Python list vs. array – when to use?
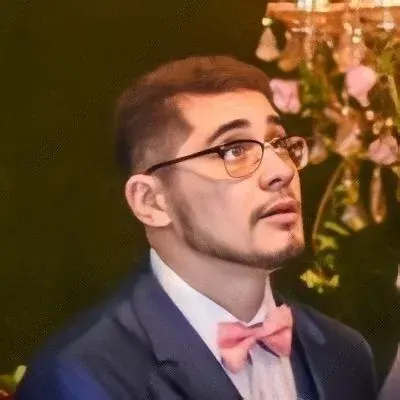
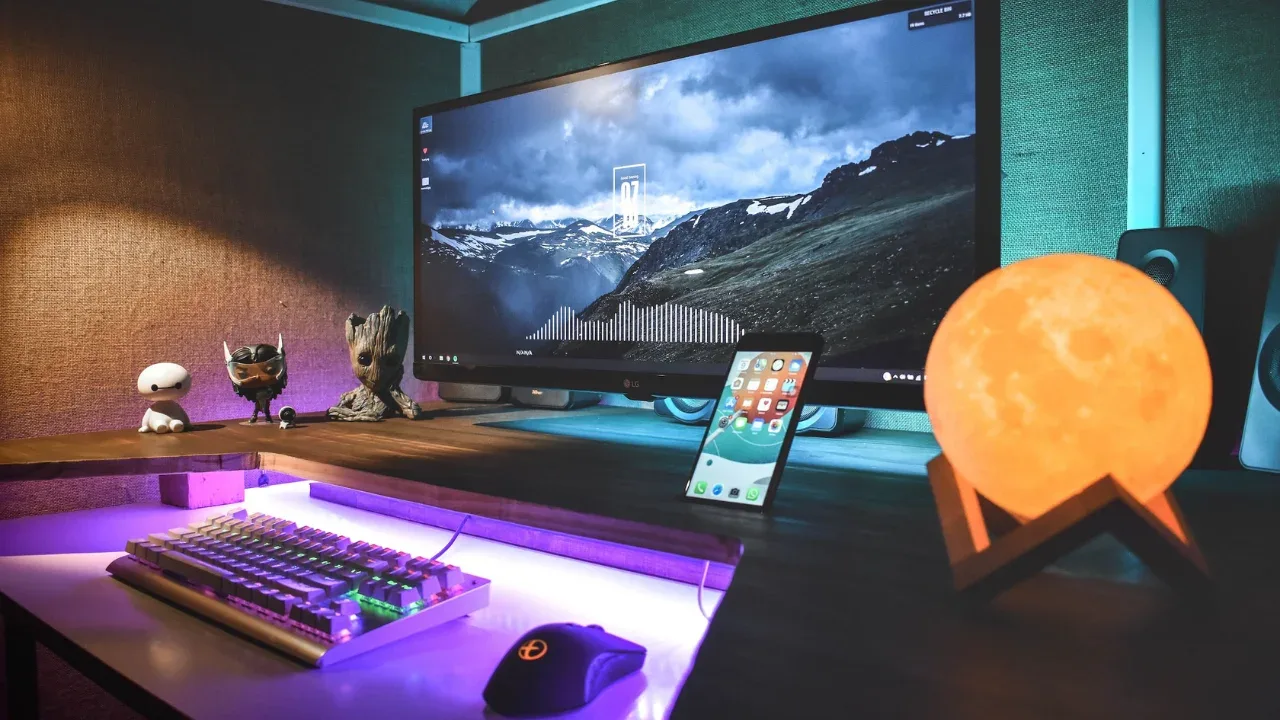
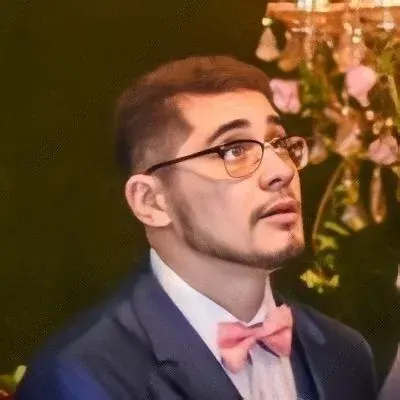
Python List vs. Array – When to Use? 😱📚
Are you new to Python and confused about when to use a list versus an array? Don't worry, you're not alone! 🤷♀️ In this blog post, we'll dive into the common issues and specific problems surrounding this question, provide easy solutions, and help you decide which data structure is the right choice for your needs.
Understanding the Difference 🤔
Before we dive deep into when to use a list or array, let's first understand the fundamental differences between them.
Python List: A list is a versatile built-in data structure that can hold elements of different types. Lists are dynamic, meaning they can grow or shrink as needed. You can easily add, remove, or modify elements within a list. Lists are created using square brackets []
and are indexed starting from zero.
Python Array: Arrays, on the other hand, are fixed-size sequences of elements of the same type. Unlike lists, arrays can only hold elements of the same data type. The array
module in the Python standard library provides an implementation of arrays. Arrays offer better performance and more memory-efficient storage for homogenous data.
When to Use a List? 📝
Lists are the go-to option for most use cases due to their flexibility and ease of use. Here are some scenarios where using a list is a good choice:
Mixed Data Types: If your elements vary in type, a list is the way to go. Lists don't impose any restrictions on the type of data they can store, allowing you to mix numbers, strings, booleans, and even other lists!
my_list = [1, 'hello', True, [2, 3, 4]]
Dynamic Structure: If you need a data structure that can change in size over time, a list is your best friend. You can add or remove elements using various built-in methods like
append()
,extend()
,insert()
, andremove()
.my_list = [1, 2, 3] my_list.append(4) # [1, 2, 3, 4]
Ease of Use: Lists are incredibly user-friendly. They provide a plethora of built-in operations and versatile indexing options. You can iterate over a list, slice it, concatenate it, and perform a wide range of operations effortlessly.
When to Use an Array? 📊
While lists are a fantastic all-purpose data structure, there are specific situations where arrays shine. Consider using an array when:
Homogenous Data: If your data consists of elements of the same type, using an array can boost performance and optimize memory usage. Arrays have a lower overhead compared to lists, making them ideal for large datasets requiring computational efficiency.
import array my_array = array.array('i', [1, 2, 3, 4, 5]) # 'i' specifies the type as integers
Working with Numeric Data: If you're dealing with numerical data and require mathematical operations, arrays have an advantage. The
array
module provides various methods for numeric computations, such as finding the minimum and maximum values, calculating sums, and more.import array my_array = array.array('d', [3.14, 2.71, 1.618]) # 'd' specifies the type as double (float) sum_of_array = sum(my_array) # 7.468
Conclusion and Call-to-Action 🎉
By now, you should have a clear understanding of when to use a list versus an array in Python. Remember, lists are excellent for their versatility and ease of use, making them the go-to choice for most scenarios. However, if you're dealing with homogeneous data or performance-critical operations, arrays are the way to go.
If you found this guide helpful or have any questions, feel free to leave a comment below. We'd love to hear from you! Happy coding! 😄🐍