Proper way to declare custom exceptions in modern Python?
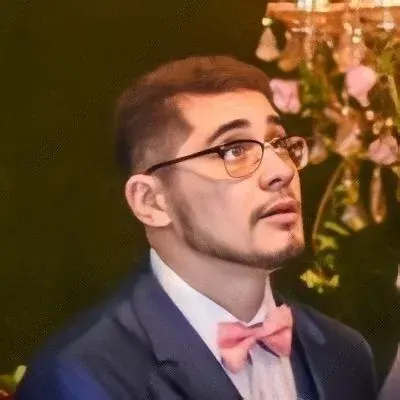
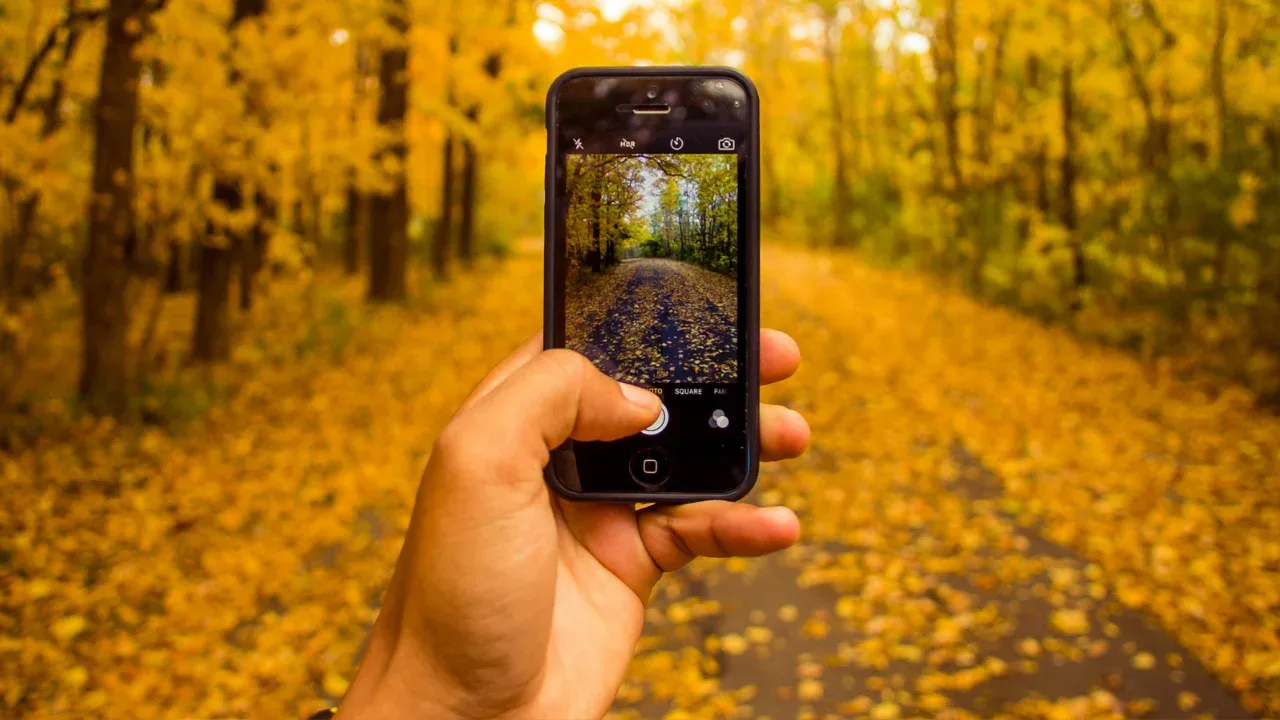
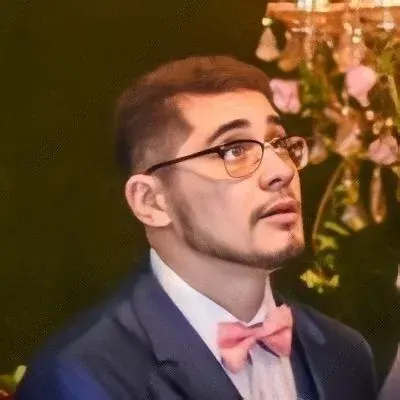
Proper way to declare custom exceptions in modern Python? 😕🐍
So you want to declare custom exception classes in Python? No worries, I got your back! In this blog post, we will explore the proper way to declare custom exceptions in modern Python, taking into account compatibility with Python 2.5, 2.6, and Python 3.*.
The Issue: Deprecation Warning 🚨
Let's start by addressing the issue you mentioned with the deprecation warning in Python 2.6.2. It seems like you encountered the deprecation of the message
attribute in BaseException
. This deprecation aims to discourage the use of message
as an attribute name.
According to PEP-352, the message
attribute had a special meaning in Python 2.5, which they are trying to deprecate. As of Python 2.6 and onwards, it is no longer advisable to use message
as an attribute name.
Custom Exception Solution 🛠️
Now, let's dive into the solution for declaring custom exception classes in a modern Pythonic way. We can achieve this by following these steps:
Inherit from the built-in
Exception
class: To create a custom exception class, start by creating a class and making it inherit from theException
class. This inheritance ensures that our custom exception is compatible with the standard exception classes.
class MyError(Exception):
pass
Define a constructor: Next, define a constructor (
__init__
) within your custom exception class to handle any extra data about the cause of the error. You can pass any relevant arguments to the constructor and handle them accordingly.
class MyError(Exception):
def __init__(self, message):
self.message = message
Override string representation methods: To provide meaningful text when the exception is printed or logged, it's a good practice to override the
__str__
(or__unicode__
in Python 2) and__repr__
methods in your custom exception class.
class MyError(Exception):
def __init__(self, message):
self.message = message
def __str__(self):
return f"MyError: {self.message}"
def __repr__(self):
return f"MyError({repr(self.message)})"
Using the custom exception: To use your custom exception, simply raise it like any other exception in your code. You can pass any relevant information to the constructor when raising the exception.
raise MyError("Something went wrong!")
Python 3 and the 'args' Parameter 🔄
You mentioned being unsure about the usage and relevance of the args
parameter in Python exceptions. In Python 3, the args
parameter is still available for backwards compatibility, but it's generally recommended to use explicit attributes in your custom exception classes instead.
Given that you defined a constructor and stored the message as an attribute (self.message
), you can include it in the exception's string representation without using args
.
Is Overriding 'init' and 'str' Necessary? 💭
You also raised a concern about the amount of typing required when overriding __init__
, __str__
, and __repr__
. While it may seem like a lot of additional code, it's considered good practice to provide meaningful representations of your custom exceptions.
By customizing __repr__
, you ensure that the error is properly represented when debugging or logging, enhancing the developer experience. Overriding __str__
allows you to display a concise error message when the exception is printed or logged, helping with troubleshooting.
Conclusion and Call-to-Action 🏁🙌
Congratulations! You now know the proper way to declare custom exceptions in modern Python. Remember to inherit from Exception
, define a constructor, and consider overriding __str__
and __repr__
for improved error handling and debugging.
So, go ahead and level up your Python exception game by creating custom exceptions like a pro! If you have any questions or other Python topics you'd like me to cover, leave a comment below or reach out on social media. Happy coding! 😄💻
👉👉👉 Have you encountered any unique scenarios when using custom exceptions in Python? Share your experiences and let's discuss in the comments! 👈👈👈