Python extract pattern matches
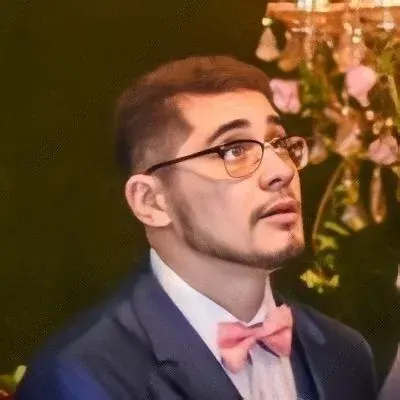
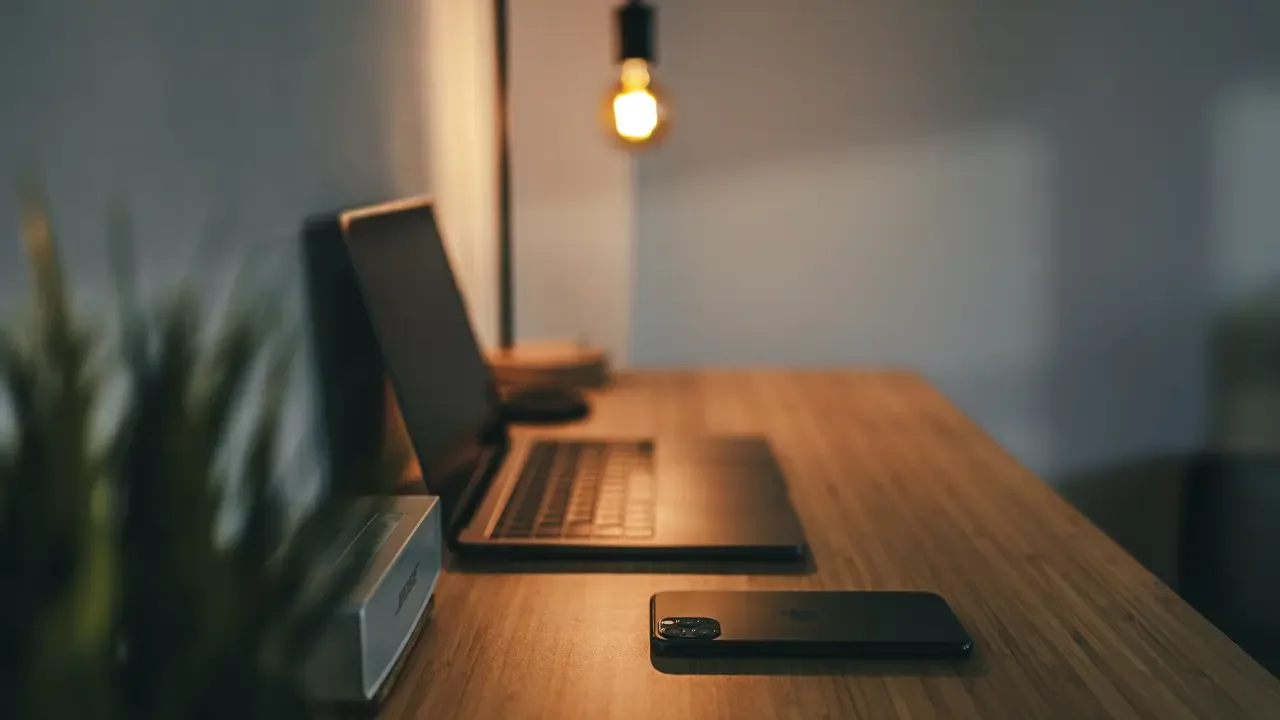
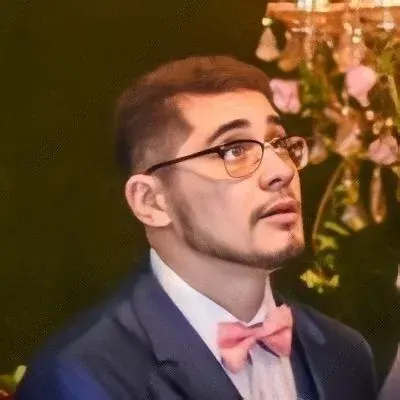
😎 Python Extract Pattern Matches: A Handy Guide
If you've ever found yourself trying to extract specific patterns from a string using regular expressions in Python, you're not alone. It can be a bit tricky, but fear not! In this guide, we'll walk you through common issues and provide easy solutions to help you extract those sought-after pattern matches. 🎯
The Scenario
Let's dive right into an example to help illustrate the problem. Imagine you have a string that looks like this:
someline abc
someother line
name my_user_name is valid
some more lines
Your mission is to extract the word my_user_name
from this string. 🕵️♀️
The Approach
To accomplish this task, we'll be using the re
module in Python, which provides support for regular expressions.
Here's a step-by-step breakdown of how we can achieve our goal:
Import the
re
module:import re
Create a regex pattern:
p = re.compile("name .* is valid", re.flags)
In this case, our pattern is
"name .* is valid"
. The.*
is a wildcard that matches any character, while the actual value we want to capture ismy_user_name
.Use
p.match()
to find the pattern in the string:match = p.match(s)
The
p.match()
function returns a match object if the pattern is found in the string, orNone
if it is not found.
Extracting the Matched Pattern
Now, the most exciting part: how do we extract my_user_name
from the match object? 🤔
To obtain the desired pattern match, we'll leverage the group()
method on the match object. Here's how to do it:
matched_string = match.group()
And just like that, matched_string
will contain the extracted pattern, which in our case is my_user_name
. 🎉
Wrap Up and Your Turn!
Congratulations! You've learned how to extract pattern matches using regular expressions in Python. 🥳
Now it's time for some hands-on practice! Take the concepts you've learned here and apply them to your own projects. Don't be afraid to experiment and tweak the patterns to match your specific needs!
Have any questions or got stuck somewhere? Let us know in the comments below. We're here to help you out! 💪
Happy coding! 👩💻👨💻