Random string generation with upper case letters and digits
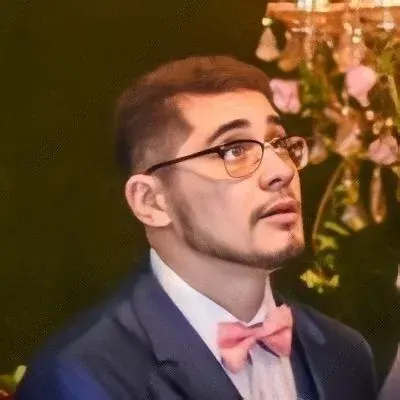
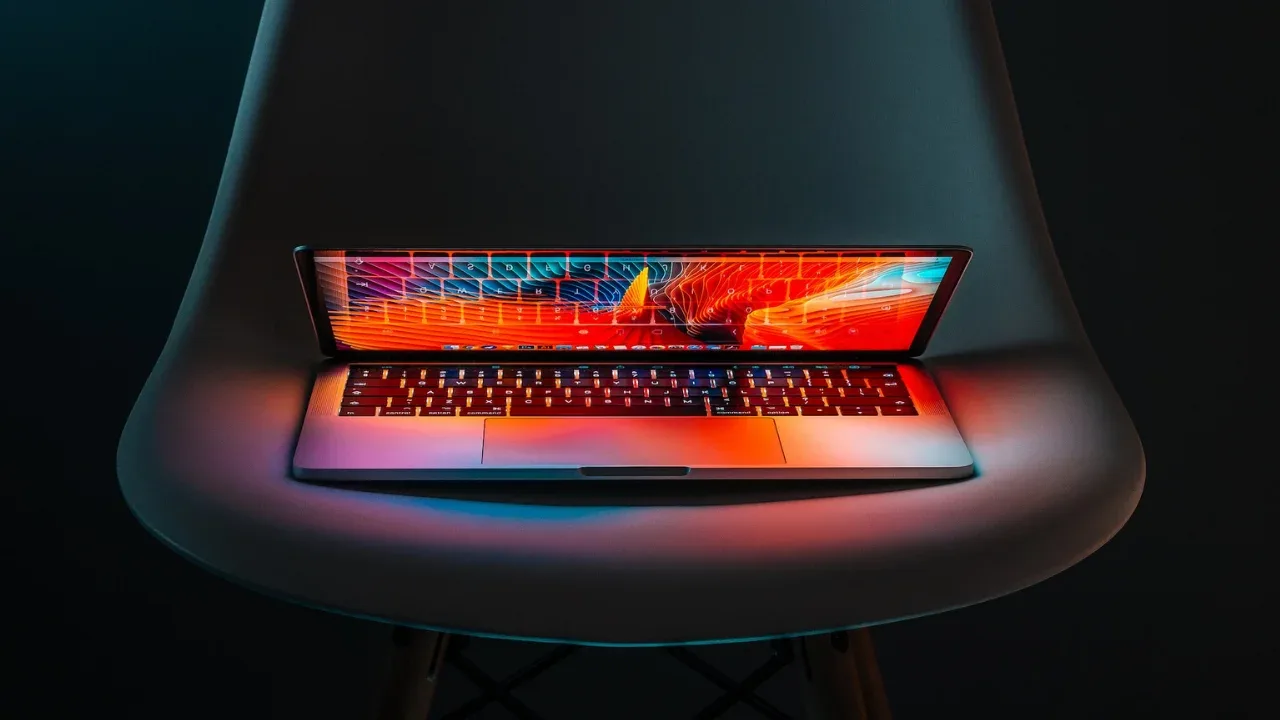
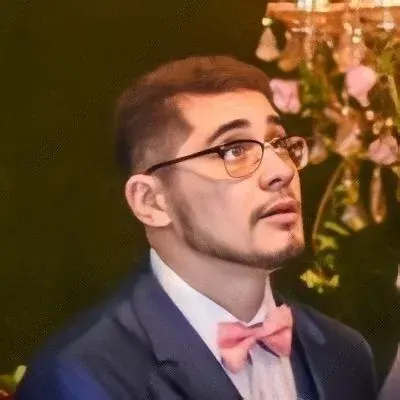
Generating Random Strings with Upper Case Letters and Digits: The Easy Way! 😎🔢🔠
Ever found yourself in need of creating a random string composed of uppercase letters and digits? Maybe you're building a password generator, or require a unique identifier for your users. Either way, fret not! We've got you covered with this handy guide that will walk you through the process, addressing common issues and providing easy solutions. Let's get started! 💪
The Problem: How to Generate a Random String with Upper Case Letters and Digits? 🤔
So you want a string that looks like 6U1S75
, 4Z4UKK
, or U911K4
? We gotchu! The main challenge lies in generating such a string that combines uppercase letters and digits, while also being completely random. But fear not, it's easier than you might think!
Solution 1: Utilize Random Number Generation and Character Mapping 🎲🔢🔡
One straightforward approach is to use a combination of random number generation and character mapping. Here's some sample code in Python to showcase the solution:
import random
import string
def generate_random_string(length):
characters = string.ascii_uppercase + string.digits
return ''.join(random.choice(characters) for _ in range(length))
random_string = generate_random_string(6)
print(random_string) # Outputs something like "6U1S75"
In this snippet, we utilize the random
module from Python's standard library. By creating a pool of characters comprised of uppercase letters (string.ascii_uppercase
) and digits (string.digits
), we can randomly select characters from this pool and create a string of the desired length. Easy peasy! 🙌
Solution 2: Utilize Cryptographically-Secure Random Number Generation 🌐🔒🔢🔡
If you require a higher level of security or randomness, it's recommended to use a cryptographically-secure random number generator. In Python, you can achieve this using the secrets
module introduced in Python 3.6. Check out this code snippet:
import secrets
import string
def generate_random_string(length):
characters = string.ascii_uppercase + string.digits
return ''.join(secrets.choice(characters) for _ in range(length))
random_string = generate_random_string(6)
print(random_string) # Outputs something like "6U1S75"
By replacing random.choice
with secrets.choice
, you ensure a more secure and unpredictable random string. This is particularly important when generating sensitive data like passwords or tokens. Better safe than sorry! 🔒💡
Time to Get Creative! ✨
Now that you know how to generate random strings with upper case letters and digits, the possibilities are endless! Explore different problem-solving scenarios or integrate this functionality into your own projects. Be it generating unique coupon codes, creating strong passwords, or assigning memorable usernames – you're all set! What cool applications can you envision? Share your thoughts and ideas in the comments below! 🤩💬
That's a wrap! You're now armed with the knowledge to generate random strings with upper case letters and digits like a pro! Whether you need it for security or fun, these solutions will have you covered. So go ahead and start incorporating random strings into your projects. Remember to double-check the security requirements and always challenge yourself to find new creative applications. Happy coding! 🚀💻