Python dataclass from a nested dict
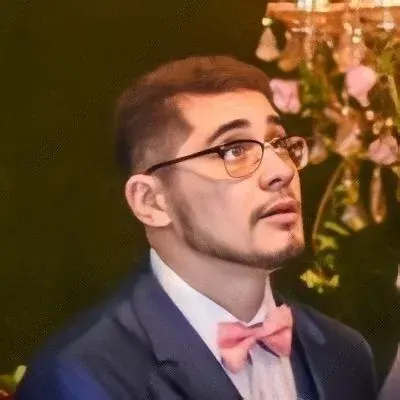
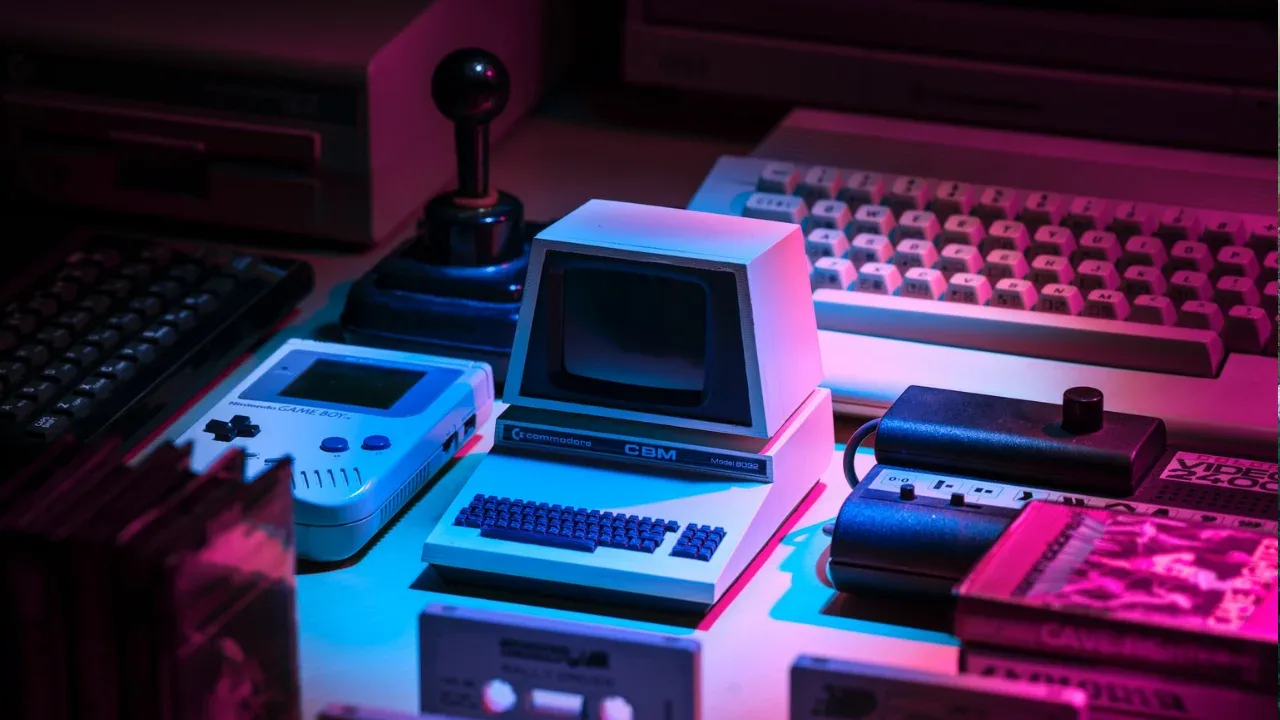
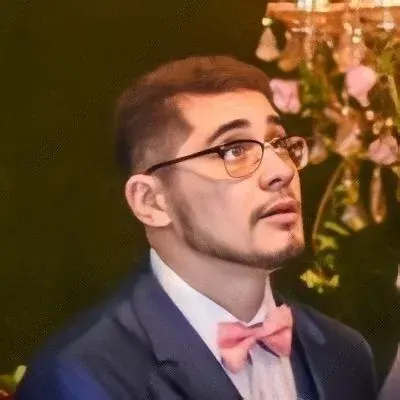
Dataclass from a Nested Dict: Converting Dicts to Dataclasses in Python 🐍
Are you struggling with converting a nested dictionary back into a dataclass in Python? You're not alone! It can be a tricky problem to solve, especially when the fields of the dataclass are themselves dataclasses. But fear not, because in this blog post, we'll explore some easy solutions to this common issue. Let's dive in! 💻🔍
The Challenge: Converting Nested Dicts to Dataclasses 🤔
Let's start by understanding the challenge at hand. Python's dataclass
module, introduced in version 3.7, provides a convenient way to define classes with automatic generation of special methods like __init__
, __repr__
, and more. One of the great features of dataclass
is the ability to convert instances of it into dictionaries using the asdict()
function.
Here's a quick example from the Python docs:
from dataclasses import dataclass, asdict
from typing import List
@dataclass
class Point:
x: int
y: int
@dataclass
class C:
mylist: List[Point]
p = Point(10, 20)
assert asdict(p) == {'x': 10, 'y': 20}
c = C([Point(0, 0), Point(10, 4)])
tmp = {'mylist': [{'x': 0, 'y': 0}, {'x': 10, 'y': 4}]}
assert asdict(c) == tmp
As you can see, the asdict()
function beautifully converts the Point
and C
instances into dictionaries. However, the reverse process of converting a nested dictionary back into a dataclass is not provided by the standard library.
Easy Solution with Pydantic 🚀
Fortunately, the Python ecosystem provides some amazing libraries that solve this problem effortlessly. One standout library is Pydantic. It not only allows you to define data validation and parsing rules, but also provides a simple way to convert dictionaries into dataclasses.
To install Pydantic, you can use pip:
$ pip install pydantic
For our example, let's convert the tmp
dictionary back into a C
dataclass using Pydantic's parse_obj()
function:
from pydantic import BaseModel, ValidationError
class Point(BaseModel):
x: int
y: int
class C(BaseModel):
mylist: List[Point]
try:
c = C.parse_obj(tmp)
print(c)
# Output: C mylist=[Point x=0 y=0, Point x=10 y=4]
except ValidationError as e:
print(e)
# Output: <validation error message>
By defining the data structure using Pydantic's BaseModel
class and calling parse_obj()
with the dictionary, we can easily convert the nested dictionary into the C
dataclass. Pydantic handles data validation during the conversion process and raises a ValidationError
if any issues arise.
Alternative Libraries for Converting Dicts to Dataclasses 📚
While Pydantic is a fantastic choice for converting dictionaries to dataclasses, there are a few other libraries worth mentioning:
Dacite: Dacite is a lightweight library that focuses on automatic dataclass instantiation from dictionaries. It provides an
from_dict()
function that can handle dictionary conversion to dataclasses with ease.Mashumaro: Mashumaro is another library that simplifies the conversion of dictionaries to dataclasses. It offers more customization options than Dacite, such as field renaming and ignoring missing fields.
It's always a good idea to explore different libraries and choose the one that best fits your needs. Each library has its own strengths and quirks, so make sure to check out their documentation and community support.
Wrap Up and Engage with the Community! 🙌
Converting a nested dictionary back into a dataclass in Python can be challenging, especially when dealing with nested dataclasses. Fortunately, libraries like Pydantic, Dacite, and Mashumaro provide easy solutions to this problem.
Now it's your turn to experiment, explore, and engage with the Python community! Have you faced challenges with converting dict to dataclass? Which libraries have you found helpful in solving this problem? Let us know in the comments section below! Share your thoughts, experiences, and recommendations. Let's learn and grow together as Pythonistas! 🐍💪
Remember to subscribe to our blog for more tech tips and tricks! Until next time, happy coding! ✨🚀
Note: The code examples provided in this blog post are based on Python 3.7 and may not work with older versions of Python. Make sure to use the appropriate versions of the mentioned libraries for compatibility.