Pandas get topmost n records within each group
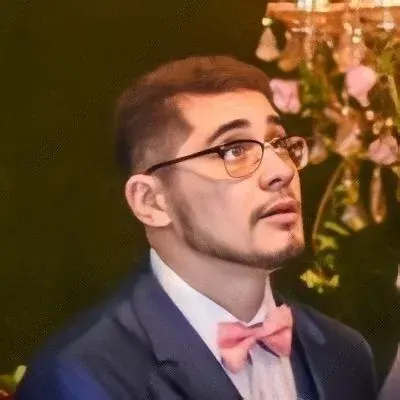
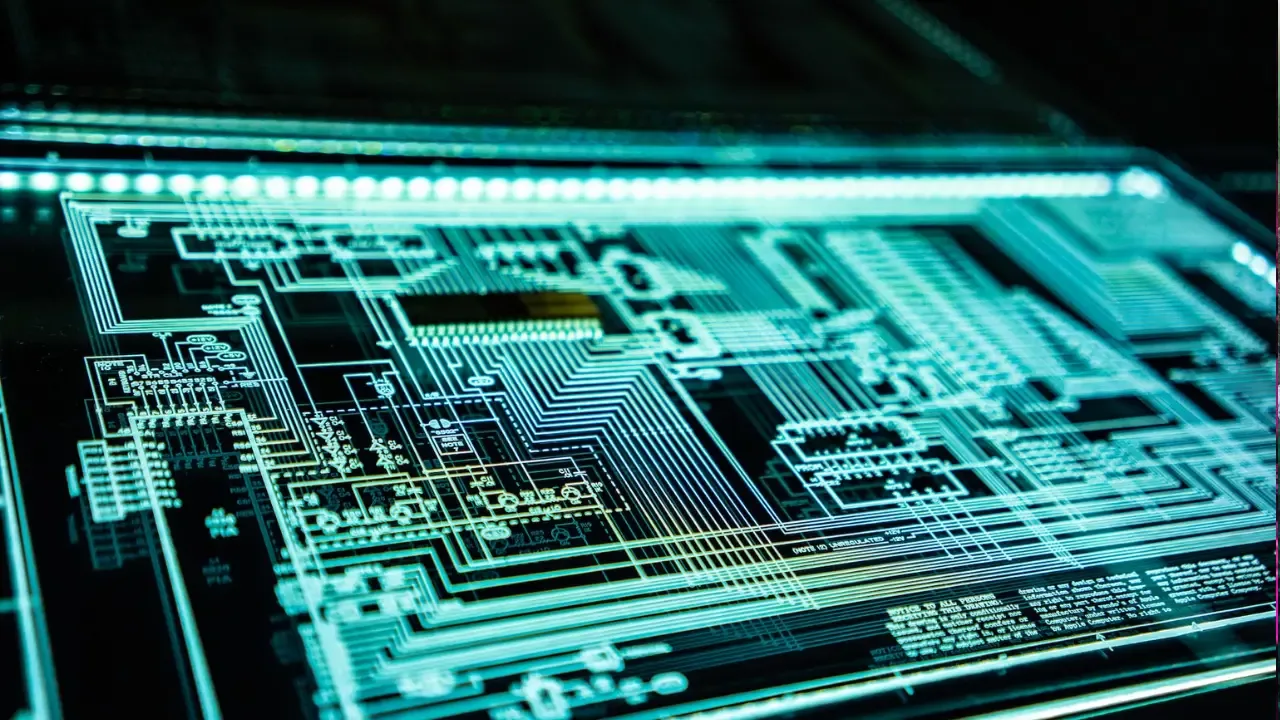
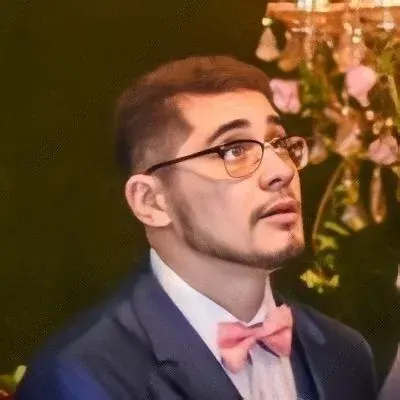
📚💻 Title: A Simple and Elegant Approach to Get the Topmost Records within Each Group in Pandas
Introduction:
So you have a pandas DataFrame and you want to extract the topmost records within each group? No worries, my tech-savvy friend, I've got you covered! In this blog post, we'll explore an effective and elegant approach to solve this problem using pandas. But wait, there's more! We'll also look at a sleek method to number records within each group, similar to the powerful window function in SQL. Let's dive in! 🏊♂️
The Problem:
Let's consider a pandas DataFrame that looks like this:
id value
0 1 1
1 1 2
2 1 3
3 2 1
4 2 2
5 2 3
6 2 4
7 3 1
8 4 1
Our goal is to obtain a new DataFrame containing the top 2 records for each unique id, like this:
id value
0 1 1
1 1 2
3 2 1
4 2 2
7 3 1
8 4 1
The Initial Approach:
The initial approach, which is functional but not as elegant, involved numbering records within groups. We used the groupby
function along with a lambda function to reset the index. Here's the code snippet:
dfN = df.groupby('id').apply(lambda x:x['value'].reset_index()).reset_index()
This resulted in a DataFrame that looked like this:
id level_1 index value
0 1 0 0 1
1 1 1 1 2
2 1 2 2 3
3 2 0 3 1
4 2 1 4 2
5 2 2 5 3
6 2 3 6 4
7 3 0 7 1
8 4 0 8 1
To obtain the desired output, we filtered the DataFrame using the 'level_1' column:
dfN[dfN['level_1'] <= 1][['id', 'value']]
A More Elegant Solution:
Now let's unveil a more elegant and efficient approach to tackle this problem. 🎩✨
You can achieve the same result without the need for the intermediate DataFrame dfN
by using the groupby
function with head(n)
. It's as simple as that! Let's see it in action:
df.groupby('id').head(2)[['id', 'value']]
This elegant solution directly filters the DataFrame based on each group's top n
records by using the head
function. And voila! You get the desired output without any additional steps.
Simulating SQL's Window Function - row_number()
:
If you're a fan of SQL's row_number()
window function, you'll be glad to know that pandas provides similar functionality! 🙌 With the help of the cumcount
function, we can conveniently simulate row_number()
within each group. Check out the code below:
df['row_number'] = df.groupby('id').cumcount() + 1
This will add a new column called 'row_number' to the DataFrame, indicating the row number within each group. Feel that SQL-like power? 😉
Conclusion and Call-to-Action:
You've now learned a simple and elegant approach to extract the topmost records within each group in pandas. No more convoluted steps or unnecessary intermediate DataFrames! Plus, we've shown you a nifty way to simulate SQL's row_number()
using cumcount()
.
Now go rock your pandas data manipulations like a boss! If you found this guide helpful, be sure to share it with your fellow pandas enthusiasts. Let's spread the data love! ❤️🐼
Please feel free to leave a comment or question below. How would you solve this problem? Do you have any other pandas tricks up your sleeve? Let's discuss and keep the pandas spirit alive! 🚀💬
Happy coding! 💻✨
[insert your call-to-action here]