Pinging servers in Python
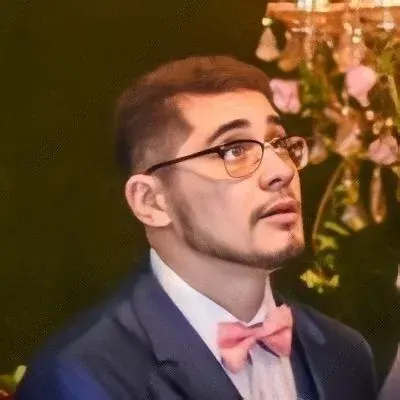
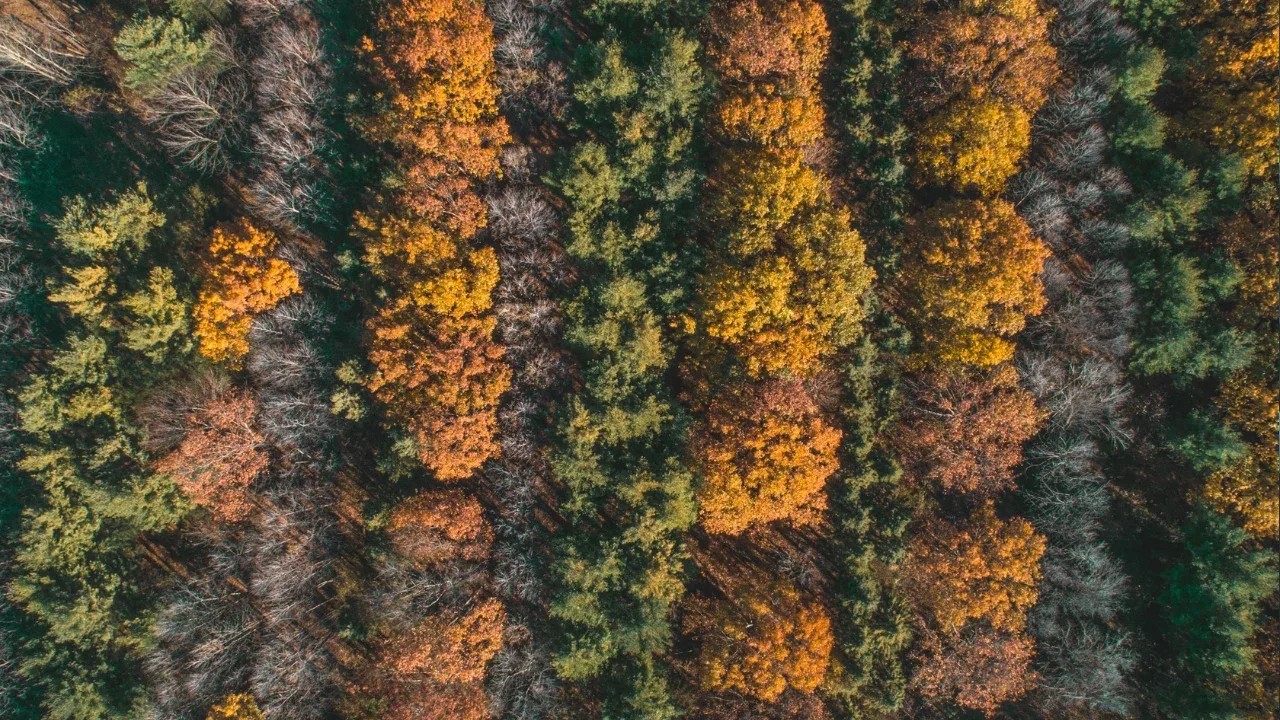
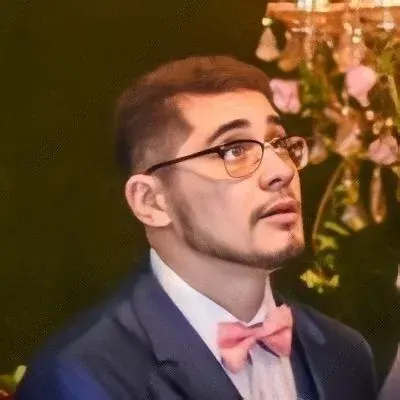
Pinging Servers in Python: A Beginner's Guide to Checking Network Connectivity 👨💻
Are you tired of manually checking if your servers are up and running? 🤔 Well, worry no more! In this blog post, we'll dive into the world of pinging servers in Python 🐍 and explore how you can effortlessly check network connectivity with just a few lines of code! 💥
The Challenge: Pinging a Server and Checking for a Response 📡
Imagine this scenario: you have a bunch of servers that are crucial for your application's performance. You need a way to programmatically check if these servers are reachable so that you can take appropriate actions if they go down. 💡
Luckily, Python provides built-in libraries that allow you to interact with network protocols and perform various networking tasks, including pinging servers. 😎
The Solution: Ping It! 🔧
Before we dive into the code, let's take a moment to understand what a ping actually is. 🤓
In simple terms, a ping is a small network packet sent from one computer to another (in our case, from your local machine to a server) and then back to your local machine. The time it takes for this round-trip communication to happen is known as the "ping time" or "latency." If the server responds within a certain time threshold, we can consider it "alive." If not, we can assume it's "down" or unreachable. 💔
Python provides a package called ping3
that simplifies the process of pinging servers and checking for a response. To install ping3
, you can use pip:
pip install ping3
Once installed, you can use the following code snippet to ping a server and determine its reachability:
import ping3
def ping(server):
response = ping3.ping(server)
return response is not None
server = "www.example.com"
if ping(server):
print(f"The server at {server} is up and running. ✅")
else:
print(f"Oh no! The server at {server} is unreachable. ❌")
In the code above, we import the ping3
package and define a ping
function that takes the server's address as input. The function uses the ping3.ping()
method to send a ping request to the server and returns True
if a response is received within the timeout threshold (default is 1 second), or False
otherwise.
By running this code, you can easily determine if a server is up or down. 🚦
Dealing with Common Issues 💣
💡 Issue #1: Firewall Blocking ICMP Echo Requests
In some cases, you might notice that your pings aren't being received, even though the server is up and running perfectly fine. The most common reason for this is a firewall blocking ICMP Echo Requests.
To overcome this issue, you can specify the timeout value when calling ping3.ping()
to increase the waiting time for a response. For example:
response = ping3.ping(server, timeout=5)
By increasing the timeout value (in seconds), you allow more time for the ping request to bypass the firewall and receive a response.
💡 Issue #2: Non-Existent Server Address
Another potential issue you might encounter is specifying an invalid server address. This can happen if you mistype the address or the server is temporarily unreachable.
To handle this, you can catch the socket.gaierror
exception that is thrown when an address-related error occurs:
import socket
def ping(server):
try:
response = ping3.ping(server)
except socket.gaierror:
return False
return response is not None
In the code above, we catch the socket.gaierror
exception and return False
to indicate that the server could not be resolved.
⚡️ Take It Further: Automate Server Monitoring!
Now that you know how to ping servers in Python, why stop there? You can take your server monitoring to the next level by creating a script that periodically pings your servers and sends notifications whenever a server goes down. 👀
You can use libraries like smtplib
to send emails or popular messaging platforms like Slack or Telegram to notify yourself or your team. Combine it with a scheduler like cron
(on UNIX-based systems) or Task Scheduler
(on Windows) to automate the script's execution.
Let your imagination run wild and tailor the monitoring solution to fit your specific needs! 🌟
So, what are you waiting for? Start pinging servers in Python today and level up your server monitoring game! 🚀
Has pinging servers in Python made your life easier? Share your thoughts, experiences, and creative solutions in the comments below! 💬👇 And don't forget to hit the share button to spread the love and help fellow developers! ❤️