Pretty printing XML in Python
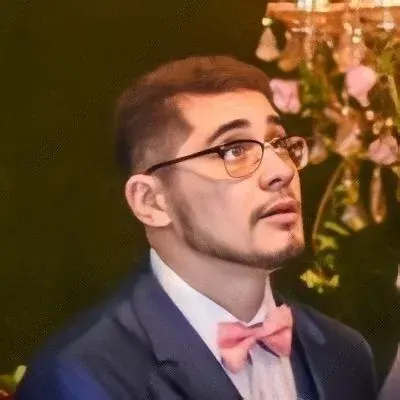
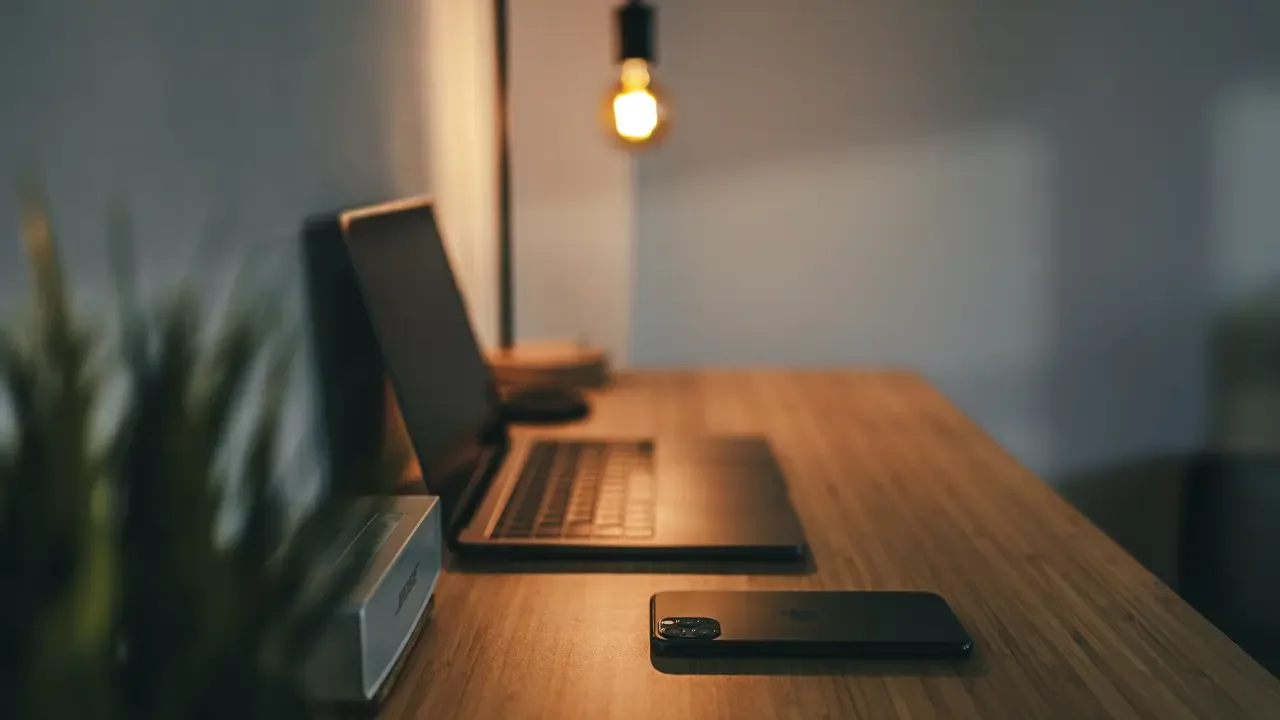
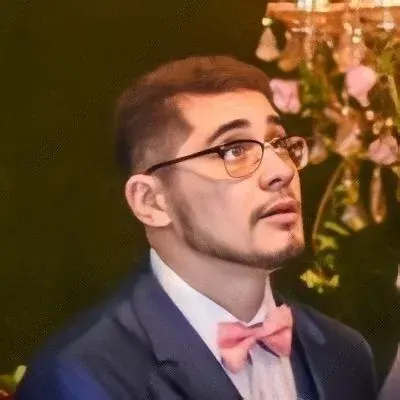
💻🔥 Pretty Printing XML in Python: The Ultimate Guide
Are you tired of staring at messy and unreadable XML code in Python? Whether you're a beginner or an experienced coder, formatting XML properly is essential for readability and maintainability. In this guide, we will explore common issues faced while pretty printing XML in Python and provide you with easy solutions to make your code shine ✨. Let's dive in!
Understanding the Problem
When dealing with XML data, it's common to encounter long strings or files filled with compacted XML code. It becomes challenging to navigate and understand the structure of the data. That's where pretty printing comes to the rescue! Pretty printing XML involves adding indentation, line breaks, and spacing to make your XML code visually appealing and easier to comprehend.
The Easy Solutions
Python provides several methods and libraries to pretty print XML effortlessly. Let's explore some of these solutions:
1. Using the xml.dom.minidom
library
Python's built-in xml.dom.minidom
library allows us to parse XML and manipulate its structure. Follow these simple steps to pretty print XML using minidom
:
import xml.dom.minidom
def pretty_print_xml(xml_string):
dom = xml.dom.minidom.parseString(xml_string)
pretty_xml_string = dom.toprettyxml(indent=' ')
return pretty_xml_string
xml_string = "<root><element>Content</element></root>"
pretty_xml = pretty_print_xml(xml_string)
print(pretty_xml)
This will output the indented and structured XML:
<root>
<element>Content</element>
</root>
2. Using the xml.etree.ElementTree
module
Python's xml.etree.ElementTree
module provides another handy way to prettify XML. Here's an example:
import xml.etree.ElementTree as ET
def pretty_print_xml(xml_string):
root = ET.fromstring(xml_string)
pretty_xml_string = ET.tostring(root, encoding='utf-8', method='xml')
return pretty_xml_string
xml_string = "<root><element>Content</element></root>"
pretty_xml = pretty_print_xml(xml_string)
print(pretty_xml.decode('utf-8'))
You will get the formatted XML as output:
<root>
<element>Content</element>
</root>
Take it to the Next Level
Now that you know how to pretty print XML, why not explore further? Here are some ideas to enhance your XML processing skills:
Strip unnecessary whitespace from your XML code using
.strip()
or regular expressions.Read XML from files and write the pretty printed version back to a file.
Combine XML manipulation with other Python libraries like
requests
orbeautifulsoup
.
Join the Discussion
Formatting XML is a common challenge faced by programmers. Share your experiences and any additional tips or tricks you have for pretty printing XML in Python in the comments below. Let's learn from each other and make our code more readable!
Happy coding! 😄🐍
We hope this guide was helpful in making your XML code beautiful and easier to work with. If you enjoyed this article, don't forget to share it with your fellow developers. Feel free to reach out and let us know what other topics you'd like us to cover. 👍