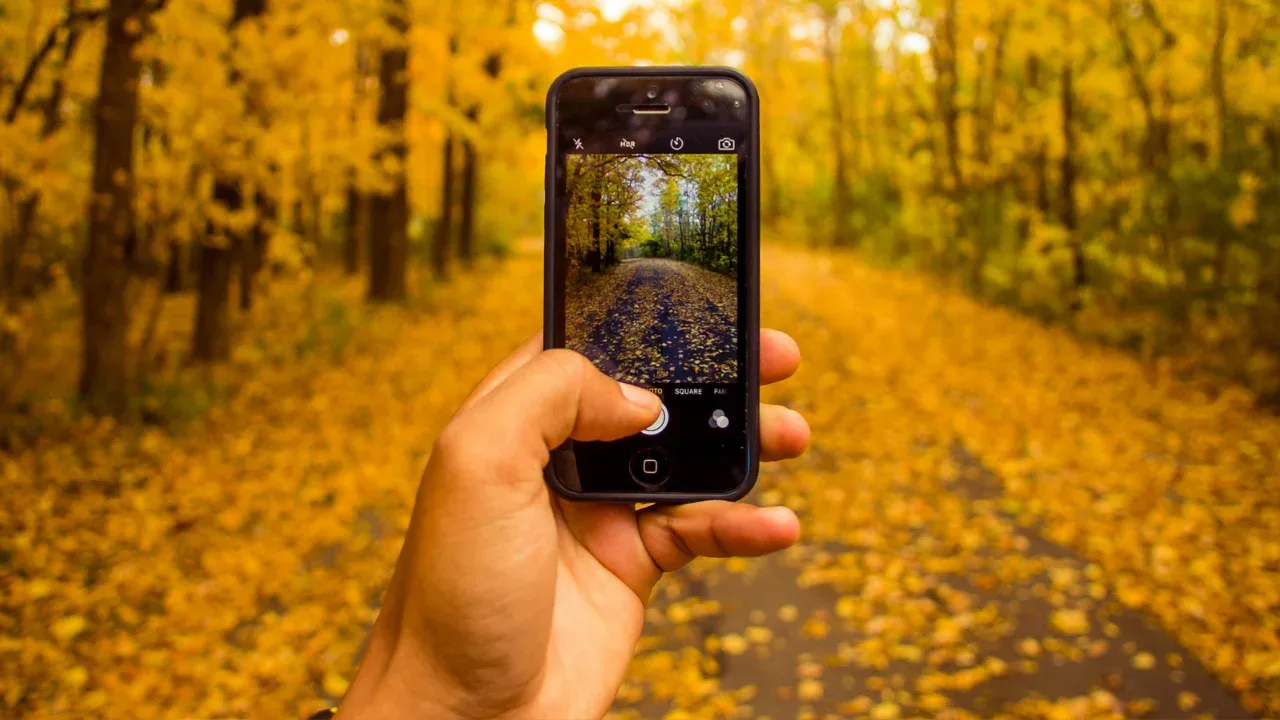
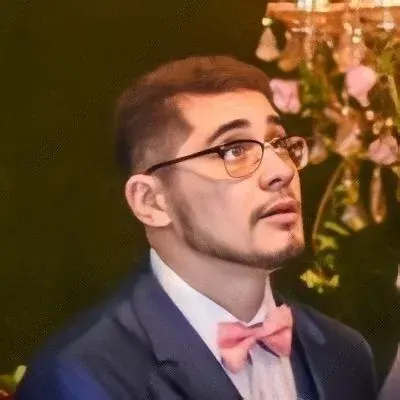
Reading Excel File using Python: Get Values of a Specific Column with Indicated Column Name
Are you struggling to extract the values of a specific column from an Excel file using Python? Don't worry, we've got you covered! In this blog post, we'll discuss a common problem faced by many Python developers and provide easy solutions to help you overcome this challenge.
The Challenge
Let's say you have an Excel file with the following data:
Arm_id DSPName DSPCode HubCode PinCode PPTL
1 JaVAS 01 AGR 282001 1,2
2 JaVAS 01 AGR 282002 3,4
3 JaVAS 01 AGR 282003 5,6
You want to extract the values of a specific column based on its name. However, the format of the desired columns may vary, depending on your configuration. For example, you may need to extract columns in the format Arm_id,DSPCode,Pincode
, but it could also change to DSPCode,Arm_id,Pincode
in the future.
The Solution
Here's a step-by-step guide to help you read the content of a specific column with the provided name:
Import the required libraries:
from xlrd import open_workbook
Define the columns you want to extract in the desired format. For example:
FORMAT = ['Arm_id', 'DSPName', 'Pincode']
Open the Excel file and iterate through each sheet:
wb = open_workbook('sample.xls')
values = []
for sheet in wb.sheets():
for row in range(sheet.nrows):
col_values = []
for col in range(sheet.ncols):
value = sheet.cell(row, col).value
try:
value = str(int(value))
except:
pass
col_values.append(value)
values.append(col_values)
Find the indices of the desired columns in
values[0]
, which contains the column names:
column_indices = [values[0].index(column_name) for column_name in FORMAT]
Extract the values of the desired columns in each row:
extracted_values = [[row[column_index] for column_index in column_indices] for row in values[1:]]
Voila!
extracted_values
now contains the values of the desired columns.
A Better Solution
While the above solution works, it can be improved. Instead of looping through each column repeatedly, you can use pandas, a powerful data analysis library, to simplify the process.
Here's an alternative solution using pandas:
Install the pandas library if you haven't already done so:
pip install pandas
Import the required libraries and read the Excel file into a pandas DataFrame:
import pandas as pd
df = pd.read_excel('sample.xls')
Use the
filter
method to extract the desired columns:
extracted_values = df.filter(FORMAT)
Conclusion
Reading the values of a specific column from an Excel file using Python doesn't have to be a daunting task. By following the steps outlined in this blog post, you can easily extract the desired columns and manipulate the data in various ways.
We hope this guide has been helpful and that you can now use this knowledge in your Python projects. If you have any further questions or would like to share your experiences, feel free to leave a comment or engage with our community.
Happy coding! 👩💻🚀
[INSERT CALL-TO-ACTION: Encourage reader engagement, e.g., "Share your experiences with reading Excel files in Python in the comments below!"]