Putting many python pandas dataframes to one excel worksheet
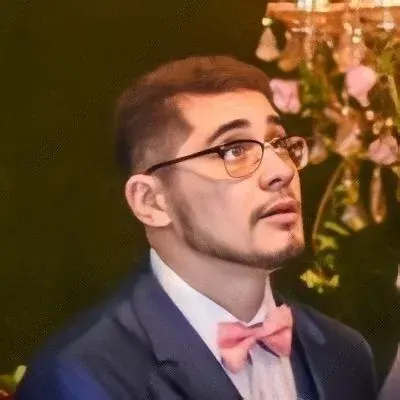
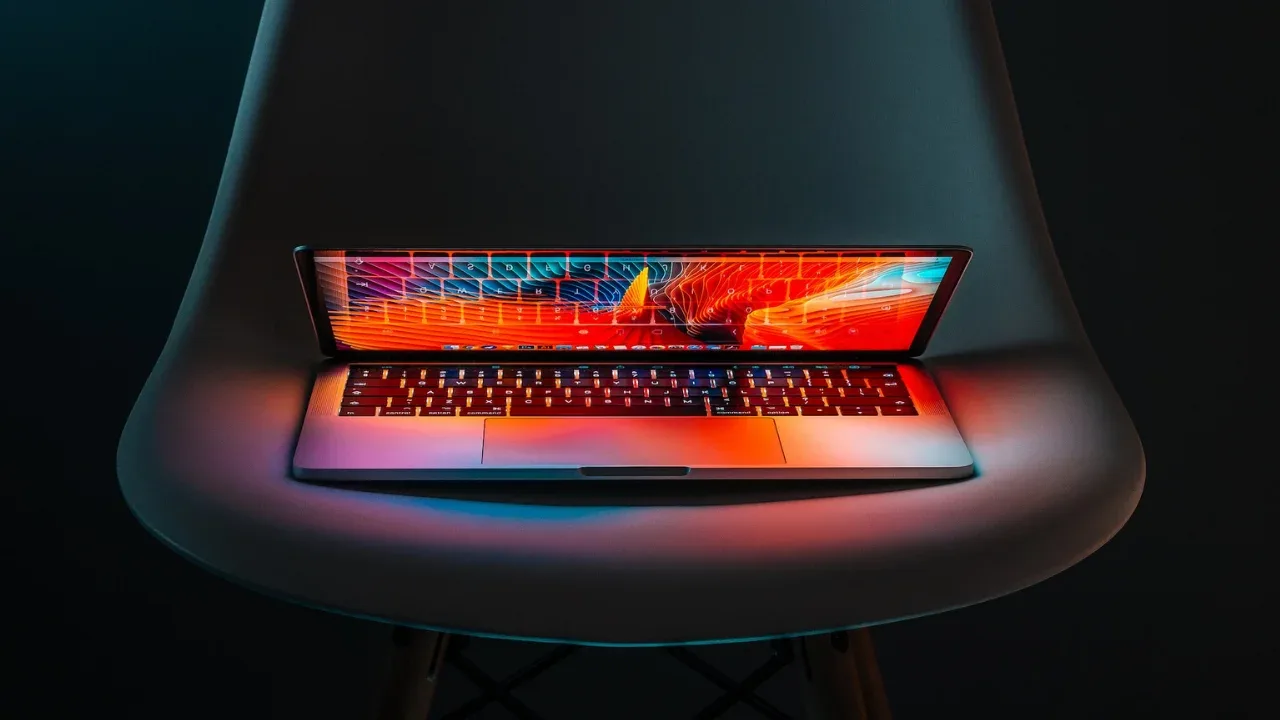
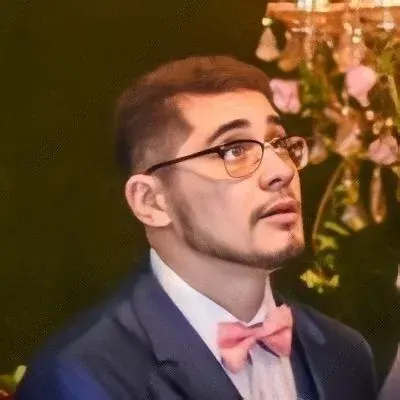
Putting many pandas dataframes to one excel worksheet: A Complete Guide
š Are you struggling to add multiple pandas dataframes into one worksheet in Excel? Don't worry, you're not alone! It can be a bit tricky to achieve this using the built-in df.to_excel
functionality. But fear not, in this blog post, we'll explore common issues, provide easy solutions, and share alternative methods to help you seamlessly integrate pandas dataframes into a single Excel worksheet. Let's dive in! š
The Common Error
When attempting to add multiple dataframes to a single worksheet using df.to_excel
, you might encounter the following error:
Sheetname 'Validation', with case ignored, is already in use.
This error occurs because by default, df.to_excel
tries to create a new worksheet with the given name Validation
every time it is called. As a result, if the worksheet already exists, it throws this error and prevents the creation of multiple dataframes within the same worksheet.
The Easy Solution
Fortunately, there is a simple solution to overcome this error and successfully add multiple dataframes to a single worksheet using df.to_excel
. The key is to create the Excel Writer object before adding the dataframes. Follow these steps:
# Step 1: Create the Excel Writer object
writer = pd.ExcelWriter('test.xlsx', engine='xlsxwriter')
# Step 2: Add the dataframes to the worksheet
df.to_excel(writer, sheet_name='Validation', startrow=0, startcol=0)
another_df.to_excel(writer, sheet_name='Validation', startrow=20, startcol=0)
# Step 3: Save and close the Excel file
writer.save()
writer.close()
By creating the Excel Writer object, df.to_excel
recognizes that the worksheet already exists and uses it for subsequent dataframe additions. This workaround ensures that no errors will be thrown, and you'll successfully have multiple dataframes in the same worksheet!
The Difference Explained
Now, let's address the difference between the initial code block you shared and the updated solution. In the first block, where you encountered the error, you created the worksheet explicitly before adding the dataframes. However, in the second block, you skipped creating the worksheet beforehand.
The critical distinction lies in the behavior of the df.to_excel
function. When you call df.to_excel
without explicitly creating the worksheet, it automatically generates the worksheet for you. In the case of the second block, the worksheet is created when you add the first dataframe. As a result, when you reach the last line of code, the "Validation" worksheet already exists, allowing subsequent additions without throwing the error.
Alternative Methods
While df.to_excel
is the built-in method for exporting dataframes to Excel, there are alternative approaches worth exploring. Two popular options are using the xlsxwriter
library and the openpyxl
library.
Option 1: Using xlsxwriter
import xlsxwriter
# Step 1: Create the Workbook object
workbook = xlsxwriter.Workbook('test.xlsx')
# Step 2: Add the worksheet and dataframes
worksheet = workbook.add_worksheet('Validation')
worksheet.write_dataframe(df, startrow=0, startcol=0)
worksheet.write_dataframe(another_df, startrow=20, startcol=0)
# Step 3: Save and close the workbook
workbook.close()
By using xlsxwriter
, you can create a Workbook object and add dataframes directly to the worksheet without the need for an Excel Writer object. This approach provides flexibility and control over the Excel file creation process.
Option 2: Using openpyxl
from openpyxl import Workbook
from openpyxl.utils.dataframe import dataframe_to_rows
# Step 1: Create the Workbook object
workbook = Workbook()
# Step 2: Add the worksheet and dataframes
worksheet = workbook.active
for row in dataframe_to_rows(df, index=False, header=True):
worksheet.append(row)
for row in dataframe_to_rows(another_df, index=False, header=True):
worksheet.append(row)
# Step 3: Save and close the workbook
workbook.save('test.xlsx')
By leveraging openpyxl
, you can create a Workbook object and manipulate worksheets directly. Through iteration over dataframe rows, you can easily add the dataframes to the worksheet.
Final Thoughts
In conclusion, adding multiple pandas dataframes to a single Excel worksheet may initially present some challenges. However, by following our easy solution and exploring alternative methods using libraries like xlsxwriter
or openpyxl
, you can achieve your desired outcome swiftly.
š So go ahead and combine your pandas dataframes in one worksheet with confidence! And if you have any further questions or alternative approaches, we'd love to hear from you in the comments below. Happy coding! šš»š”