Python xml ElementTree from a string source?
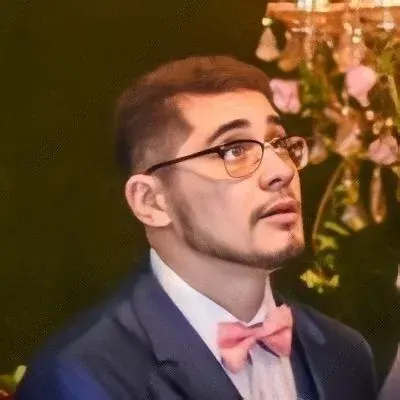
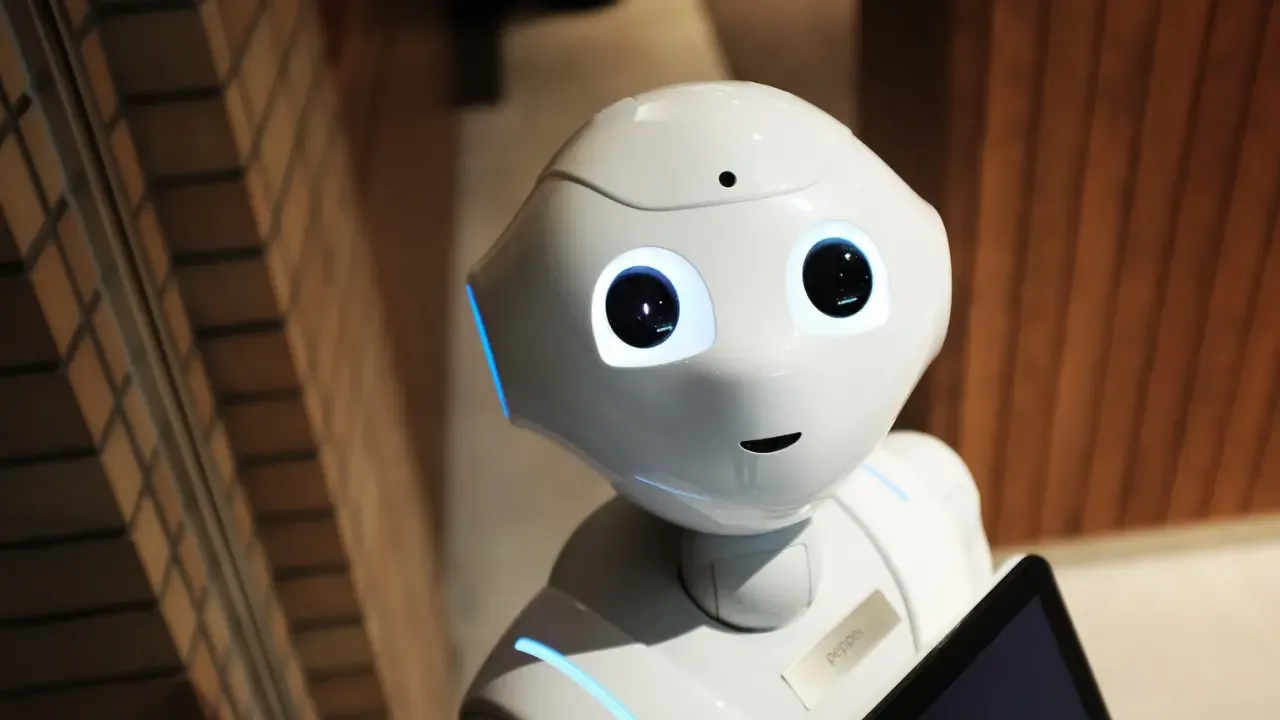
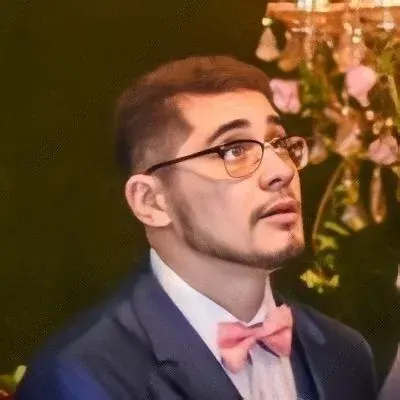
Python xml ElementTree from a string source?
š¤ You might be wondering if there's a way to use ElementTree
in Python to parse XML data from a string, without the need to write the string to a file and read it again. Well, the good news is: there is! š
The ElementTree
module in Python provides a simple and efficient way to parse and modify XML data. While its parse()
method primarily reads XML data from a file, it's definitely possible to use it to parse XML data from a string source as well. Let's dive into the details! š»š
The Problem:
š The parse()
method of ElementTree
normally expects a file-like object, but how do we use it if we already have the XML data in a string?
The Solution:
š The solution lies in the fromstring()
method provided by ElementTree
. This method allows us to directly parse XML data from a string. Here's an example of how to use it:
import xml.etree.ElementTree as ET
xml_data = "<root><child>Some text</child></root>"
tree = ET.ElementTree(ET.fromstring(xml_data))
# Now, you can access the root element of the parsed XML
root = tree.getroot()
# Do whatever you need to do with the parsed data
# For example, accessing elements and attributes:
child_text = root.find("child").text
print(child_text)
In this example, we create an ElementTree
object passing the parsed XML element from the fromstring()
method. Then, we can use this ElementTree
object to access the root element of the parsed XML data and perform any necessary operations.
š And that's it! You can now parse and manipulate XML data directly from a string with ElementTree
without the need for intermediate file operations.
But wait, there's more! š
The ElementTree
module provides many more useful functionalities for XML processing. Here are a few additional tips and tricks to enhance your XML parsing game:
- Parsing XML from URLs
š If you have XML data hosted at a URL, you can use the parse()
function directly with the URL as the parameter. For example:
import xml.etree.ElementTree as ET
url = "https://example.com/data.xml"
tree = ET.parse(url)
root = tree.getroot()
# Perform operations with the parsed XML
- Handling namespaces
š If you're working with XML that uses namespaces, you need to consider them when searching for elements or attributes. The ElementTree
module provides the register_namespace()
method to associate a namespace prefix with a URL. Here's a quick example:
import xml.etree.ElementTree as ET
ET.register_namespace("", "http://example.com/mynamespace")
xml_data = "<root xmlns='http://example.com/mynamespace'><child>Some text</child></root>"
tree = ET.ElementTree(ET.fromstring(xml_data))
# Now, you can search for elements in the namespace
root = tree.getroot()
child = root.find("{http://example.com/mynamespace}child")
print(child.text)
- Iterating over XML elements
š In some cases, you may need to iterate over all the elements in an XML document. The ElementTree
module provides an iter()
method that allows you to iterate over a specific element or all elements in the XML. Here's an example:
import xml.etree.ElementTree as ET
xml_data = "<root><child>Some text</child><child>Another text</child></root>"
tree = ET.ElementTree(ET.fromstring(xml_data))
root = tree.getroot()
for child in root.iter("child"):
print(child.text)
Share your experience!
š¢ We hope this guide helped you understand how to parse XML data from a string source using ElementTree
in Python. If you have any questions or would like to share your own tips and tricks for XML processing, we would love to hear from you! Leave a comment below and let's discuss. Happy coding! š»š