Python non-greedy regexes
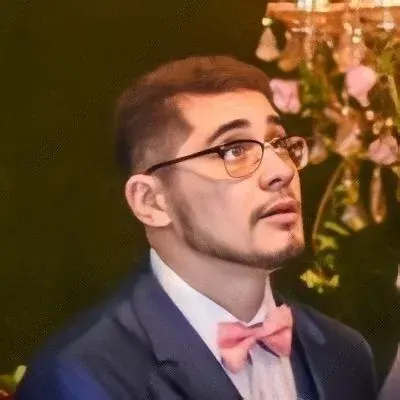
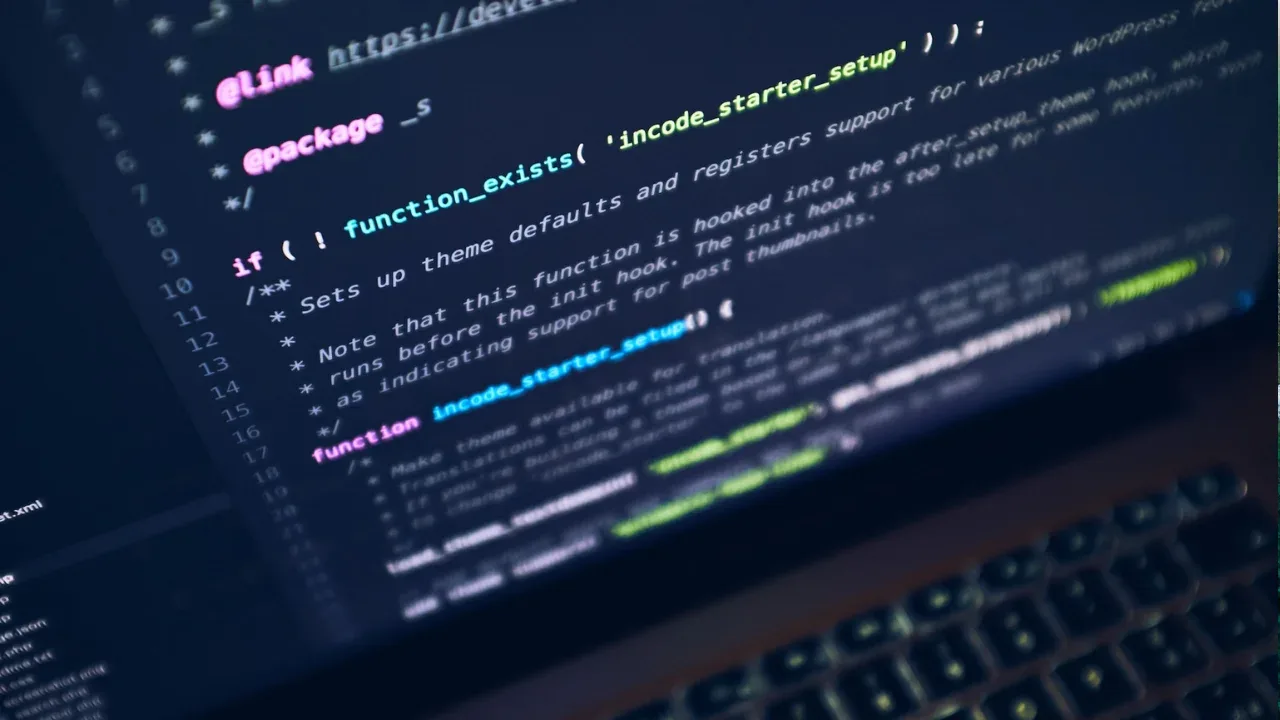
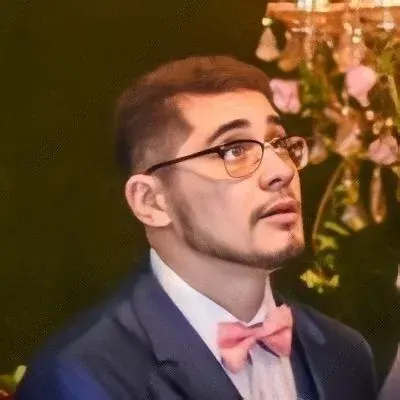
๐ Blog Post: Python Non-Greedy Regexes - Untangling the Mysteries ๐งต
Introduction: Welcome to the world of Python regexes! Regex is a powerful tool used to find and manipulate patterns in text. In this blog post, we'll tackle a common issue involving non-greedy regexes in Python. We'll learn how to make our regex match the smallest possible string, rather than the longest.
Understanding the Problem ๐:
Let's start by diving into the problem at hand. Our reader has a regex pattern like (.*)
, and they want to match only the innermost parentheses (b)
. However, by default, Python's regex engine is greedy, which means it tries to match the longest possible string. So, instead of matching (b)
, it matches b) c (d
.
Finding a Solution ๐ก:
Our reader is aware that using [^)]
(anything except a closing parenthesis) instead of .
can solve their problem in this specific case. However, they're looking for a more general solution that keeps their regex clean.
Regex Quantifiers ๐ถ๏ธ:
To achieve non-greedy matching in Python regex, we can use the ?
quantifier. When placed after a character or group, it instructs the regex engine to match as few repetitions as possible. Let's modify our original regex pattern to (.*?)
.
Let's Break it Down:
(
: Start of capturing group..
: Matches any character except a newline.*?
: Matches zero or more occurrences, as few as possible.)
: End of capturing group.
With this modification, the regex will now match the smallest possible string that satisfies the pattern. In our case, it would successfully match (b)
.
Call-To-Action: ๐ฃ Now that you've learned how to solve the non-greedy regex problem in Python, it's your turn! Put your newfound knowledge into practice and try solving similar challenges. Experiment with different patterns and quantifiers to refine your skills.
Conclusion:
Non-greedy regexes can be a real lifesaver when it comes to matching specific patterns in Python. By using the ?
quantifier, we can ensure that our regex matches the smallest possible string. This technique not only helps us solve the immediate problem of matching innermost parentheses but also keeps our regex clean and maintainable.
Remember, regex is a powerful tool, so wield it wisely! Happy coding! ๐ป
๐ Do you find Python non-greedy regexes helpful? Share your thoughts and experiences in the comments section below. Let's create a regex-loving community! ๐