Progress indicator during pandas operations
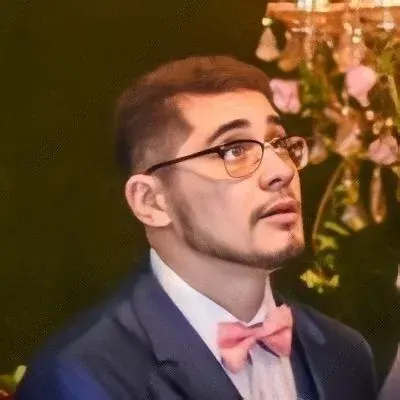
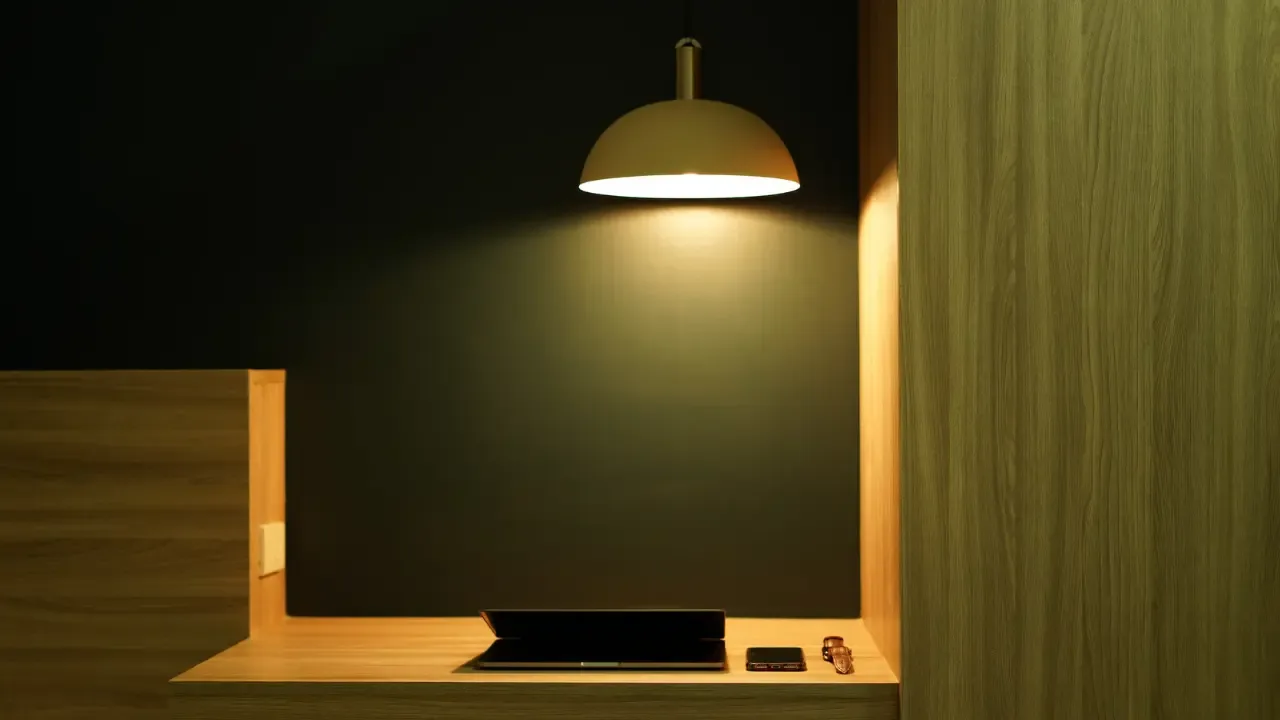
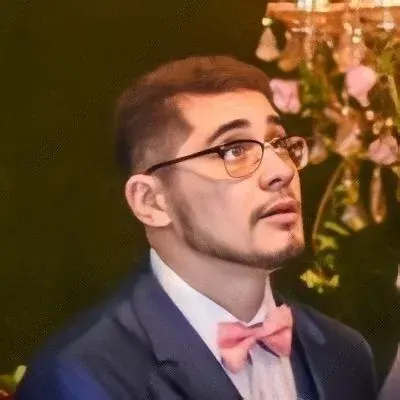
📊 Track Progress During Pandas Operations: A Complete Guide
Are you tired of waiting for long-running pandas operations to complete? Do you wish you had a way to track the progress of your data frame operations? Well, you're in luck! In this guide, we'll explore common issues surrounding progress indicators during pandas operations and provide you with easy solutions to keep you informed every step of the way. Let's dive in! 💻
🤔 The Problem: Lack of Progress Indicators in Pandas
If you regularly work with large data frames containing millions of rows, you know how time-consuming certain pandas operations can be. It's frustrating to have no idea how much longer you'll have to wait before a particular operation completes. That's where progress indicators come into play.
The question we're addressing today is: "Does a text-based progress indicator for pandas split-apply-combine operations exist?" The user wants to know if there's a way to track the progress of operations like groupby
and apply
in real-time, especially when working with complex functions like feature_rollup
.
The user has already tried using canonical loop progress indicators for Python, but they don't interact with pandas in a meaningful way. They are looking for a solution that seamlessly integrates with pandas to provide an informative progress output.
⚡️ The Solution: tqdm to the Rescue
Fortunately, there is a fantastic Python library called tqdm
that solves our progress tracking problem. tqdm
stands for "taqaddum," which means "progress" in Arabic. It allows us to create progress bars and provides us with useful progress information right in our iPython notebook. Let's see how we can use tqdm
to track the progress of pandas operations.
First, make sure you have tqdm
installed. If not, you can install it by running the following command:
!pip install tqdm
Once you have tqdm
installed, you can start using it in your code. Here's an example that demonstrates how to use tqdm
with the groupby
and apply
operations:
from tqdm import tqdm
import pandas as pd
# Create a progress bar using tqdm
progress_bar = tqdm(total=len(df_users))
# Define the function to be applied
def feature_rollup(row):
# Your function implementation here
# Apply the function with tqdm
df_users.groupby(['userID', 'requestDate']).apply(lambda x: feature_rollup(x, progress_bar.update(1)))
# Close the progress bar
progress_bar.close()
In this example, we import tqdm
and create a progress bar using tqdm(total=len(df_users))
. The total
parameter is set to the length of your data frame, which gives tqdm
the information it needs to track progress accurately.
Inside the apply
operation, we pass a lambda function that calls feature_rollup
on each group. Additionally, we use progress_bar.update(1)
within the lambda function to increment the progress bar by one for each row processed.
Finally, we close the progress bar using progress_bar.close()
. Voila! You now have a text-based progress indicator for your pandas split-apply-combine operations.
💡 The Call-to-Action: Contribute and Share Your Progress
Now that you have a solution to track progress during pandas operations, why not share your newfound knowledge with others? Tell us about your experiences using tqdm
or any other progress tracking methods in the pandas community. Together, we can improve the library and make data analysis even more enjoyable for everyone. Comment below or tweet us using #PandasProgress to join the conversation.
Remember, tracking progress is not just about reducing waiting time; it's also about gaining insights into the performance of your code and improving your workflows. Happy coding! 🚀