pandas GroupBy columns with NaN (missing) values
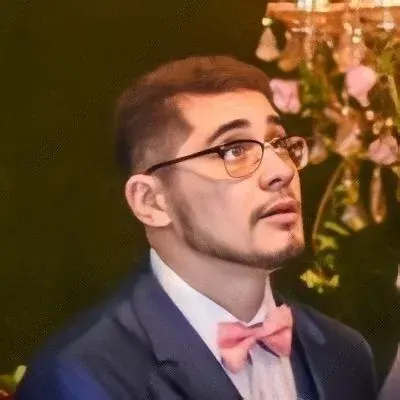
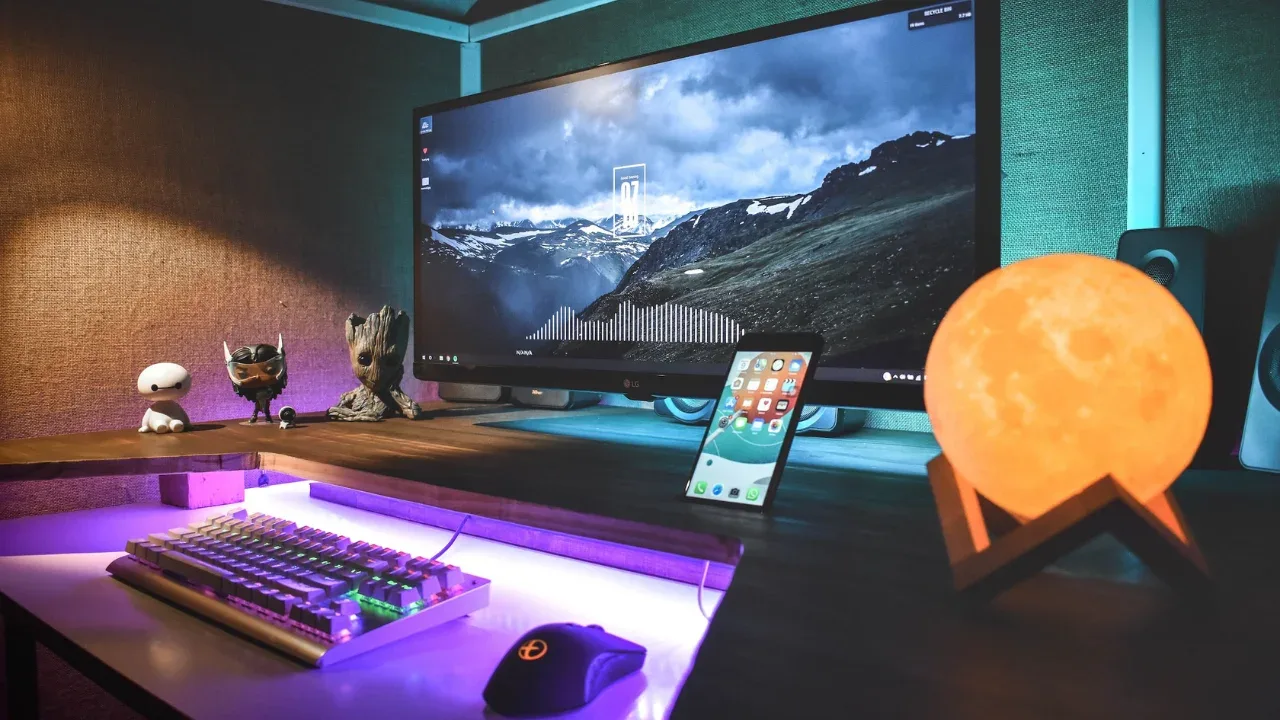
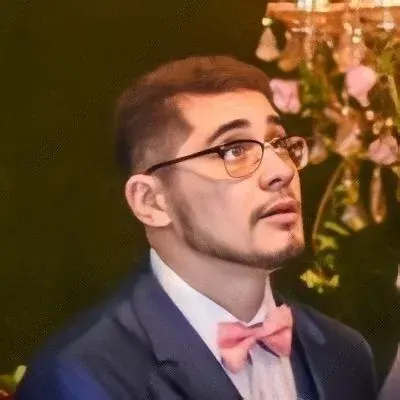
πΌπ Pandas GroupBy Columns with NaN (Missing) Values π€
Are you struggling with grouping your DataFrame columns that have NaN (missing) values? π Don't worry, we've got your back! In this blog post, we'll address this common issue and provide you with easy solutions π So, let's dive in and find out how to tackle this problem with Pandas π
First, let's set the context. You have a DataFrame with some missing values in columns that you wish to groupby. Here's an example to illustrate the situation:
import pandas as pd
import numpy as np
df = pd.DataFrame({'a': ['1', '2', '3'], 'b': ['4', np.NaN, '6']})
# Check the groups
df.groupby('b').groups
If you run the code above, you'll notice that Pandas has dropped the rows with NaN target values. π± But fear not, because we'll show you how to include these rows in your groupby operation! πͺ
π‘ Solution 1: Fill NaN with a Placeholder
One simple solution is to fill in the NaN values with a unique placeholder before performing the groupby operation. This placeholder will make sure that the rows with missing values are not dropped. Let's see how you can do it:
df.fillna('missing').groupby('b').groups
By using the fillna()
function, we replace NaN values with the string "missing". Now, if you run the groupby operation again, you'll see that the rows with missing values are included in the results. π
π‘ Solution 2: Groupby and Include NaN with a Special Indicator
If you prefer to keep track of the NaN values separately, you can use a special indicator when performing the groupby operation. Let's see how:
df.groupby(df['b'].fillna('missing', inplace=False)).groups
By using fillna()
within the groupby operation and passing inplace=False
, the NaN values will be replaced with "missing" only for the purpose of grouping. This way, you'll still have the original NaN values intact while including them in the groupby results. π
π Bonus Tip: Creating a Reusable Function
If you find yourself performing similar operations with multiple columns and complex functions, writing a reusable function might be a good idea! π You can encapsulate the steps discussed above into a function that can handle missing values for various columns and apply your desired function.
For example:
def groupby_with_missing(df, column, function):
df[column].fillna('missing', inplace=True)
return df.groupby(column).apply(function)
By creating a function like the one above, you can easily apply it to different columns with missing values and utilize your complex functions within the apply
step. This way, you keep your code clean and avoid repetition. β¨
π― Call-to-Action: Engage and Share
Now that you've learned how to groupby columns with NaN values in Pandas, it's time to put this knowledge into action! π Share this post with your fellow data enthusiasts who might be facing the same issue, and let them benefit from these easy solutions too! π¬
If you have any other Pandas questions or need further assistance, leave a comment below. Let's create a vibrant discussion and help each other grow! π
Happy coding with Pandas! πΌπ₯