Python 3 turn range to a list
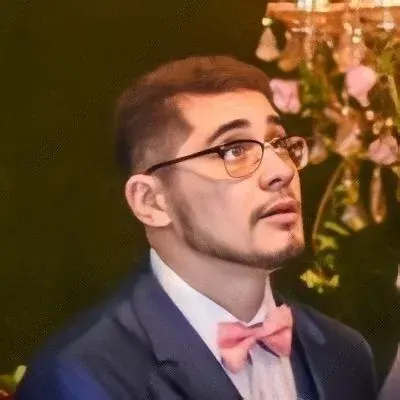
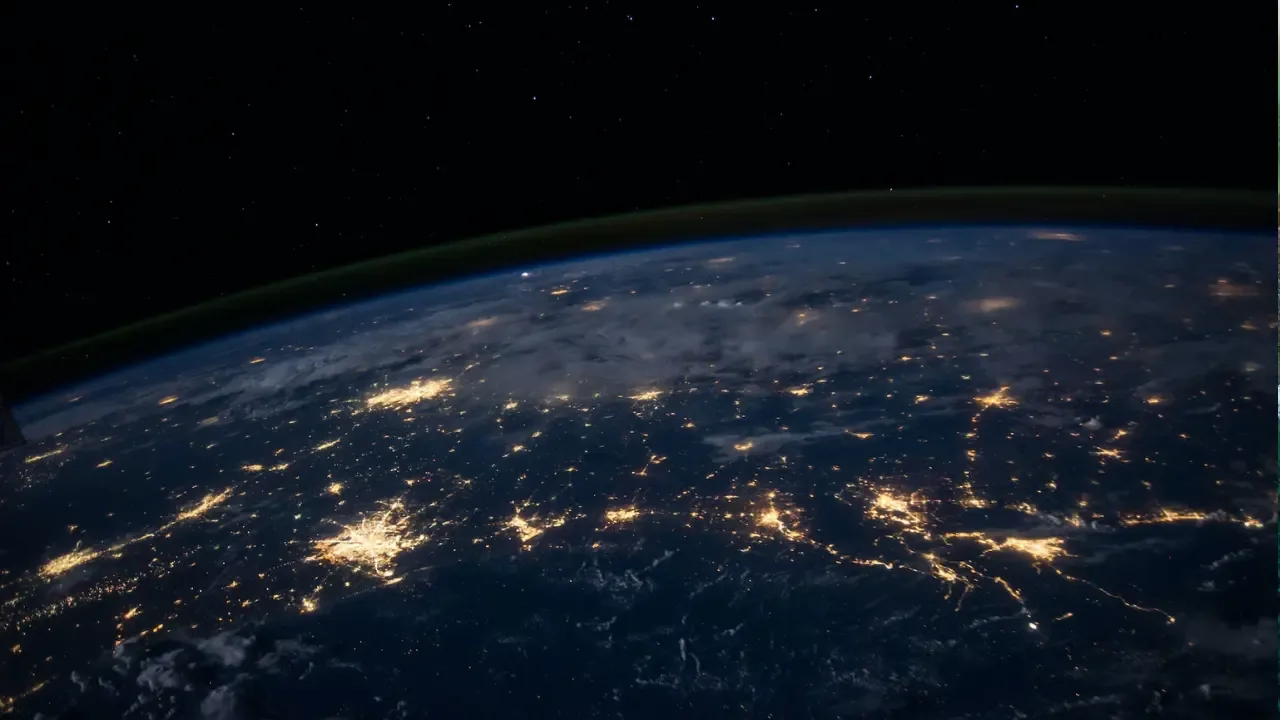
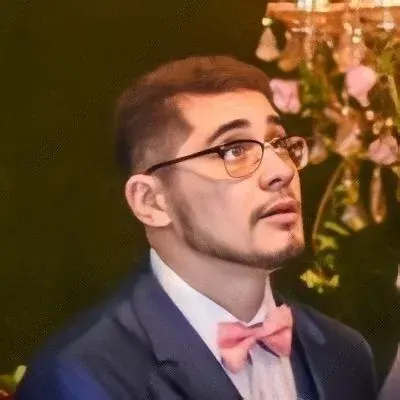
Python 3: Turning Range into a List š
⨠Hey there, Pythonistas! Are you struggling with turning a range into a list in Python 3? Don't worry, we've got you covered! In this blog post, we'll discuss the common issues you might encounter, provide easy solutions, and give you a compelling call-to-action to keep you engaged. Let's dive in! šŖ
Understanding the Problem š¤
The context around this question is that the range()
function in Python 2 used to return a list, but in Python 3, it behaves more like the xrange()
function in Python 2. This change was made to improve memory usage and performance for larger ranges. So, how can we turn a range into a list in Python 3?
Easy Solution: Using the list()
Function šÆ
To convert a range into a list in Python 3, you can simply pass the range to the list()
function. Let's see it in action:
some_list = list(range(1, 1000))
That's it! The list()
function takes any iterable and converts it into a list. This includes ranges as well. We passed our desired range as an argument to the list()
function and assigned the result to the some_list
variable. Now, some_list
contains all the numbers from 1 to 1000 in a list format.
Handling Large Ranges with Generators š
While using the list()
function is the straightforward solution, it might not be the best approach for very large ranges. Creating a list with millions of elements can consume a lot of memory. In such cases, using a generator expression along with the list()
function can be a more memory-efficient solution.
some_list = list(i for i in range(1, 1000000))
In this example, we're using a generator expression (i for i in range(1, 1000000))
instead of directly passing the range to the list()
function. The generator expression lazily generates each element of the range as required, reducing memory consumption. The list()
function then converts the generator into a list.
Dive Deeper: Understanding Generators and List Comprehensions šāāļø
Generators and list comprehensions are powerful features of Python that allow you to create sequences in a concise and memory-efficient way. If you're interested in learning more about them and when to use each, check out our detailed guide on generators and list comprehensions.
⨠Pro Tip: If you ever need to convert a range into a list backwards, simply provide a negative step value:
some_list = list(range(1000, 0, -1))
Your Turn to Engage! š¬
We hope this guide helped you understand how to turn a range into a list in Python 3. Now it's your turn to engage! Share your thoughts, questions, or additional tips in the comments section below. Are there any other Python topics you'd like us to cover? Let us know! š„
Happy coding and see you in the next blog post! āļø