pandas how to check dtype for all columns in a dataframe?
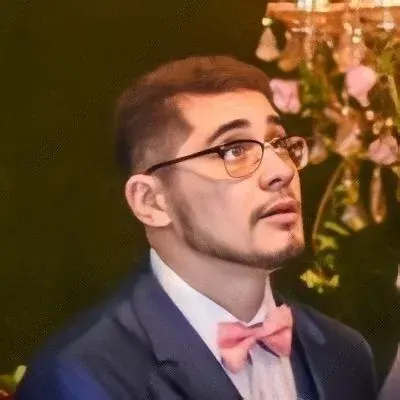
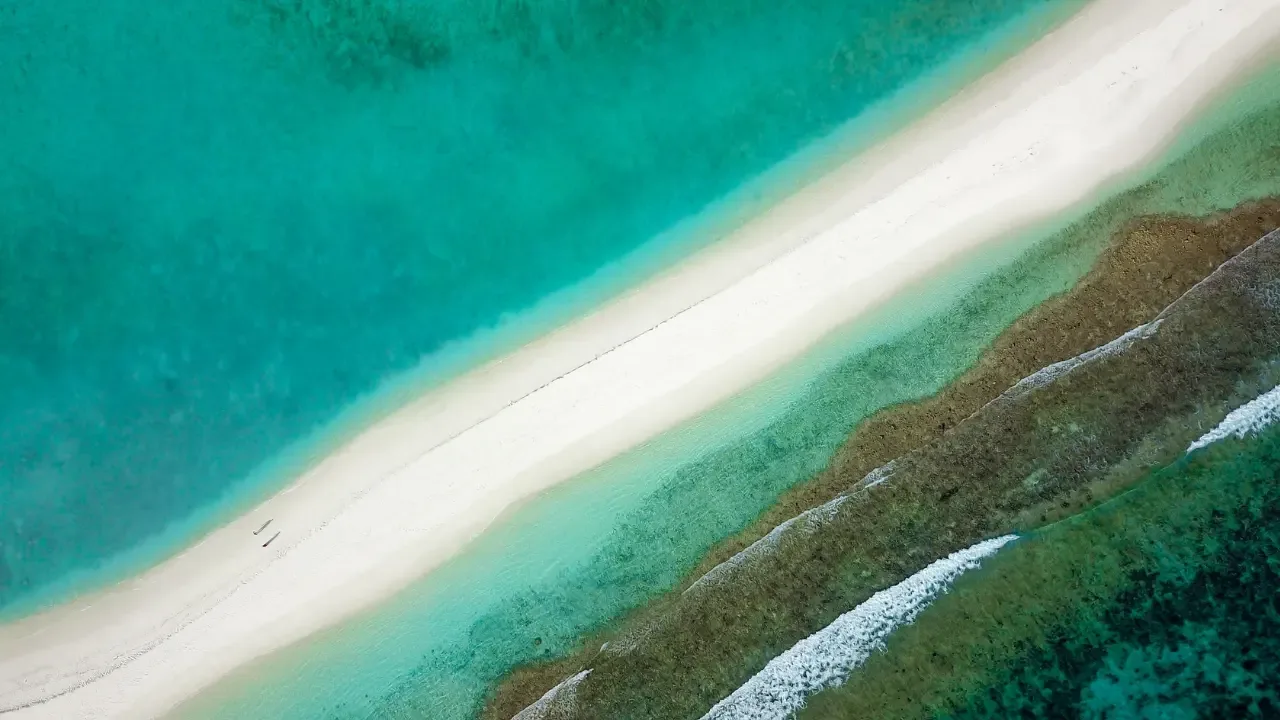
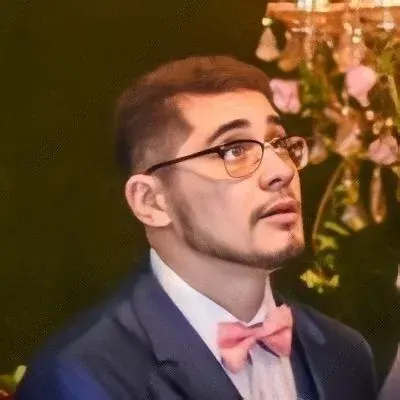
⚡️Quick Guide: How to Check the Data Types of All Columns in a Pandas DataFrame? ⚡️
Are you currently working with a Pandas DataFrame and wondering how to check the data types of all columns at once? 🧐 Look no further! In this blog post, we'll address this common question and provide you with easy solutions. Let's dive right in! 💪
The Problem:
It seems that the dtype
attribute only works for pandas.DataFrame.Series
, leaving us wondering if there's a function that can display the data types of all columns in one go. 🤔
The Solution:
Fortunately, Pandas offers a method called dtypes
that allows you to check the data types of all columns in a DataFrame! 🎉 Let's learn how to use it.
Option 1: Using the 'dtypes' Method
To use the dtypes
method, simply call it on your DataFrame. Here's an example:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['John', 'Jane', 'Mike'],
'Age': [25, 30, 35],
'Salary': [5000, 7000, 9000]}
df = pd.DataFrame(data)
# Check the data types of all columns
column_dtypes = df.dtypes
print(column_dtypes)
Running this code will output the following:
Name object
Age int64
Salary int64
dtype: object
Voilà! You now have a neat summary of the data types for each column in your DataFrame. 🎊 Notice that the dtype: object
indicates that the column contains non-numeric data, while int64
represents integer data types.
Option 2: Displaying the Result in a Table
If you prefer a more visual representation, you can convert the column_dtypes
object into a DataFrame and display it as a table. Check out the example below:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['John', 'Jane', 'Mike'],
'Age': [25, 30, 35],
'Salary': [5000, 7000, 9000]}
df = pd.DataFrame(data)
# Check the data types of all columns
column_dtypes = df.dtypes
# Create a DataFrame from the 'column_dtypes' object
dtypes_df = pd.DataFrame(column_dtypes, columns=['Data Type'])
print(dtypes_df)
Running this code will output the following:
Data Type
Name object
Age int64
Salary int64
Now you have a beautiful table that showcases the data types of each column! 📊
Conclusion:
Checking the data types of all columns in a Pandas DataFrame is as easy as pie! Today, we learned how to use the dtypes
method to achieve this with just a few lines of code. 🥧
Next time you find yourself in need of quickly checking the data types of all columns, don't forget to give this method a try! It will save you time and give you a better understanding of your data. ✨
Remember, knowledge is power, and now you have the power to conquer data type analysis in Pandas! 💪
If you found this blog post helpful, share it with your fellow data enthusiasts and help them level-up their Pandas skills! 🚀
👉 What are some other common challenges you face while working with Pandas DataFrames? Share your thoughts in the comments below! Let's learn and grow together! 🌟