Python Pandas: Get index of rows where column matches certain value
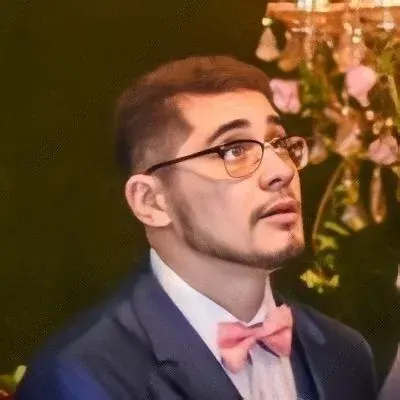
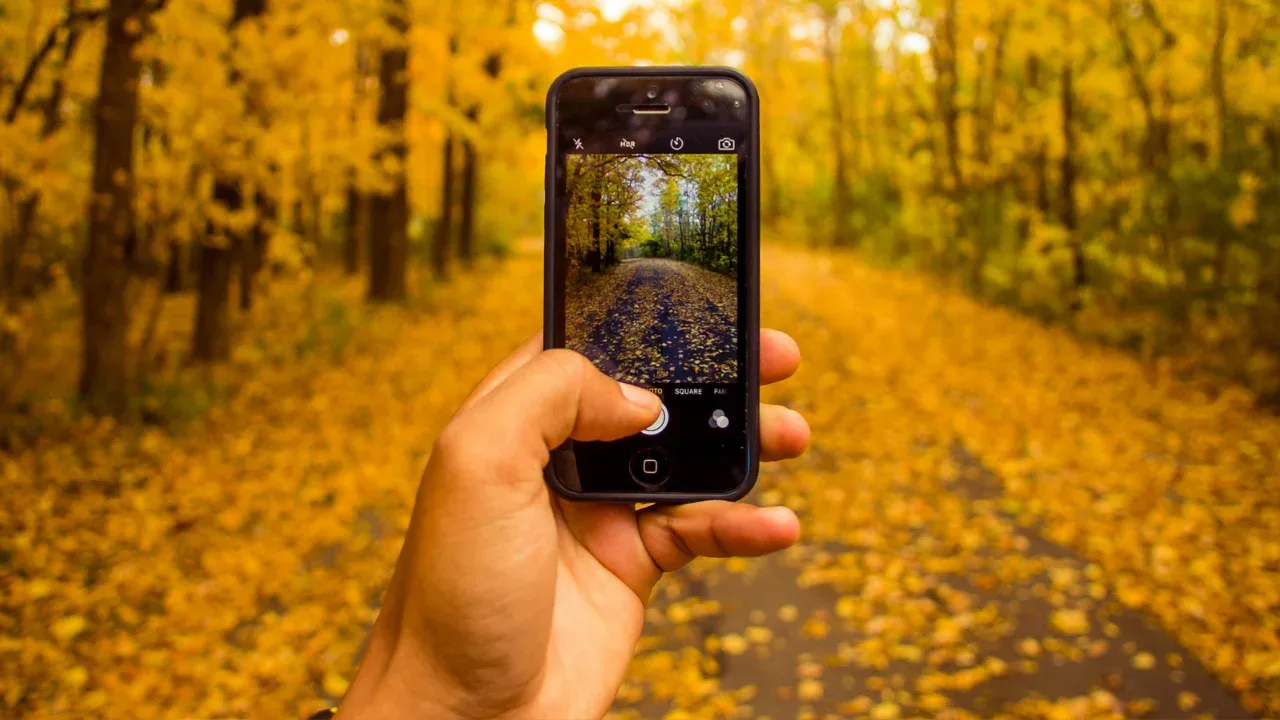
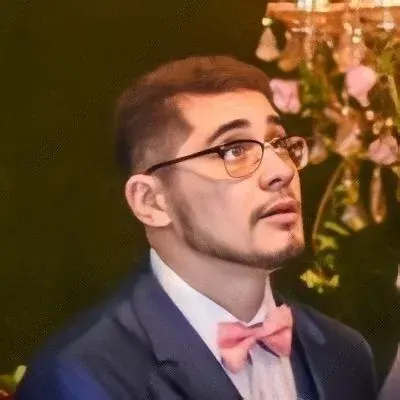
Python Pandas: Get index of rows where column matches certain value š»š¼
š Welcome to another blog post where we'll be diving into a common problem faced by Python Pandas users: getting the index of rows where a specific column matches a certain value. š¤
Let's begin by looking at the problem statement:
Given a DataFrame with a column "BoolCol", we want to find the indexes of the DataFrame in which the values for "BoolCol" are equal to True. š
The Context š
The author of the question mentioned that they currently have a working solution using iteration. Here's the code snippet they provided:
for i in range(100, 3000):
if df.iloc[i]['BoolCol'] == True:
print i, df.iloc[i]['BoolCol']
While this approach does the job, it's not the most efficient or "pandas-way" of doing it. So, after some research, the author found an alternative solution:
df[df['BoolCol'] == True].index.tolist()
However, there seems to be an issue with this solution, as the indexes obtained don't match when checked using df.iloc[i]['BoolCol']
. The result turns out to be False instead of True. š
The Correct Pandas Solution ā
To get the index of rows where the column matches a certain value, we can use the loc
or iloc
accessor along with a boolean condition:
df.loc[df['BoolCol'] == True].index.tolist()
This approach ensures that we are correctly filtering the DataFrame and obtaining the desired indexes. š
Example and Explanation š
Let's have a closer look at how this solution works with a simple example:
import pandas as pd
data = {'BoolCol': [True, False, True, False]}
df = pd.DataFrame(data)
indexes = df.loc[df['BoolCol'] == True].index.tolist()
print(indexes)
In this example, we create a DataFrame with a BoolCol
column consisting of True and False values. By using df.loc[df['BoolCol'] == True].index.tolist()
, we filter the DataFrame based on the condition df['BoolCol'] == True
and obtain the indexes where this condition is met.
The output of this example will be [0, 2]
, indicating that the rows with indexes 0 and 2 have a value of True in the BoolCol
.
š” Remember that indexing in Python starts from 0.
Engage with the Community š
Now that you know the correct way to get the index of rows where a column matches a certain value, why not share your thoughts, experiences, or alternative solutions with the community? Leave a comment below, and let's keep the conversation going! š
Conclusion š
In this blog post, we addressed the common problem of getting the index of rows where a column matches a certain value in Python Pandas. We explored the correct pandas solution using the loc
accessor and provided an example for better understanding. Remember to use this solution instead of iterating over the DataFrame, as it is more efficient and follows the pandas best practices.
Happy coding! šš