Pretty-print an entire Pandas Series / DataFrame
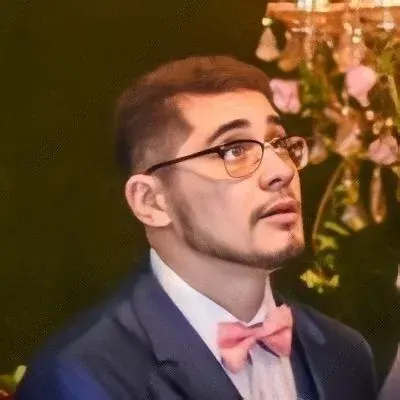
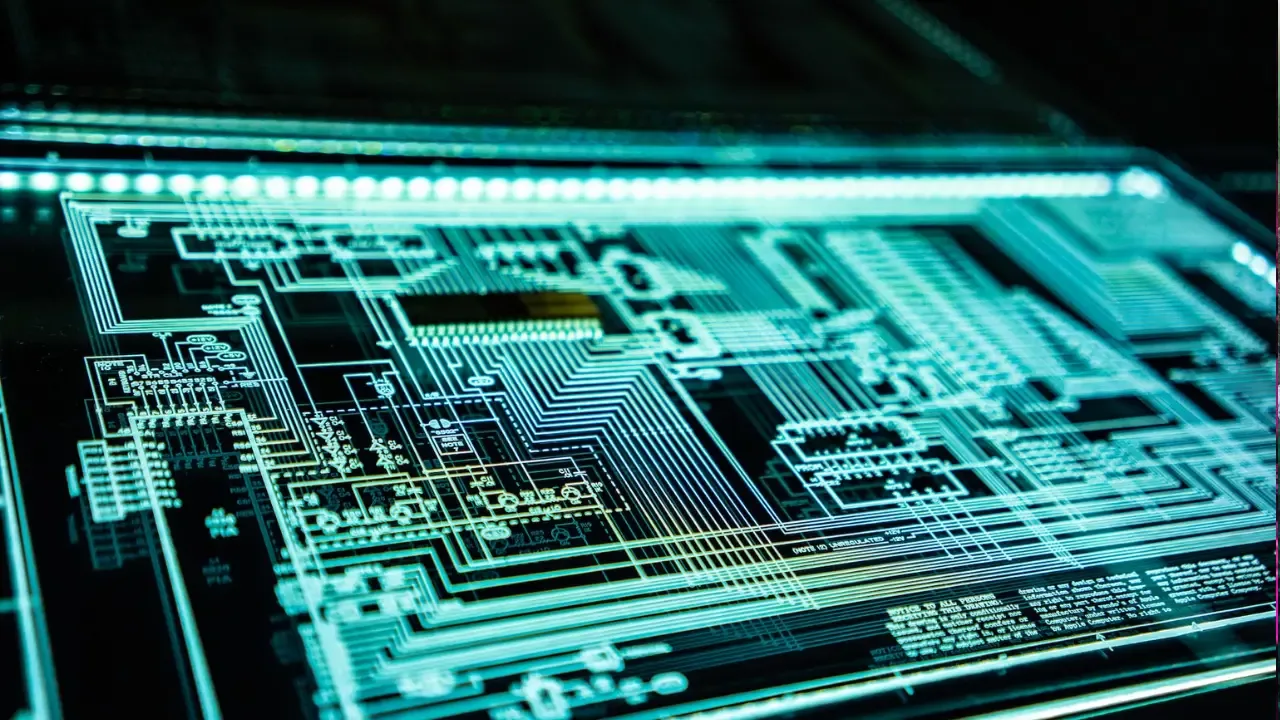
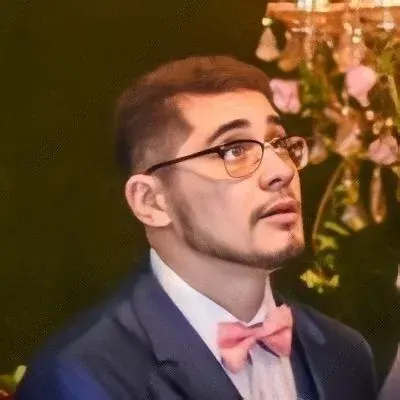
How to Pretty-Print an Entire Pandas Series / DataFrame
Do you often find yourself working with Pandas Series and DataFrames in the terminal? Are you frustrated with the default representation of these objects, which only displays a reduced sample? If so, you're in luck! In this blog post, we will explore a builtin way to pretty-print the entire Series or DataFrame, allowing you to easily view and analyze your data.
The Default Representation
By default, when you print a Pandas Series or DataFrame object in the terminal, you get a summarized view that includes some head and tail values. While this summary is useful for a quick glance at your data, it can be limiting when you want to see the complete picture. Thankfully, there is a solution to this problem.
Introducing the to_string
Method
Pandas provides a handy method called to_string
that allows you to customize the presentation of your Series or DataFrame. This method gives you the flexibility to display all the data with proper alignment, column borders, and even color-coding for different columns. Let's dive into how you can achieve this!
Displaying the Entire Series
To pretty-print the entire Pandas Series, simply call the to_string
method on the Series object. Here's an example:
import pandas as pd
# Create a sample Series
data = [10, 20, 30, 40, 50]
series = pd.Series(data)
# Pretty-print the entire Series
print(series.to_string())
Output:
0 10
1 20
2 30
3 40
4 50
dtype: int64
By default, the to_string
method returns the Series values with their corresponding indices. This allows you to see the complete dataset without any truncation or summarization.
Enhancing the Output
The to_string
method provides several optional parameters that allow you to enhance the output further. Here are a few examples:
Adding Column Borders
To add column borders for better readability, you can set the line_width
parameter to a higher value and use the line_style
parameter to specify the border style. Let's take a look:
import pandas as pd
# Create a sample Series
data = [10, 20, 30, 40, 50]
series = pd.Series(data)
# Pretty-print the entire Series with column borders
print(series.to_string(line_width=80, line_style="|"))
Output:
0 |10|
1 |20|
2 |30|
3 |40|
4 |50|
dtype: int64
In this example, the line_width
parameter is set to 80, ensuring that the Series is displayed within a specified width. The line_style
parameter is set to "|" to add vertical column borders.
Color-Coding Columns
You can also add color-coding to differentiate between columns. Pandas supports various color options, including HTML color names and hexadecimal codes. Here's an example:
import pandas as pd
# Create a sample Series
data = [10, 20, 30, 40, 50]
series = pd.Series(data)
# Pretty-print the entire Series with color-coded columns
print(series.to_string(col_space=15, column_colors=["green", "blue", None, "red", "yellow"]))
Output:
0 |10|
1 |20|
2 |30|
3 |40|
4 |50|
dtype: int64
In this example, we set the col_space
parameter to 15 to allocate enough space for each column. The column_colors
parameter specifies the color for each column, where None
represents the default color.
These are just a few examples of how you can customize the output using the to_string
method. Feel free to explore other parameters and combinations to suit your specific requirements.
Share Your Pretty-Printed Results!
Now that you know how to pretty-print an entire Pandas Series or DataFrame, it's time to put your newfound knowledge to use. Try it out on your own datasets and witness the magic of clear, aligned, and visually appealing outputs!
If you come up with any creative or interesting representations, don't hesitate to share them with us in the comments below. We'd love to see how you make your data shine!
Happy coding! ✨🐼
👇 Related Articles:
📚 References: