pandas groupby, then sort within groups
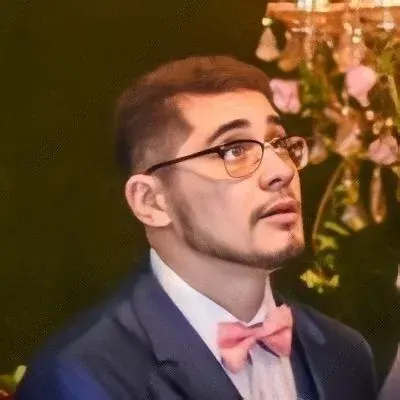
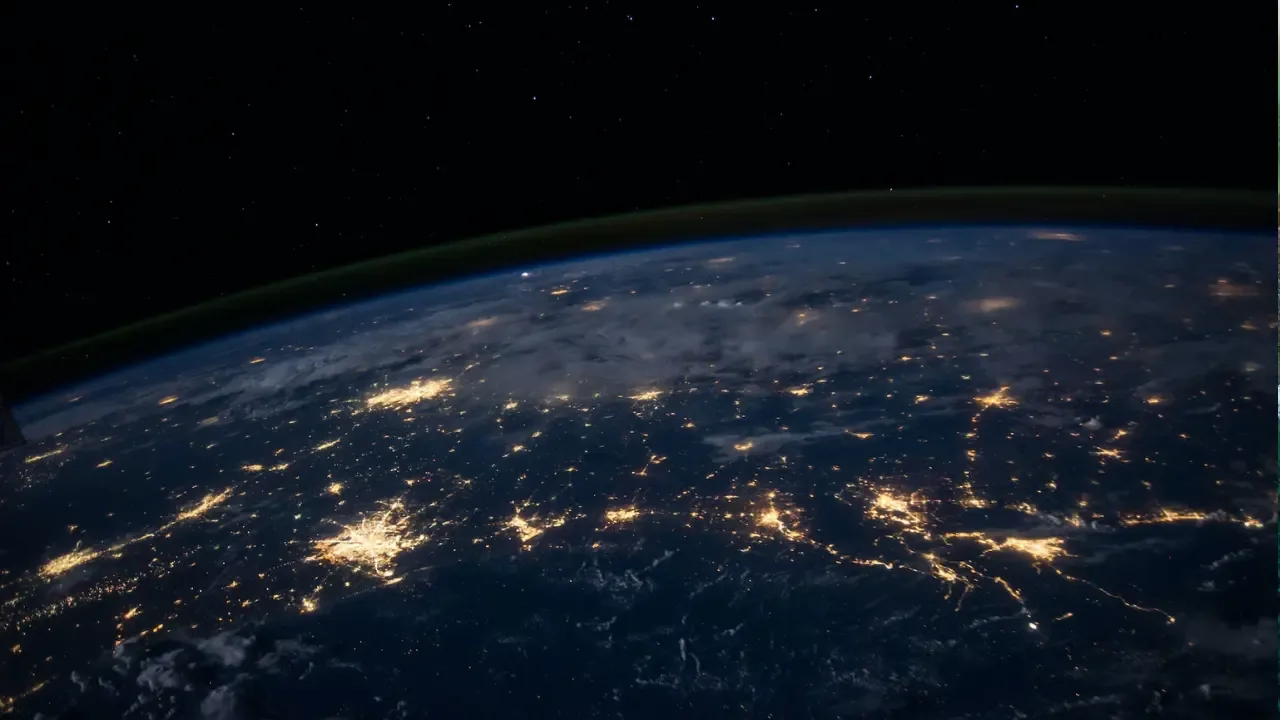
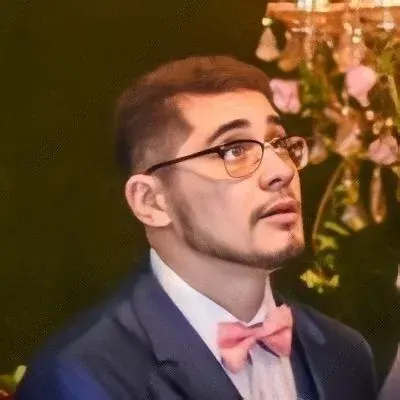
π‘ Title: Mastering Pandas: Groupby and Sort within Groups Made Easy
Are you struggling to group your pandas DataFrame by multiple columns and then sort the aggregated results within those groups? Look no further! In this blog post, we'll tackle this common issue with a step-by-step guide and provide you with easy solutions. πΌπͺ
Understanding the Problem
In the provided context, you have a DataFrame containing three columns: 'count', 'job', and 'source'. Your objective is to group the DataFrame by the 'job' and 'source' columns, aggregate the 'count' column by summing it up, and then sort the aggregated results within each group based on the 'count' column in descending order. Finally, you want to extract the top three rows from each group. Let's dive in!
Solution 1: Using the groupby
and apply
Method
One way to achieve the desired outcome is by using the .groupby()
method and applying a custom sorting and limiting function to each group. Here's how you can do it:
df.groupby(['job', 'source']).apply(lambda x: x.sort_values('count', ascending=False).head(3))
In this solution:
We use
.groupby(['job', 'source'])
to group the DataFrame by the 'job' and 'source' columns.Next, we apply a lambda function to each group using
.apply()
.Inside the lambda function, we use
.sort_values('count', ascending=False)
to sort the group by the 'count' column in descending order.Finally, we extract the top three rows from each group using
.head(3)
.
Solution 2: Chaining Methods for a Cleaner Approach
If you prefer a more concise and readable solution, you can leverage method chaining to accomplish the same result. Here's the clean version:
(df.groupby(['job', 'source'])
.apply(lambda x: x.sort_values('count', ascending=False).head(3))
)
This solution is identical to the first one, but we've eliminated the need for a separate line for each step by chaining the methods together. This approach can make your code more concise and easier to read.
Conclusion and Call-to-Action
Congratulations! You now know how to group your pandas DataFrame by multiple columns, sort the aggregated results within each group, and limit the output to the top three rows. πβ¨
Grouping and sorting within groups are powerful techniques that can help you extract meaningful insights from your data. Next time you encounter a similar task, remember the solutions we discussed in this blog post.
Now it's your turn! Put your newly acquired knowledge into practice. Try out these solutions with your own datasets and let us know how it goes in the comments below. π We'd love to hear about your experiences and any other pandas challenges you're facing.
Keep exploring, keep learning, and keep mastering pandas! πβ¨