Print without b" prefix for bytes in Python 3
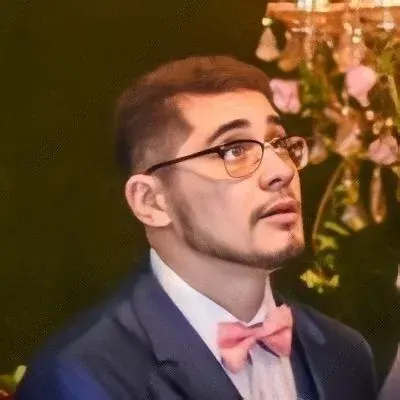
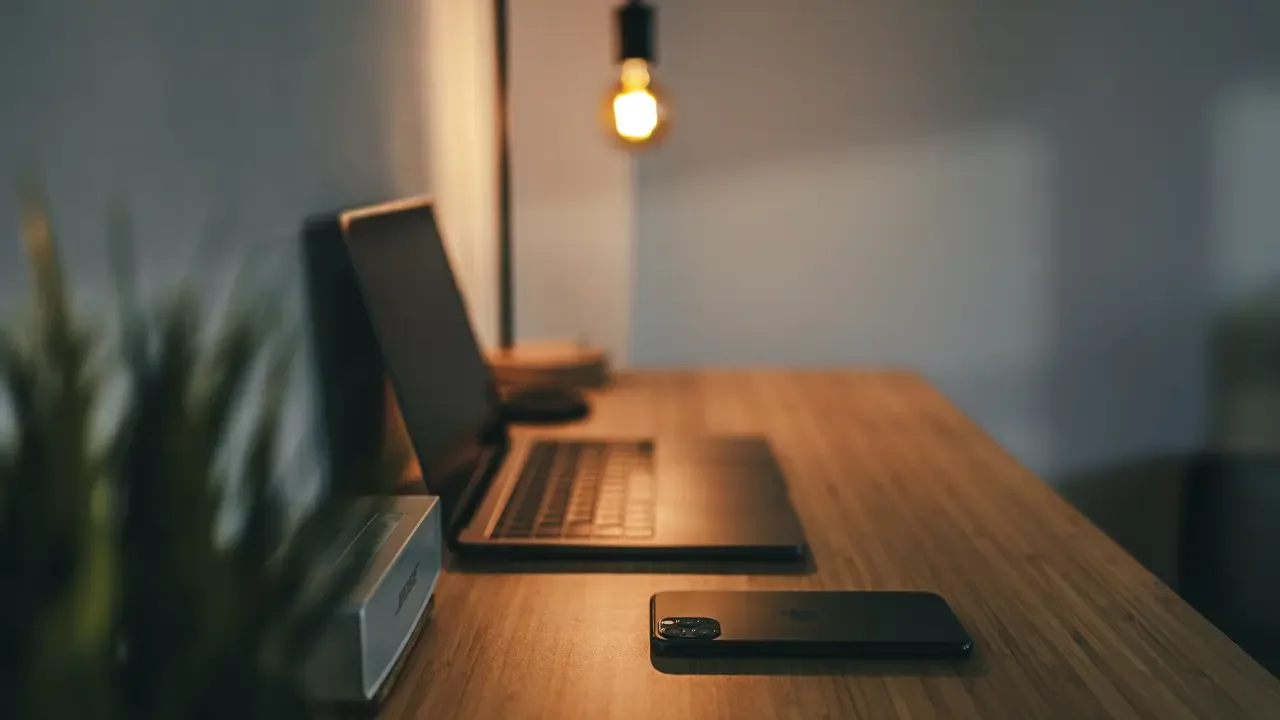
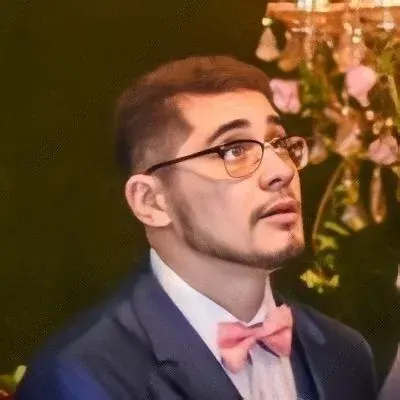
🖨 Print without b' prefix for bytes in Python 3
So you're working with Python 3 and bytes
strings and you want to print them without that annoying b'
prefix. You're not alone! This is a common issue that Python developers face. But fear not, for I'm here to guide you through this problem and provide easy solutions.
🤔 The Problem
By default, when you print a bytes
string in Python 3, it includes the b'
prefix to indicate that it's a bytes object. This prefix can be unwanted and make your output look a bit messy. Let's take a look at an example:
>>> print(b'hello')
b'hello'
As you can see, the b'
prefix is added to the output. But what if you just want to see the string itself, without the prefix?
💡 The Solution
Luckily, Python provides several ways to print bytes
strings without the b'
prefix. Let's explore a few options:
1. Decode the bytes to a string
One simple solution is to decode the bytes using the .decode()
method and print the resulting string. Here's how you can do it:
>>> print(b'hello'.decode())
hello
By decoding the bytes, we convert them into a Unicode string which can be printed without the prefix.
2. Use the str()
function
Another way to remove the b'
prefix is by converting the bytes
object to a string using the str()
function:
>>> print(str(b'hello', 'utf-8'))
hello
In this example, we pass the bytes
object and the encoding (utf-8
) to the str()
function, which returns a string representation without the prefix.
3. Format the bytes string
If you prefer to keep the original bytes
object intact and just modify the display, you can use string formatting to remove the prefix. Here's an example:
>>> print(f'{b"hello"[2:-1]}')
hello
In this case, we're using an f-string to format the bytes
object. By slicing the bytes string from index 2 to -1, we exclude the prefix from the output.
👉 Your Turn!
Now that you know a few ways to print bytes
strings without the b'
prefix in Python 3, give it a try in your own code! Experiment with different solutions and see which one works best for you.
If you have any other Python-related questions or need further assistance, don't hesitate to let me know in the comments below. Happy coding! 😄🐍
References: