pandas DataFrame: replace nan values with average of columns
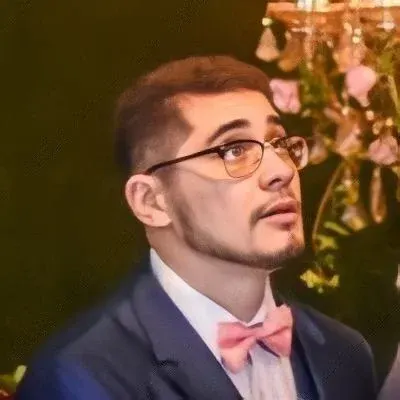
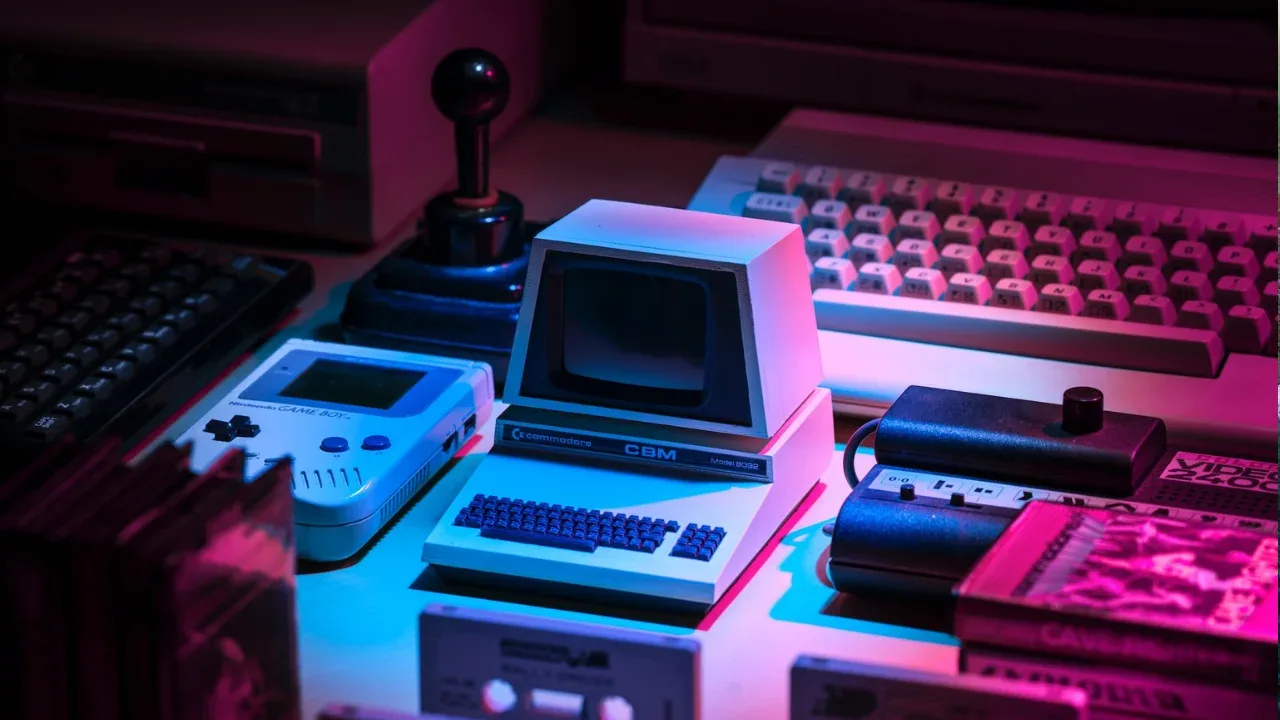
🐼 Pandas DataFrame: Replace NaN Values with Average of Columns
If you've been working with pandas DataFrames, you might have encountered the issue of having NaN (Not a Number) values in your data. These NaN values can occur due to various reasons such as missing data or data cleaning operations.
In this blog post, we will address the common question of how to replace NaN values in a pandas DataFrame with the average of the respective columns. We will provide you with easy solutions to tackle this problem and unleash the full potential of your data.
The Challenge
Let's start by understanding the problem at hand. Imagine you have a pandas DataFrame filled mostly with real numbers, but you notice a few NaN values scattered within. You want to replace these NaN values with the average value of their respective columns. So how do we go about it?
Solution 1: Using the fillna() Method
One intuitive solution is to utilize the fillna()
method provided by pandas DataFrame. This method allows us to replace NaN values with specified values. In our case, we want to replace them with the average of their respective columns. Here's how you can achieve it:
# Calculate the column-wise average
column_averages = df.mean()
# Replace NaN values with column averages
df.fillna(column_averages, inplace=True)
In the above code snippet, we first calculate the column-wise average using the mean()
method. We then use the fillna()
method to replace any NaN values with their respective column averages. The inplace=True
parameter ensures that the changes are made directly to the original DataFrame.
Solution 2: Using the fillna() Method with Dictionary
Another way to achieve the same result is by using a dictionary to map column names with their respective averages. Here's an example:
# Calculate the column-wise average
column_averages = df.mean()
# Create a dictionary mapping column names to column averages
average_dict = column_averages.to_dict()
# Replace NaN values with column averages using the dictionary
df.fillna(average_dict, inplace=True)
In this solution, we first calculate the column-wise average as before. Then, we convert the column averages into a dictionary using the to_dict()
method. Finally, we pass the dictionary to the fillna()
method to replace NaN values with their respective column averages.
Call-to-Action: Dive into the World of Data with pandas!
Replacing NaN values with the average of columns is just one of the many powerful capabilities of pandas. If you found this blog post helpful, imagine the possibilities that await you with pandas! Don't hesitate to explore more pandas functionalities and supercharge your data analysis skills.
Share your success stories or challenges faced while working with pandas DataFrames in the comments below. Let's engage in discussions, learn from each other, and unlock the full potential of our data!
Remember, with pandas, the world of data is at your fingertips. Happy coding! 😊🐼
Related question: How can I replace NaN values in a pandas DataFrame with zeros? Check out our blog post on "Pandas DataFrame: Replacing NaN Values with Zeros" to learn more!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
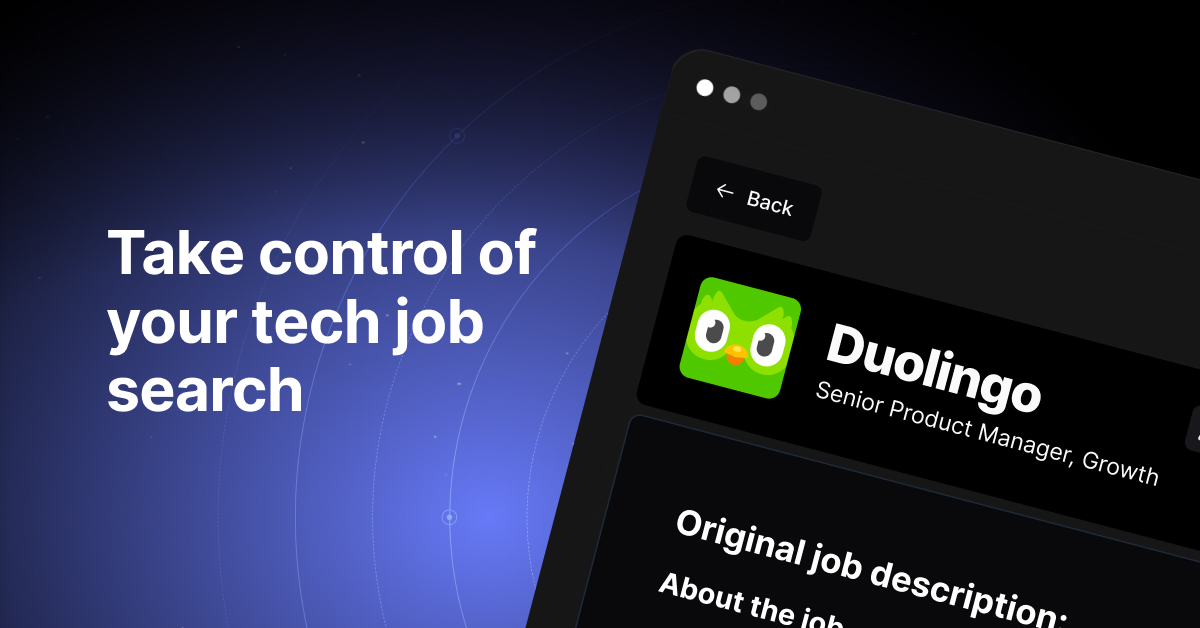