Pandas read_csv: low_memory and dtype options
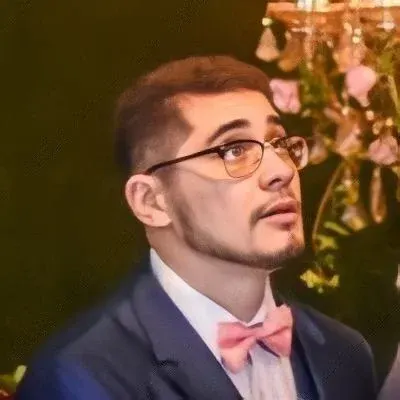
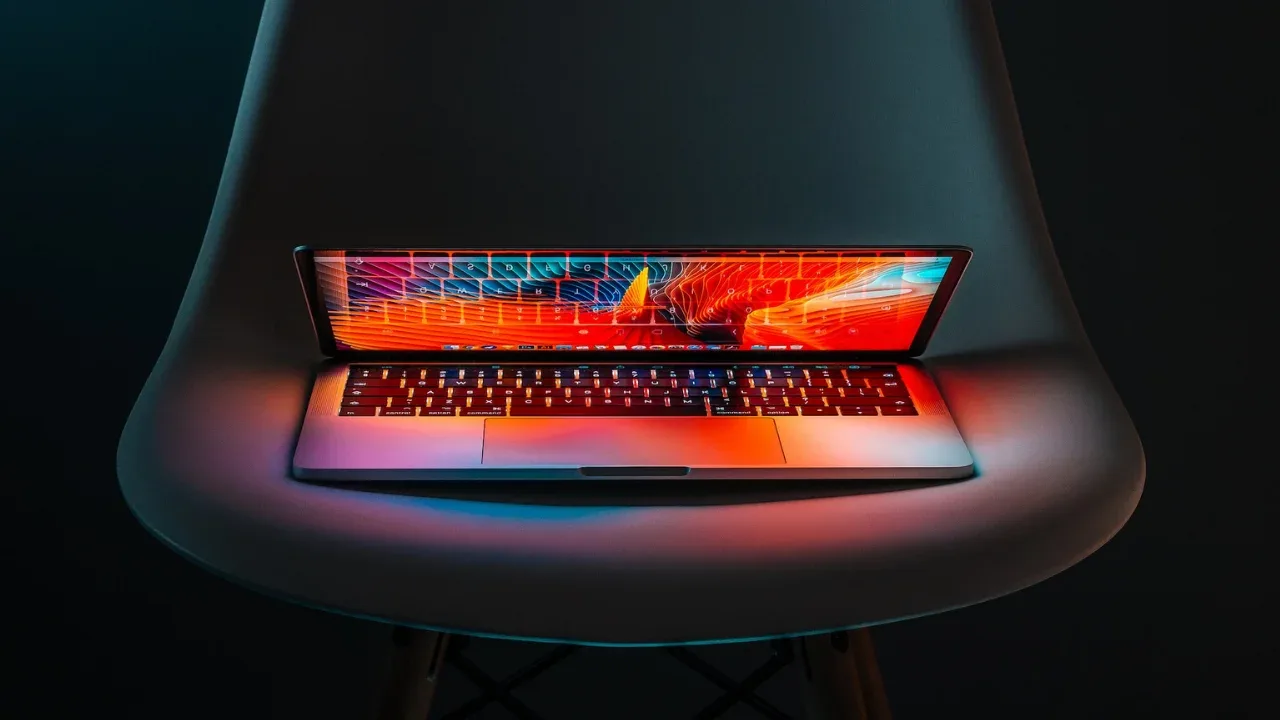
The Ultimate Guide to Pandas read_csv: low_memory and dtype options πΌπ»
If you have ever encountered the DtypeWarning
when using the pd.read_csv()
function in Pandas, you're not alone. This warning appears when some columns in your CSV file have mixed types, and it suggests specifying the dtype
option on import or setting low_memory=False
. But what does all of this mean? Let's dive in and demystify these options! π‘
Understanding the problem π΅οΈββοΈ
When you import a CSV file using pd.read_csv()
, Pandas tries to automatically infer the data types of each column. By default, it conservatively reads a small sample of the data to determine the data type for each column, which is referred to as "low memory" mode.
However, when some columns in your CSV file contain mixed types (e.g., both integers and strings), Pandas might not accurately infer the data type, leading to the DtypeWarning
. The warning serves as a heads-up that there might be unexpected results or slower performance due to the ambiguity in data types.
The dtype
option πͺ
The dtype
option allows you to explicitly specify the data type for each column when reading a CSV file with pd.read_csv()
. By setting the dtype
parameter to a dictionary mapping column names to data types, you can provide explicit instructions to Pandas on how to interpret the data.
Here's an example of how to use the dtype
option:
import pandas as pd
dtype_options = {'column1': int, 'column2': str, 'column3': float}
df = pd.read_csv('somefile.csv', dtype=dtype_options)
In this example, we specified that 'column1'
should be interpreted as an integer, 'column2'
as a string, and 'column3'
as a float. By explicitly setting the data types, you can avoid the DtypeWarning
and ensure the data is read correctly.
The low_memory
option ποΈββοΈ
Now, back to the low_memory
option. When low_memory=True
(which is the default), Pandas only reads a small sample of the data to determine the data types, resulting in a faster import process. This option is suitable for most cases when your data types are consistent within each column.
However, if you have mixed data types in your columns, setting low_memory=False
can help Pandas accurately infer their types by scanning the entire file before importing the data. Keep in mind that setting low_memory=False
could increase memory usage and slow down the import process, so use it judiciously.
To import the CSV file while setting low_memory=False
, use the following code:
import pandas as pd
df = pd.read_csv('somefile.csv', low_memory=False)
The best approach π
To ensure a smooth data import process and avoid the DtypeWarning
, consider the following steps:
Examine your CSV file and identify columns with mixed types.
Decide whether you want to use the
dtype
option or setlow_memory=False
.If the data types are consistent within each column, use the default
low_memory=True
for faster performance.If you have mixed data types, use the
dtype
option to explicitly set the data types.If you encounter memory issues or exceptionally mixed data types, set
low_memory=False
.
Your turn to take action! β¨
Now that you're armed with knowledge about the low_memory
and dtype
options in pd.read_csv()
, it's time to put it into practice! Next time you encounter the DtypeWarning
or face an import issue with mixed data types, remember this guide and take the appropriate action.
Share your experience with handling mixed data types in the comments below, and let's dive deeper into the world of Pandas together! πΌπ
References π
Happy coding! π»π‘
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
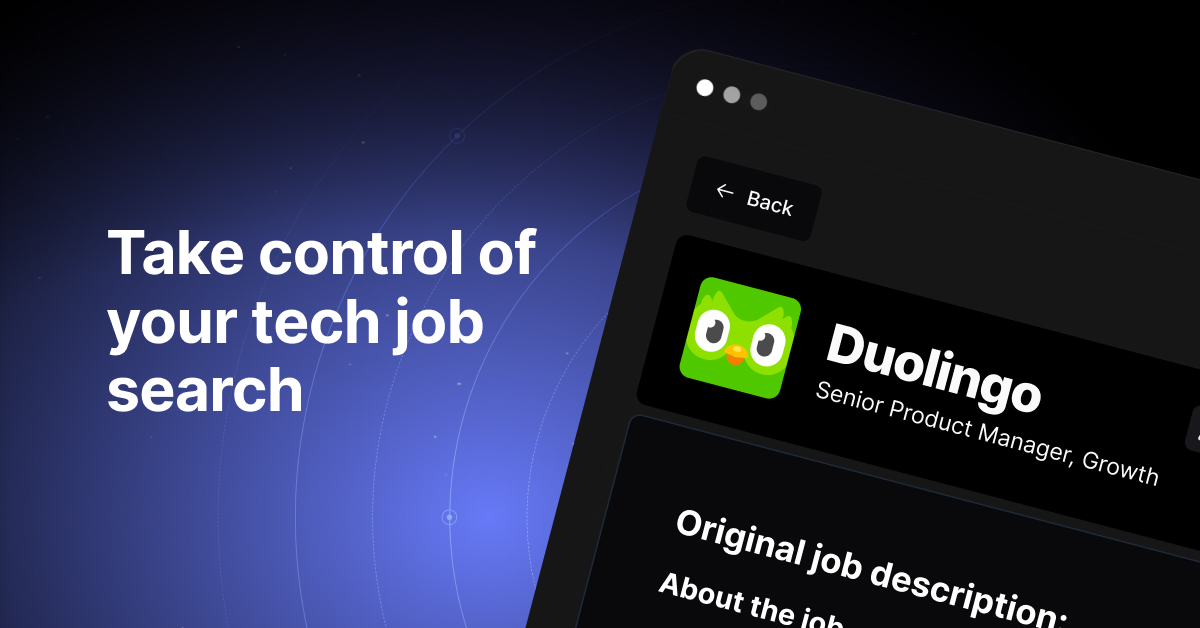