Python JSON serialize a Decimal object
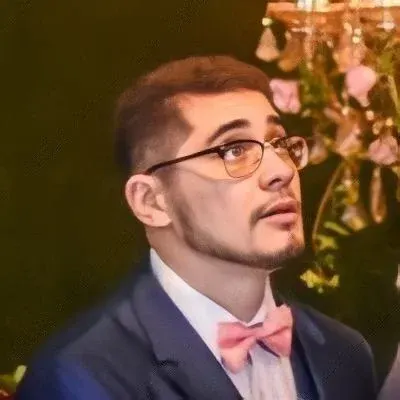
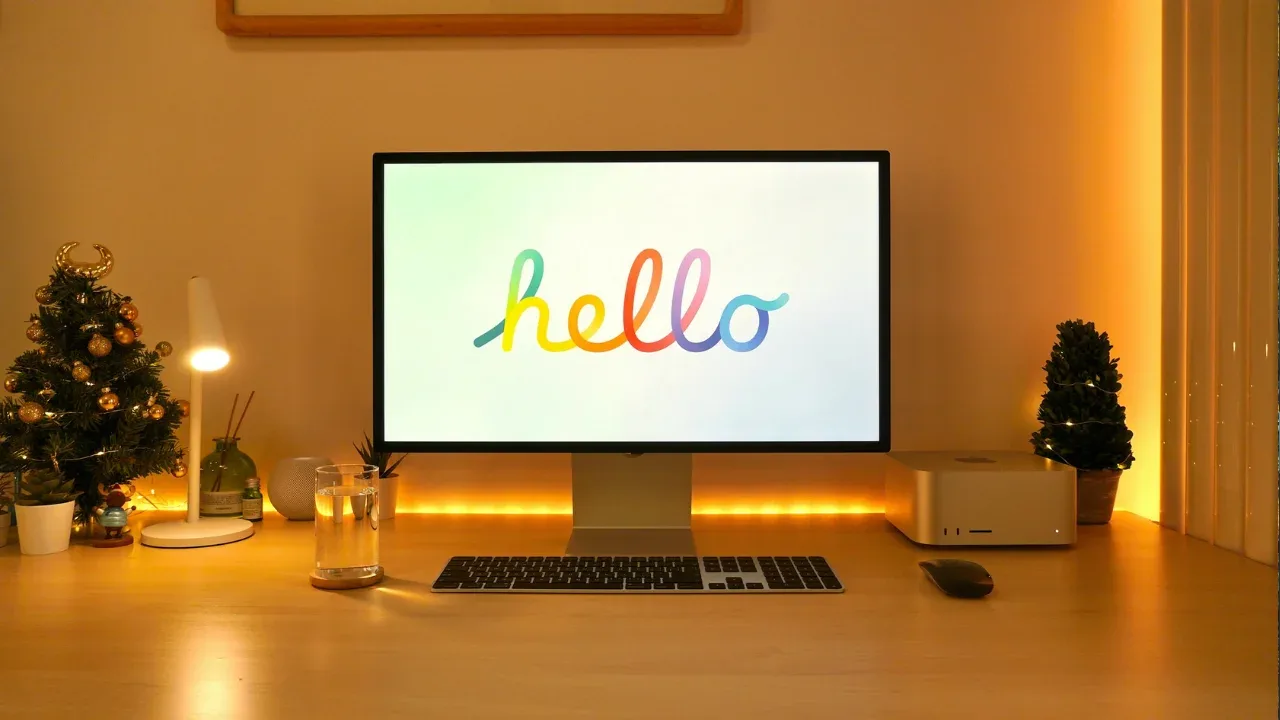
Python JSON Serialize: Dealing with Decimal Precision in JSON Encoding
š„ļø Hey there tech enthusiasts! Welcome back to our blog! Today, we'll tackle a common issue that many Python developers face when serializing Decimal objects to JSON. š”
Imagine this scenario: You have a Decimal object, let's call it Decimal('3.9')
, as part of an object you want to encode to a JSON string. However, you want the JSON string to represent the Decimal as a float without precision loss. Your desired output looks like this: {'x': 3.9}
. Sounds like a simple task, right? Well, not exactly.
The Problem š¤
You might be tempted to use the json.dumps()
function to serialize the object. However, if you do that directly, you'll end up with a precision loss, resulting in a JSON string like this: {'x': 3.8999999999999999}
. Definitely not what we're aiming for! š«
So, how can we convert a Decimal object to a JSON float without sacrificing precision? It's time to reveal the easy solutions!
Easy Solution 1: Custom JSON Encoder šļø
One way to solve this problem is by creating a custom JSON encoder that handles Decimal objects properly. Here's an example of how you can implement it:
import json
from decimal import Decimal
class DecimalEncoder(json.JSONEncoder):
def default(self, o):
if isinstance(o, Decimal):
return float(o)
return super().default(o)
In this code snippet, we extend the JSONEncoder
class and override the default()
method. Inside the method, we check if the object is an instance of Decimal. If it is, we convert it to a float using the float()
function. This ensures that the resulting JSON string represents the Decimal object without precision loss.
To use our custom encoder, you need to pass it as the cls
argument to the dumps()
function:
decimal_obj = Decimal('3.9')
json_str = json.dumps({'x': decimal_obj}, cls=DecimalEncoder)
print(json_str)
Running this code will give you the desired output: {'x': 3.9}
.
Easy Solution 2: Convert to String š¤
If creating a custom JSON encoder seems like too much work for your use case, another straightforward solution is to convert the Decimal object to a string before serializing it. Here's an example:
import json
from decimal import Decimal
decimal_obj = Decimal('3.9')
decimal_obj_str = str(decimal_obj)
json_str = json.dumps({'x': decimal_obj_str})
print(json_str)
In this approach, we simply convert the Decimal object to a string using the str()
function before passing it to json.dumps()
. This way, the JSON encoder treats it as a regular string, preserving the precise representation.
When you run this code, the output will once again be {'x': 3.9}
. Problem solved! š
Call-to-Action: Share Your Thoughts! š£
Dealing with precision loss when serializing Decimal objects to JSON can be a headache. But fear not, my fellow Pythonistas! With these easy solutions under your belt, you can now confidently tackle this problem in no time.
We hope you found this guide helpful! Let us know in the comments which solution you prefer and if there are any other Python topics you'd like us to explore next. Don't forget to share this post with your friends who might also find it useful! Happy coding! šš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
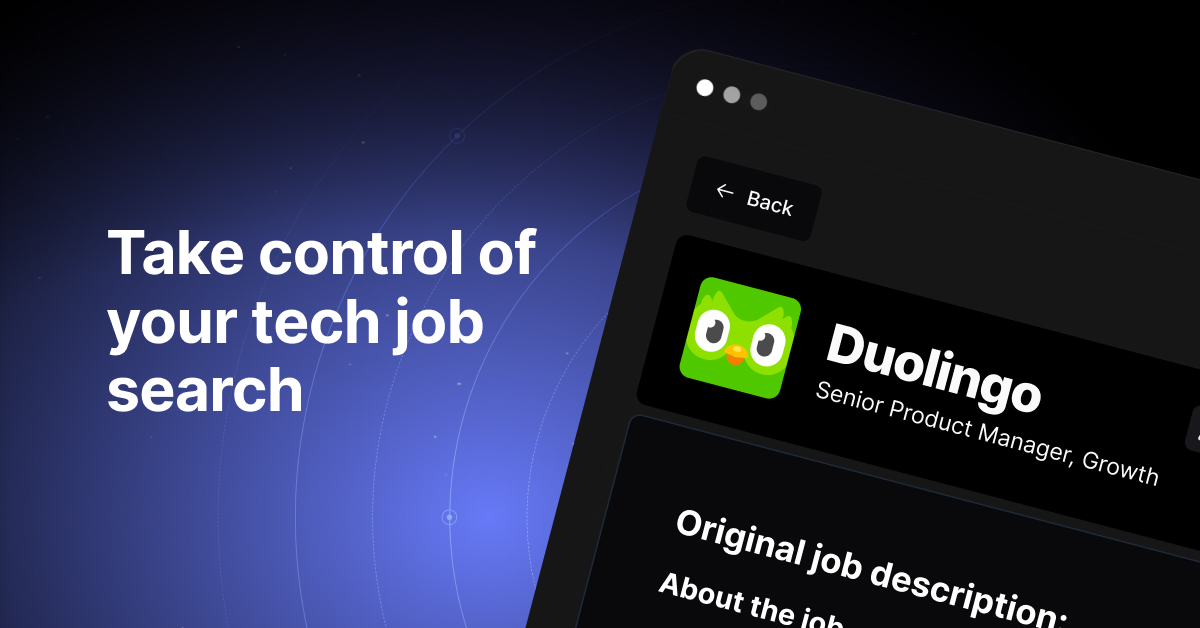