Python 3: UnboundLocalError: local variable referenced before assignment
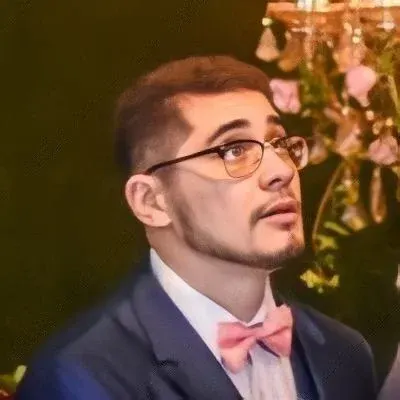
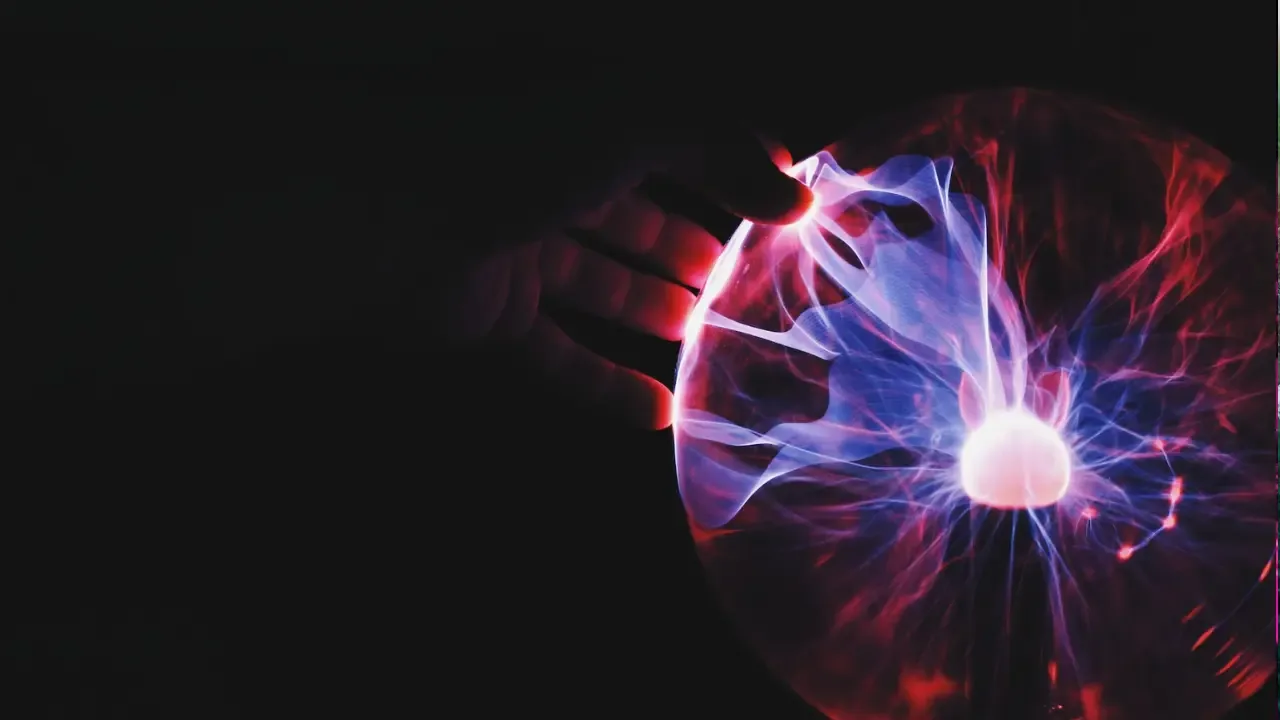
🔥 Python 3: UnboundLocalError: local variable referenced before assignment 🔥
Hey there, fellow Pythonistas! 👋 Have you ever encountered the dreaded "UnboundLocalError: local variable referenced before assignment" error while coding in Python? Fear no more, because I'm here to rescue you from the depths of debugging hell! 🚀
Understanding the Issue
Let's dive right into the heart of the problem. In the code snippet provided, we have a global variable Var1
defined outside the function function()
. Within the function, we attempt to modify Var1
using the assignment operator -=
.
However, Python thinks that Var1
is a local variable within the function since we're reassigning its value. And here lies the problem! ⚠️
The Culprit: Name Shadowing
🔍 The concept to understand here is called "name shadowing." This occurs when you have both a global and a local variable with the same name. The local variable takes precedence over the global variable within the scope of the function. When you try to modify the local variable before it has been assigned, Python throws the UnboundLocalError
.
In our code snippet, Python assumes Var1
inside the function is a local variable because we're assigning it. However, we refer to Var1
before assigning it inside the function, leading to the UnboundLocalError
alert.
Easy Solutions to Fix the Error
Thankfully, we have a handful of easy-peasy solutions to tackle this issue:
Solution 1: Use the global
Keyword
One way to solve this problem is by declaring Var1
as a global variable inside the function using the global
keyword. This explicitly tells Python that we're referring to the global variable instead of a local one.
Var1 = 1
Var2 = 0
def function():
global Var1 # Declare Var1 as a global variable
if Var2 == 0 and Var1 > 0:
print("Result 1")
elif Var2 == 1 and Var1 > 0:
print("Result 2")
elif Var1 < 1:
print("Result 3")
Var1 -= 1
function()
Solution 2: Make Use of Function Arguments
Another approach is to pass the required variables as arguments to the function instead of relying on global variables. By doing so, we avoid any ambiguity between the local and global scopes.
Var2 = 0
def function(Var1): # Pass Var1 as an argument
if Var2 == 0 and Var1 > 0:
print("Result 1")
elif Var2 == 1 and Var1 > 0:
print("Result 2")
elif Var1 < 1:
print("Result 3")
Var1 -= 1
Var1 = 1
function(Var1) # Invoke the function with Var1 as an argument
The Power Is in Your Hands 💪
Now that you know how to overcome the "UnboundLocalError: local variable referenced before assignment" error, you can level up your Python game 🐍✨. So go ahead, dive into your code, and fix those bugs with confidence!
If you found this guide helpful, don't hesitate to share it with your fellow developers who might be wrestling with the same issue. Sharing is caring, after all! 😄 And if you have any other Python-related questions or topics you'd like me to cover, drop a comment below or reach out on social media! Let's keep the conversation flowing! 💬📢
Happy coding! 🎉🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
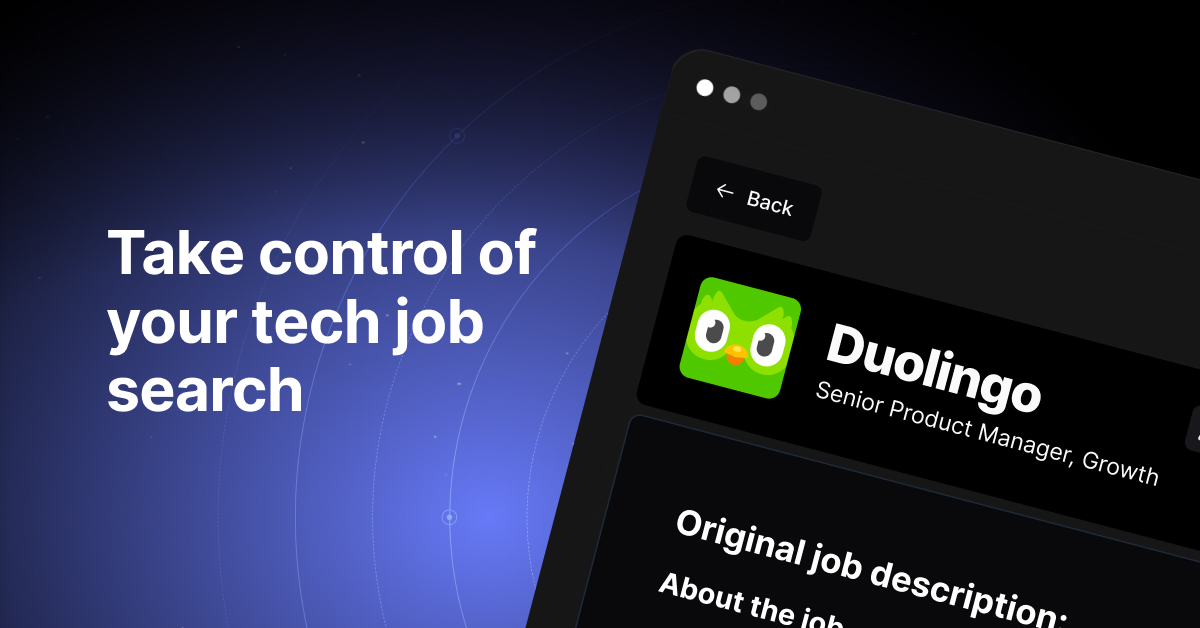