Programmatically saving image to Django ImageField
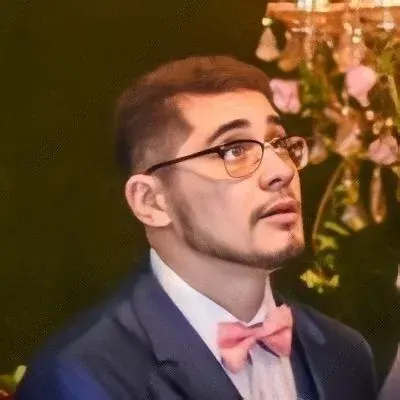
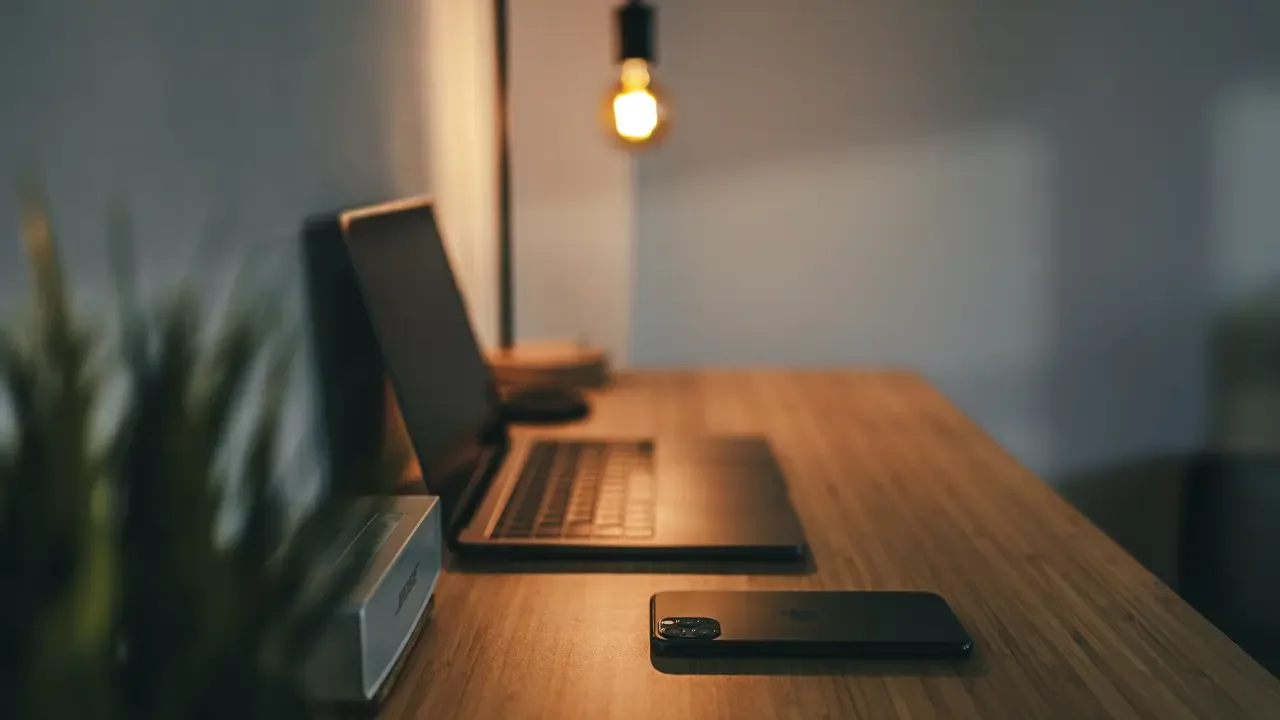
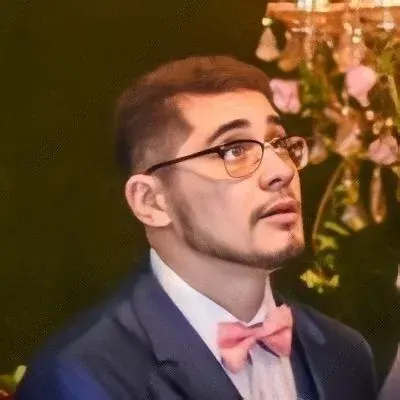
📸 Programmatically Saving Images to Django ImageField
Capture, store, and display your images in Django with ease!
So, you've been trying everything under the sun to programmatically save an image to a Django ImageField, but nothing seems to be working? Don't worry, we've got you covered. In this guide, we'll address common issues, provide easy solutions, and empower you to conquer this problem once and for all. Let's dive in! 😎
The Setup
Before we jump into the solution, let's understand the context. You have a Django model with an ImageField, and you need to associate an existing image file path with that field. Seems simple enough, right? Let's see the problem you're facing.
The Problem
You've written the code in multiple ways, but it always results in Django creating a second file, renaming it with an underscore at the end, and leaving it empty. 🤔 Here's an illustration to make it crystal clear:
## Image generation code runs....
/Upload
generated_image.jpg 4kb
## Attempt to set the ImageField path...
/Upload
generated_image.jpg 4kb
generated_image_.jpg 0kb
ImageField.Path = /Upload/generated_image_.jpg
The Solution
Fear not! We have the solution that will make your life much easier. Here's how you can programmatically associate the existing image file path with the ImageField:
from django.core.files import File
import os
# Assuming 'model' is your Django model instance
image_path = "path/to/your/image.jpg" # Replace with your image file path
# Open the image file in binary mode
with open(image_path, 'rb') as image_file:
# Create a Django File object with the image file
django_file = File(image_file)
# Generate a unique file name for the ImageField
filename = os.path.basename(image_path) # Extract the file name from the path
model.ImageField.save(filename, django_file)
That's it! By opening the image file in binary mode, creating a Django File object with it, and calling the save()
method on your ImageField, you'll be able to associate the existing image file path seamlessly. 🙌
Compatibility Note
You mentioned that you encountered this issue when running under Apache on Windows Server. Surprisingly, the same code worked flawlessly when running under the 'runserver' on XP. This behavior suggests a potential compatibility issue specific to your server environment. Though further investigation is needed, at least you have a working solution on the XP environment for now.
Wrapping Up
Congratulations on finding the perfect solution to programmatically save images to your Django ImageField! We hope this guide has resolved your issue and made your Django development journey smoother.
If you have any questions or want to share your experience, leave a comment below. Happy coding! 💻🚀