Random row selection in Pandas dataframe
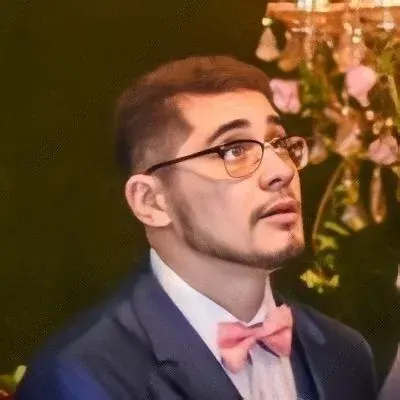
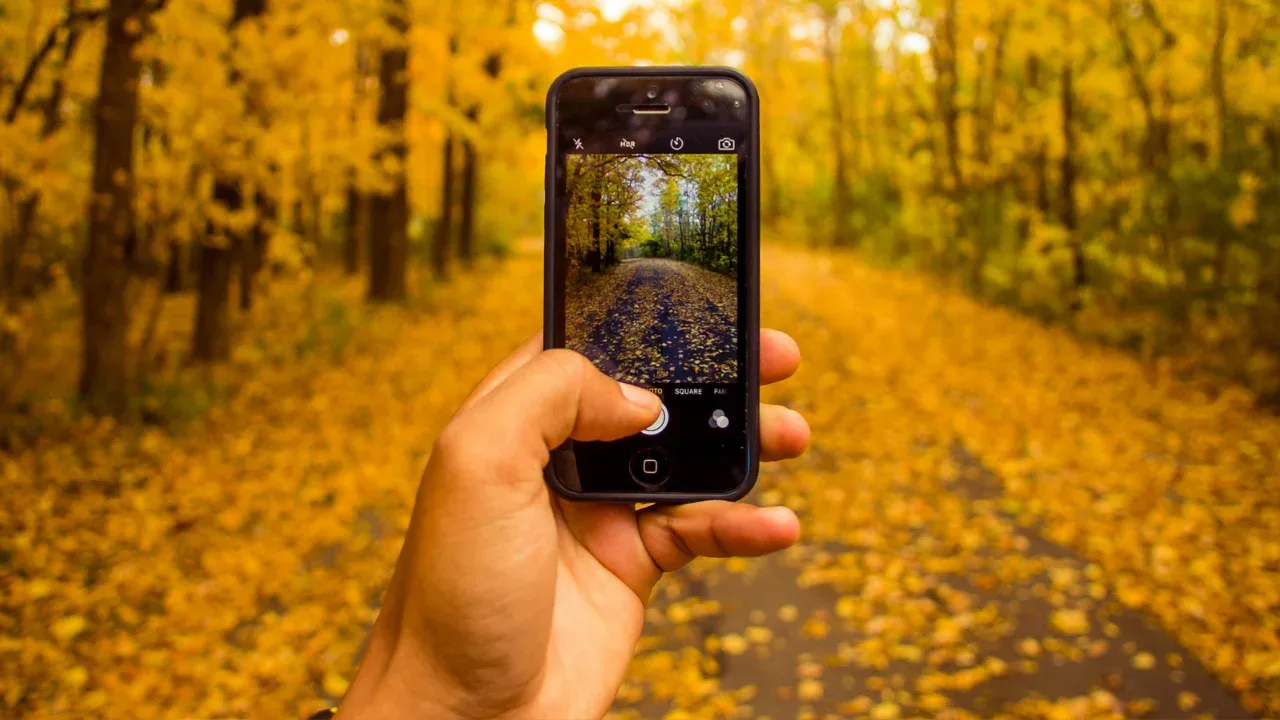
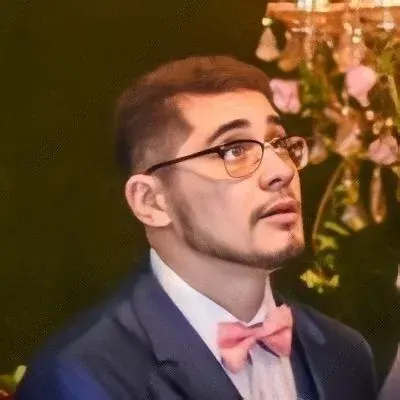
Random Row Selection in Pandas DataFrame: A Complete Guide
Are you looking to select random rows from a DataFrame in Pandas? 🤔 Well, you've come to the right place! In this blog post, we'll explore how to tackle this common task and provide you with easy solutions to achieve it. Let's dive in! 🚀
The Challenge 💡
As a Python data analyst or data scientist, you may often encounter the need to randomly select rows from a DataFrame. However, unlike R's some(x, n)
function, Pandas does not have a built-in method for this purpose. 😕
Easy Solutions 🎉
Thankfully, with the latest version of Pandas (version 20 and above), a handy method called sample()
has been introduced that makes random row selection a breeze. 🎊 Here's how you can use it:
df.sample(n)
By specifying the parameter n
, you can determine the number of random rows you want to select from the DataFrame df
. Amazing, right? 😄
Example Scenario 📚
Let's consider a practical example to solidify our understanding. Suppose we have a DataFrame called employees
that contains information about employees in a company. We want to randomly select 5 employees for an upcoming survey.
import pandas as pd
# Create the employees DataFrame (example data)
data = {
'Name': ['John', 'Emma', 'Michael', 'Sarah', 'David', 'Olivia', 'James', 'Sophia', 'Alexander', 'Isabella'],
'Age': [28, 32, 45, 36, 41, 29, 33, 49, 37, 31],
'Department': ['HR', 'Sales', 'IT', 'Finance', 'HR', 'Marketing', 'Finance', 'Sales', 'IT', 'Marketing']
}
employees = pd.DataFrame(data)
# Randomly select 5 employees
random_employees = employees.sample(5)
print(random_employees)
Output:
Name Age Department
0 John 28 HR
7 Sophia 49 Sales
5 Olivia 29 Marketing
8 Alexander 37 IT
4 David 41 HR
In the example above, we created a DataFrame called employees
with the employees' information. By using the sample()
method and specifying 5
as the number of rows we want to select, we obtained a new DataFrame random_employees
containing 5 randomly chosen employees. Neat, isn't it? 😎
Conclusion 🎯
Random row selection in Pandas DataFrame is no longer a challenge! With the introduction of the sample()
method in version 20 and above, you can easily select random rows with just a single line of code. 💪
So go ahead, leverage the power of Pandas and make your data analyses more exciting! Give the sample()
method a try in your next project and let us know how it worked out for you. 💬 We'd love to hear your experiences!
If you found this blog post helpful, don't forget to share it with your fellow Python enthusiasts. Together, we can simplify complex problems and empower the community. Happy coding! 🙌