Print string to text file
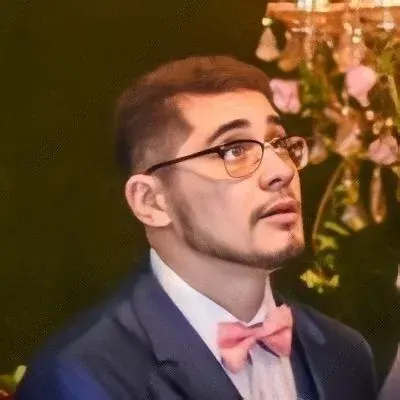
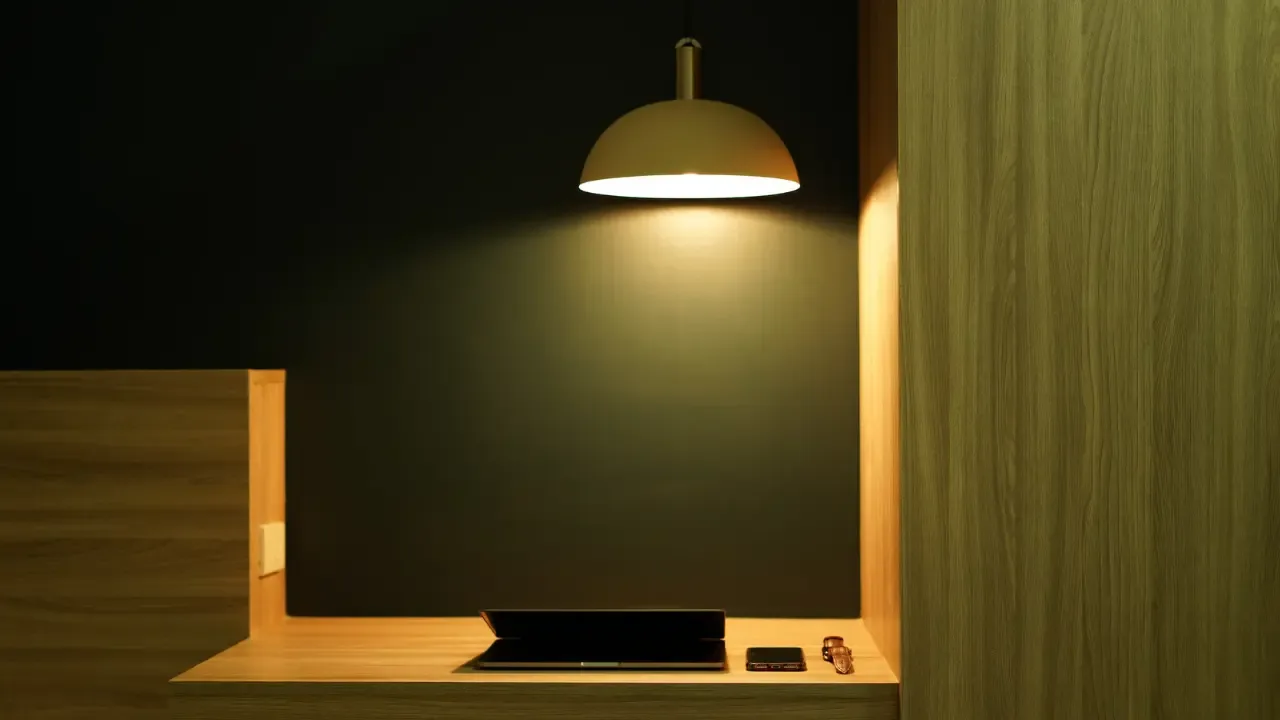
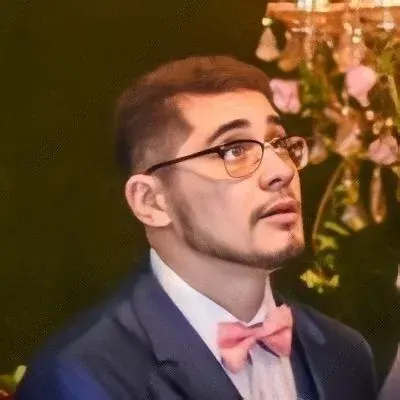
📝 How to Print a String to a Text File using Python
Are you trying to print a string to a text file using Python and unsure of how to do it? You're in luck! In this article, we'll discuss the common issues faced when performing this task and provide easy solutions to help you get the job done. So let's dive right in! 💪
📂 Common Issue: Substituting a String Variable into a Text Document
The code snippet you provided is a great starting point. However, it seems like you're trying to replace the value of the string variable TotalAmount
in the text document. Currently, your code is simply writing the text "Purchase Amount: TotalAmount"
to the file.
To substitute the value of the TotalAmount
variable into the text document, you can make use of string formatting in Python.
💡 Solution: Using String Formatting to Substitute Variable Value
To achieve your goal, you can modify your code snippet as follows:
text_file = open("Output.txt", "w")
TotalAmount = 100 # Assume a value for TotalAmount
text_file.write("Purchase Amount: {}".format(TotalAmount))
text_file.close()
In this updated code snippet, we're using curly braces {}
as a placeholder for the value of TotalAmount
. By utilizing the format()
method of the string, we can replace the placeholder with the actual value of the variable.
✨ Example: Printing Calculated Total Amount
To illustrate the complete process, let's consider an example where the variable TotalAmount
is calculated using some logic or fetched from another part of the code. We'll assume the value of TotalAmount
to be 100 for simplicity.
text_file = open("Output.txt", "w")
TotalAmount = 100
text_file.write("Purchase Amount: {}".format(TotalAmount))
text_file.close()
When you run the code, it will write the following line to the Output.txt
file:
Purchase Amount: 100
By using string formatting, we successfully substitute the value of TotalAmount
into the text document.
📣 Call-to-Action: Share Your Experience!
Now that you know how to print a string to a text file using Python, why not give it a try yourself? Modify the example code provided and experiment with different strings and variable values. Share your experience and any interesting findings in the comments below. We'd love to hear from you! 🎉
So go ahead, start coding, and don't forget to hit that share button to spread the knowledge among your fellow Python enthusiasts. Happy coding! 😄🐍