psycopg2: insert multiple rows with one query
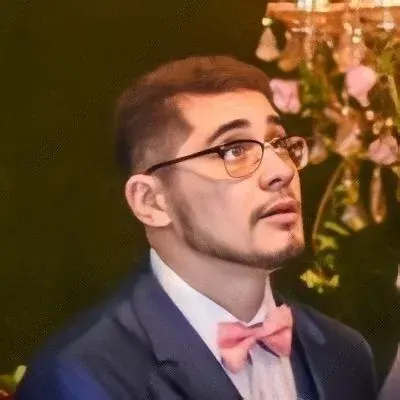
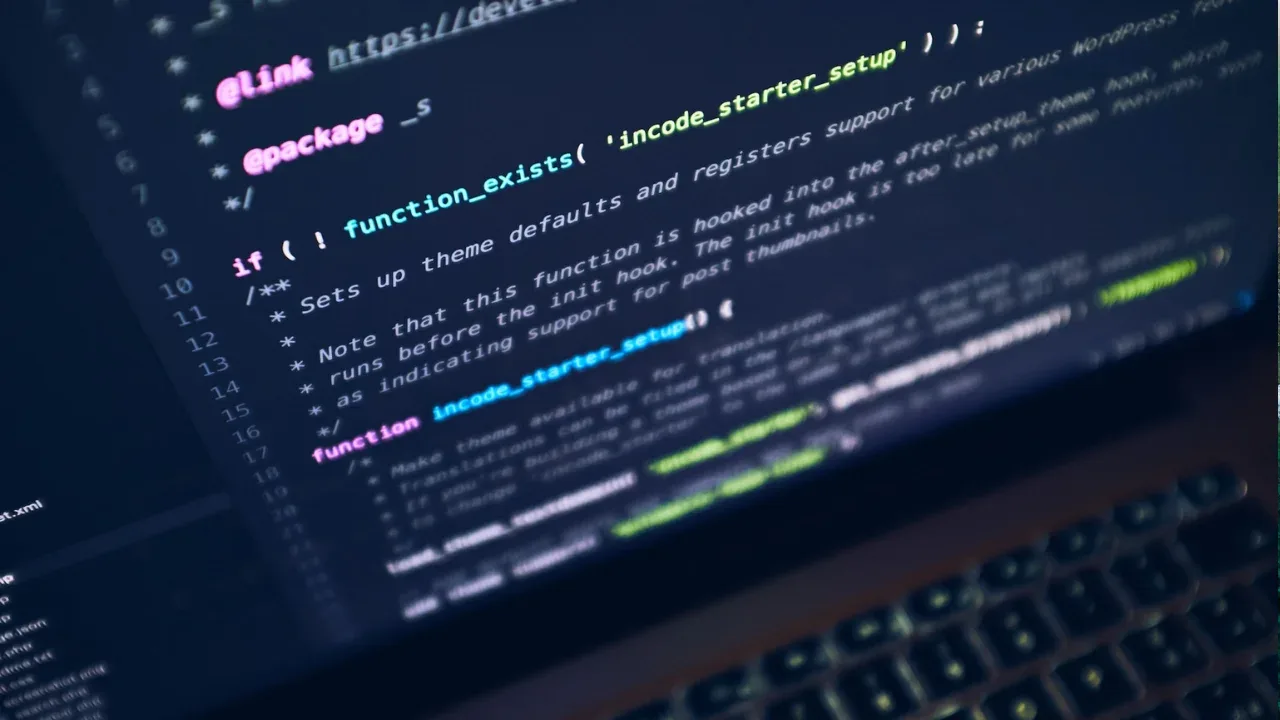
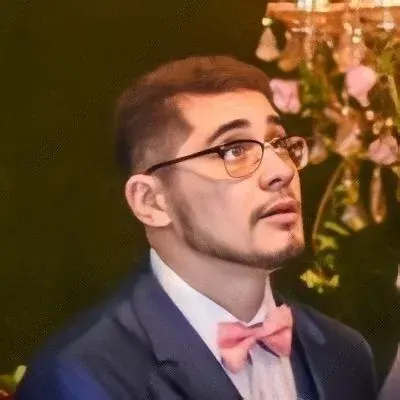
📝 Tech Blog Post: Insert Multiple Rows with One Query in psycopg2 📝
Are you tired of writing long and complicated code just to insert multiple rows with one query in psycopg2? We feel your pain! 😫
In this blog post, we'll address the common issues around this problem and provide you with easy and simplified solutions so that you can save valuable time and energy. Let's dive in! 💪
The Challenge: Inserting Multiple Rows with One Query
So, you need to execute a query that inserts multiple rows into a table, but the number of rows is not constant. You want to achieve something like this:
INSERT INTO t (a, b) VALUES (1, 2), (3, 4), (5, 6);
The code snippet you mentioned works, but you're looking for a simpler way to accomplish this task. We're here to help you out! 🙌
The Simplified Solution: Using executemany()
Good news! psycopg2 provides a handy function called executemany()
that simplifies the process of inserting multiple rows with one query. 🎉
Here's the easy-to-understand code snippet to achieve your desired result:
data = [(1, 2), (3, 4), (5, 6)]
query = "INSERT INTO t (a, b) VALUES (%s, %s)"
cursor.executemany(query, data)
Let's break it down step by step:
Create a list called
data
containing tuples, where each tuple represents the values for each row you want to insert.Define the query using placeholders
%s
for the values you want to insert. In this case,(a, b)
.Use the
executemany()
function on your cursor object and pass thequery
anddata
as arguments. This function will handle the iteration and efficiently execute the query for each tuple indata
.
That's it! You've successfully simplified your code and achieved the insertion of multiple rows with just one query. 🚀
Take it to the Next Level
Now that you've discovered this simplified method, you can optimize your code even further! Here are some additional tips to enhance your experience with psycopg2:
Error Handling: Wrap your
executemany()
call with atry-except
block to catch any potential errors that might occur during the execution process.Dynamic Data: If the number of rows in your data is variable and unpredictable, you can modify your code to dynamically generate the
data
list based on your application's requirements.
Share Your Thoughts and Experiences
We hope this blog post has helped you overcome the challenge of inserting multiple rows with one query in psycopg2. Now it's your turn! Share your thoughts, experiences, or any other tips you may have in the comments section below. We can't wait to hear from you! 👇
Remember, simplicity is key in tech, and we're here to make your life easier. Stay tuned for more useful tech guides and solutions to enhance your productivity. Happy coding! 💻✨
Please note that the code snippets provided in this blog post are simplified for demonstration purposes. Always ensure to apply best practices and security measures according to your specific use case.