pandas: filter rows of DataFrame with operator chaining
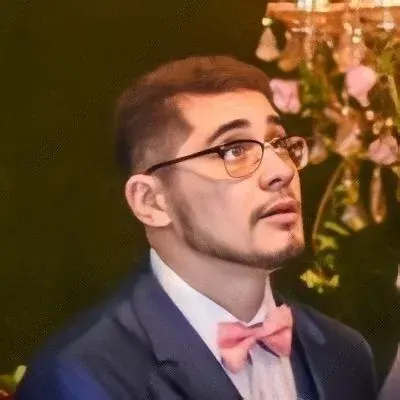
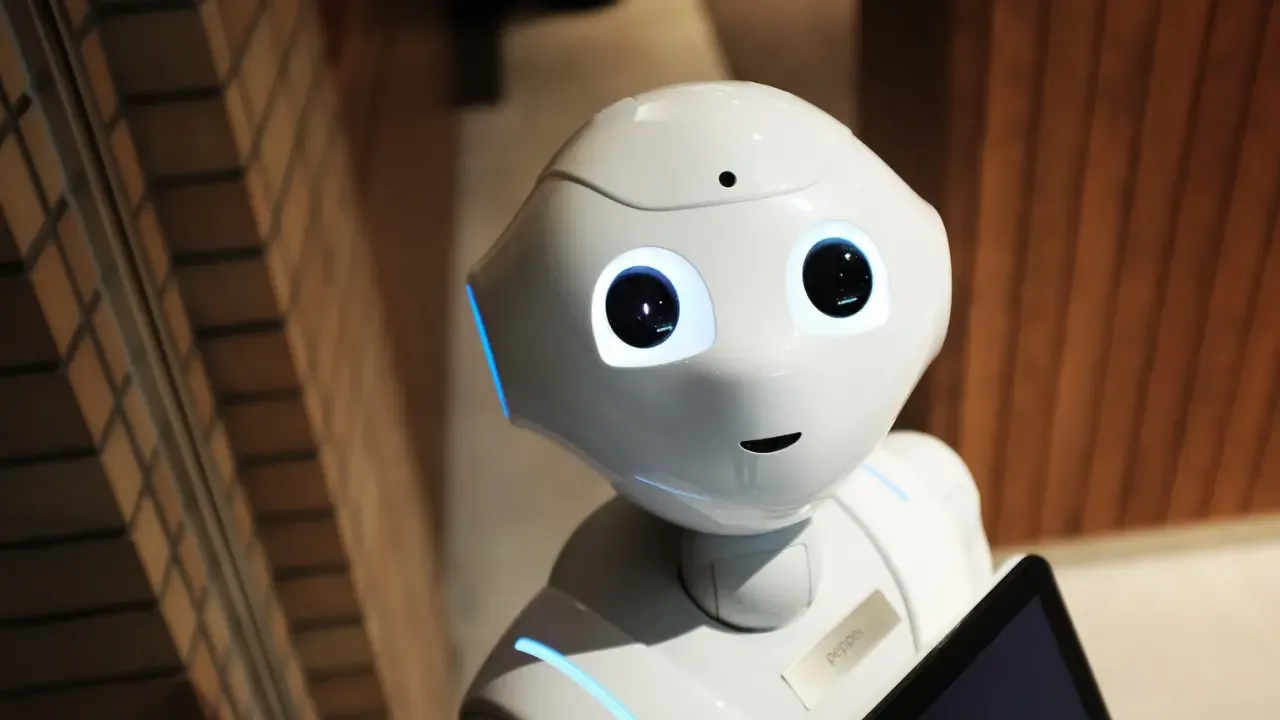
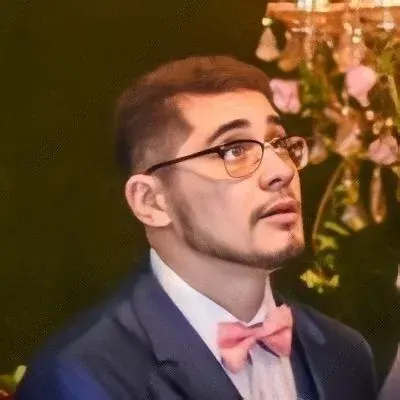
Filtering Rows in pandas: Operator Chaining to the Rescue! πΌπ
Have you ever found yourself wishing for a more streamlined way to filter rows in a pandas DataFrame? Look no further! π In this blog post, we'll explore how to use operator chaining to filter rows in pandas, addressing common issues along the way and providing easy solutions. Let's dive right in! π¦
The Traditional Approach π
Up until now, the most common way of filtering rows in pandas has been using bracket indexing. It sure gets the job done, but it can be a bit clunky and might not fit into your desired workflow. Here's an example of the traditional approach:
df_filtered = df[df['column'] == value]
While this works perfectly fine, it requires assigning the filtered DataFrame to a new variable, df_filtered
. This two-step process might not be the most appealing option for some of us.
Introducing Operator Chaining βοΈ
Luckily, pandas offers a much cleaner and efficient solution through operator chaining. With operator chaining, you can perform multiple operations on a DataFrame in a single line of code. Let's see how we can use operator chaining to filter rows in pandas, shall we? π
df_filtered = df[df['column'].eq(value)]
Isn't that just amazing? By chaining the .eq()
operator (which stands for "equals") with the column we want to filter, we can achieve the same result as before, but in a more elegant way. No need to assign a new variable separately - it's all done in one shot!
Take It a Step Further with .query()
π
If you enjoy the beauty of simplicity, you might even want to consider using the query()
method provided by pandas. This method allows you to filter rows using a more SQL-like syntax. Let me show you:
df_filtered = df.query("column == @value")
By leveraging the power of the query()
method, you can access variables directly within the query string using the @
symbol, making your code even more concise! π
Your Turn to Level Up β¬οΈ
π Wow! Now that you know how to use operator chaining to filter rows in pandas, it's time to take your skills to the next level! Go ahead and give it a try in your own projects. You'll be amazed at how much cleaner and more readable your code can become. Remember, simplicity is key! π
If you have any questions or want to share your experiences with pandas or operator chaining, feel free to leave a comment below. Let's start a discussion and learn from each other! Together, we can master the art of data manipulation with pandas. πΌπ₯
Happy coding! π»β¨