Parsing XML with namespace in Python via "ElementTree"
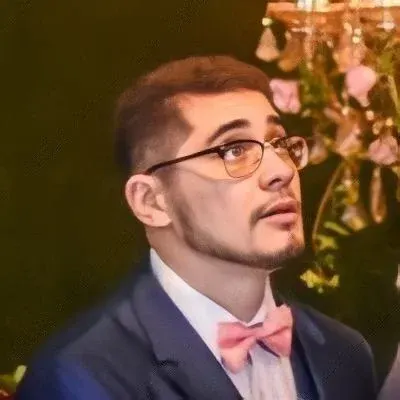
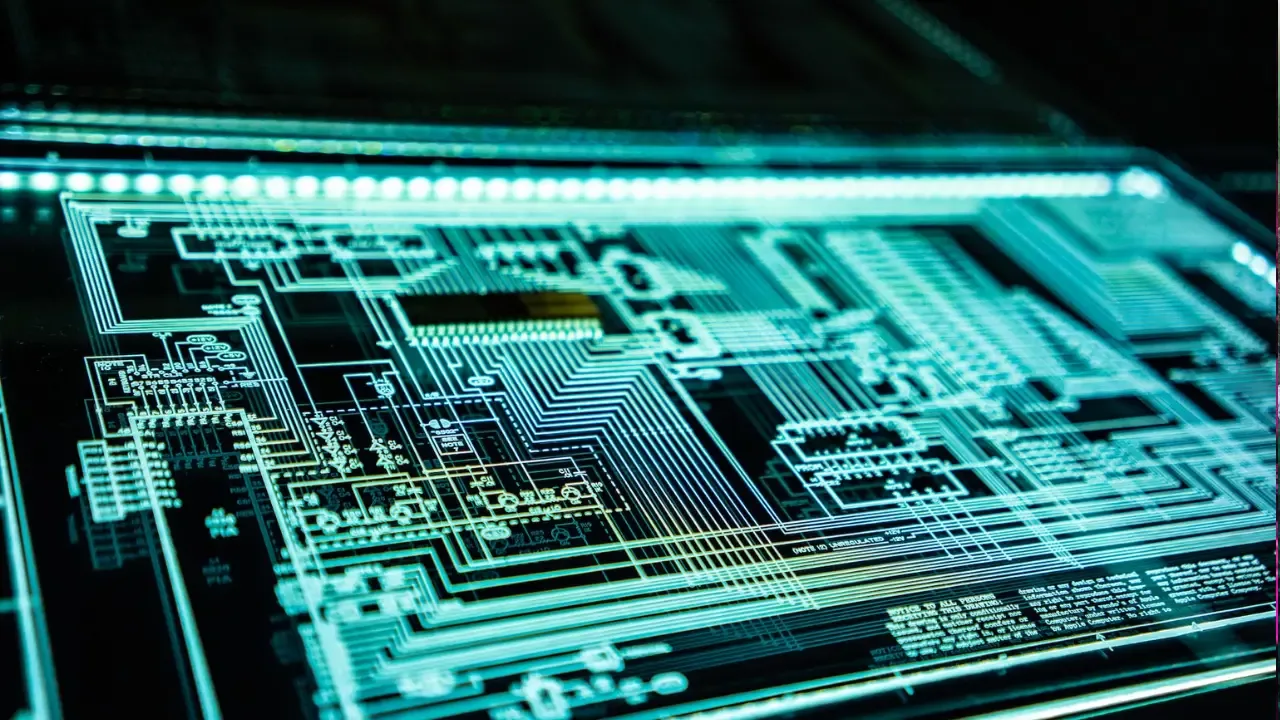
Parsing XML with Namespace in Python via 'ElementTree'
Have you ever encountered the prefix 'xyz' not found in prefix map
error while trying to parse XML with namespaces using Python's ElementTree
library? 🤔 Don't worry, you're not alone! Many developers struggle with this issue when dealing with complex XML files. But fear not, in this blog post, we'll guide you through the process of parsing XML with namespaces in Python, specifically using ElementTree
. 🎉
Understanding the problem
Let's start by understanding the problem you encountered. The XML you provided has multiple nested namespaces, including the owl
namespace. When you try to find the owl:Class
tags using the root.findall('owl:Class')
statement, you end up with the SyntaxError: prefix 'owl' not found in prefix map
error. 😢
This error occurs because ElementTree
doesn't automatically handle namespaces. You need to explicitly define and map the namespaces to make it work.
Solution
To parse XML with namespaces using ElementTree
, there are a few steps you need to follow. Let's break it down:
Step 1: Define the namespace map
First, you should define the namespace map for all the namespaces used in the XML. In this case, we have the owl
namespace. You can create a dictionary with the namespace prefixes as keys and their corresponding URIs as values.
namespaces = {
"rdf": "http://www.w3.org/1999/02/22-rdf-syntax-ns#",
"owl": "http://www.w3.org/2002/07/owl#",
"xsd": "http://www.w3.org/2001/XMLSchema#",
"rdfs": "http://www.w3.org/2000/01/rdf-schema#",
"": "http://dbpedia.org/ontology/"
}
Notice that we also include an empty namespace ""
because the default namespace doesn't have a prefix.
Step 2: Parse the XML with the namespace map
Next, when parsing the XML, you need to pass the namespaces
dictionary as the namespaces
argument to the ElementTree.parse()
function. This will associate the namespaces with their corresponding prefixes.
import xml.etree.ElementTree as ET
tree = ET.parse("filename", ET.XMLParser(encoding="utf-8"))
root = tree.getroot()
By providing the namespaces
dictionary, you're telling ElementTree
how to interpret the XML namespaces.
Step 3: Find the desired elements
Now, to find the owl:Class
tags, you can use the modified namespace-aware XPath expression. In this case, the XPath expression would be 'owl:Class'
.
classes = root.findall('.//owl:Class', namespaces)
By passing the namespaces
dictionary as the second argument, you're telling ElementTree
how to interpret the namespaces when matching the XPath expression.
Step 4: Extract the desired values
To extract the values of the rdfs:label
elements within the found owl:Class
tags, you can iterate over the classes
list and use the findtext()
method with the modified XPath expression 'rdfs:label'
.
for class_elem in classes:
label = class_elem.findtext('rdfs:label', namespaces=namespaces)
print(label)
The findtext()
method will search for the first matching element and return its text content.
Take action and parse XML with ease! ✨
Now that you know how to parse XML with namespaces using ElementTree
, you can confidently handle complex XML files in your Python projects. 💪
Don't let those namespaces scare you! With the right approach, you can conquer any XML parsing task. 🚀
So go ahead, give it a try, and let us know in the comments how your XML parsing journey is going. Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
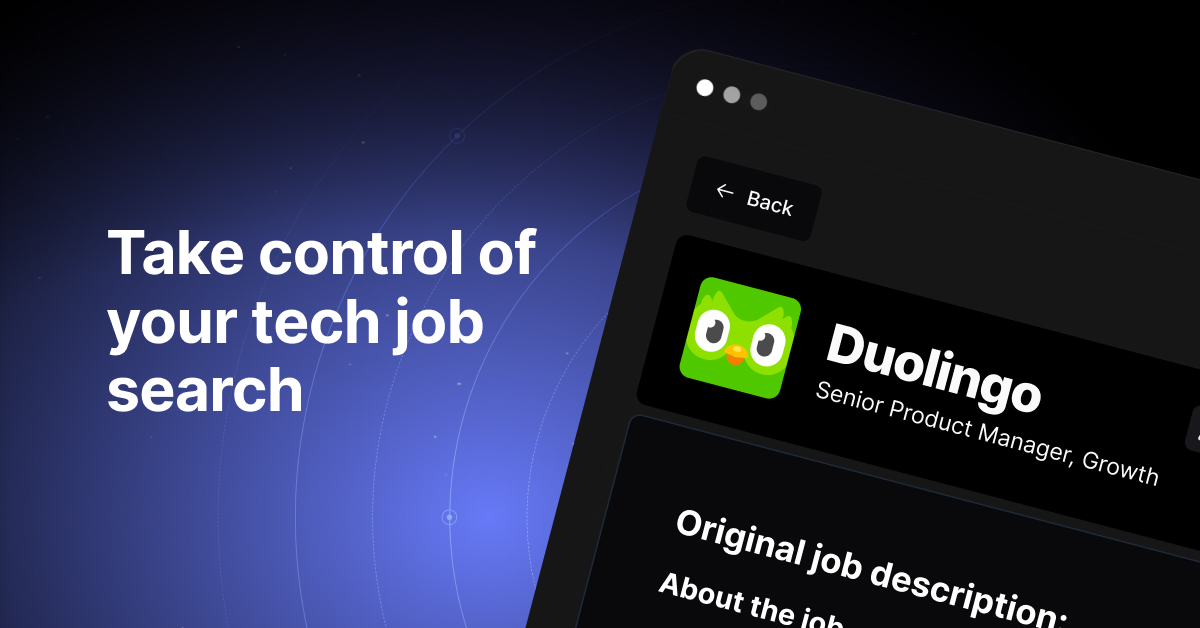