python pandas remove duplicate columns
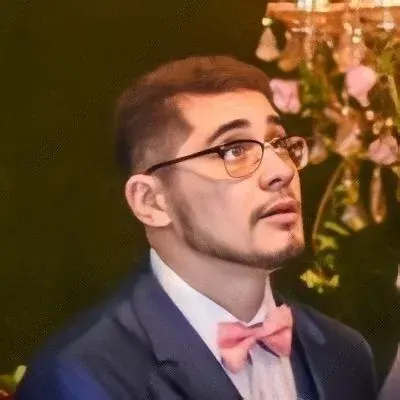
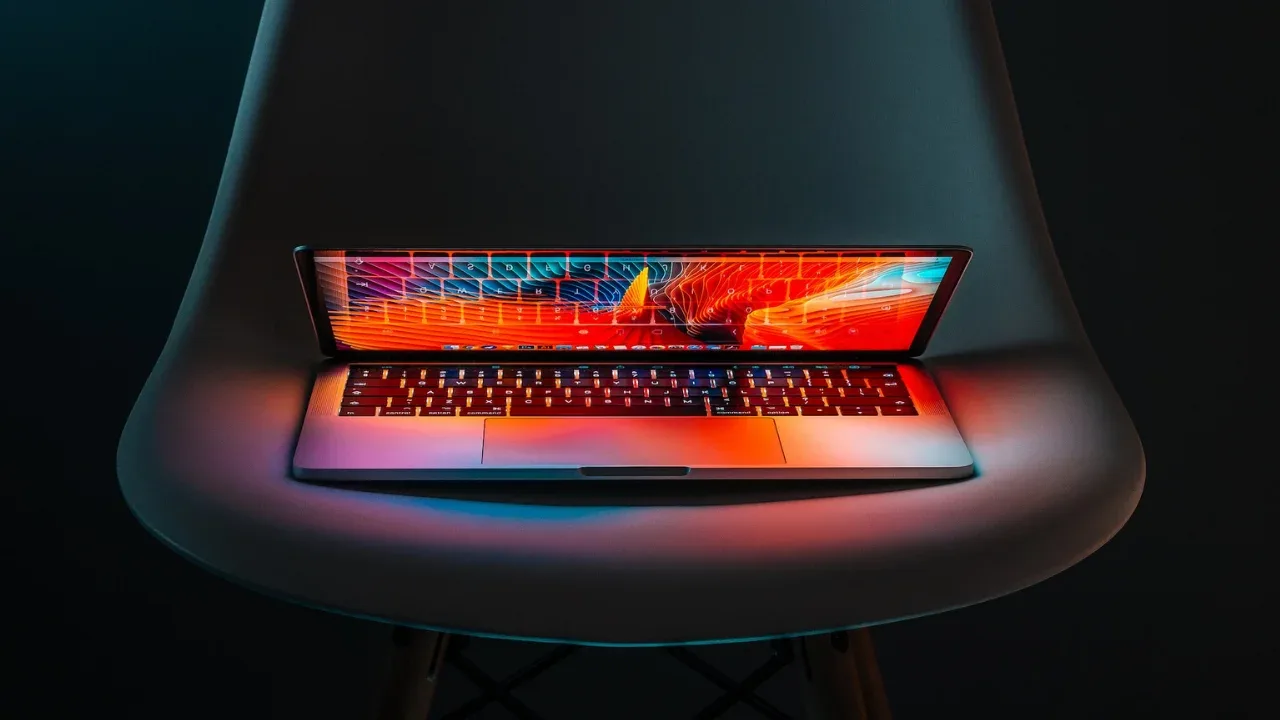
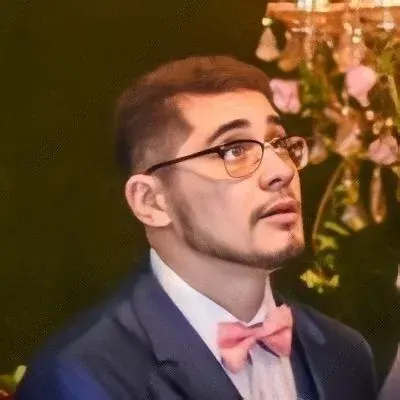
Python Pandas: Removing Duplicate Columns
Hey there, fellow Pandas enthusiast! π
In this blog post, we're going to tackle a common problem: removing duplicate columns from a Pandas DataFrame. Whether you're a newbie like our friend here or already a Pandas pro, we'll break it down for you and provide simple solutions. Let's dive in!
The Scenario
Our friend is reading a text file that contains duplicate columns into a DataFrame using the read_table()
function. The column names look like this:
Time, Time Relative, N2, Time, Time Relative, H2, etc...
As you can see, we have repeating columns like "Time" and "Time Relative" with the same data. Our objective is to remove the duplicate columns and get the following result:
Time, Time Relative, N2, H2
The Struggle
Our friend has made attempts at dropping or deleting the duplicate columns but encountered the following error message:
Reindexing only valid with uniquely valued index objects
Don't worry, our friend is not alone in this struggle. Many have faced similar issues when dealing with duplicate columns in Pandas. But fret not! We have some easy solutions for you.
π οΈ Solution 1: Using the T
and drop_duplicates()
Methods
One way to remove duplicate columns is by transposing the DataFrame and then applying the drop_duplicates()
method. Here's how you can do it:
df = df.T.drop_duplicates().T
This approach transposes the DataFrame, removes the duplicate rows (which are now columns), and transposes it back to the original shape. However, you might still run into the "uniquely valued index" error when using this method, especially if you have non-unique index values.
π οΈ Solution 2: Using the T
and groupby(level=0)
Methods
Another approach is to use the T
method along with the groupby(level=0)
method to remove duplicate columns. Here's the code:
df = df.T.groupby(level=0, axis=1).first().T
This method groups the columns by their labels (level=0) and takes the first occurrence of each group, effectively removing the duplicate columns. It should work even if you have non-unique index values.
π‘ Tip: Upgrade Your Pandas and Python Versions
Our friend mentioned using an older version of Pandas (0.9.0) and Python (2.7.3). While the solutions provided above should work with those versions, we highly recommend upgrading to the latest versions of both Pandas and Python to take advantage of bug fixes, new features, and better performance. You can visit the official Pandas and Python websites for more information on the latest releases.
Conclusion
Removing duplicate columns from a Pandas DataFrame might seem like a daunting task, but fear not! With the right tools and a little know-how, you can easily tackle this problem. We explored two simple and effective solutions using the T
method combined with either drop_duplicates()
or groupby(level=0)
. Remember to upgrade your Pandas and Python versions to enjoy the latest enhancements.
So go ahead, give these solutions a try, and let us know in the comments how it worked for you. Got any other Pandas conundrums you need help with? We're here to assist you!
Happy coding! ππΌπ