Pandas dataframe fillna() only some columns in place
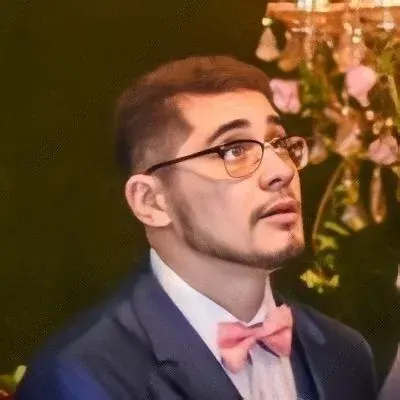
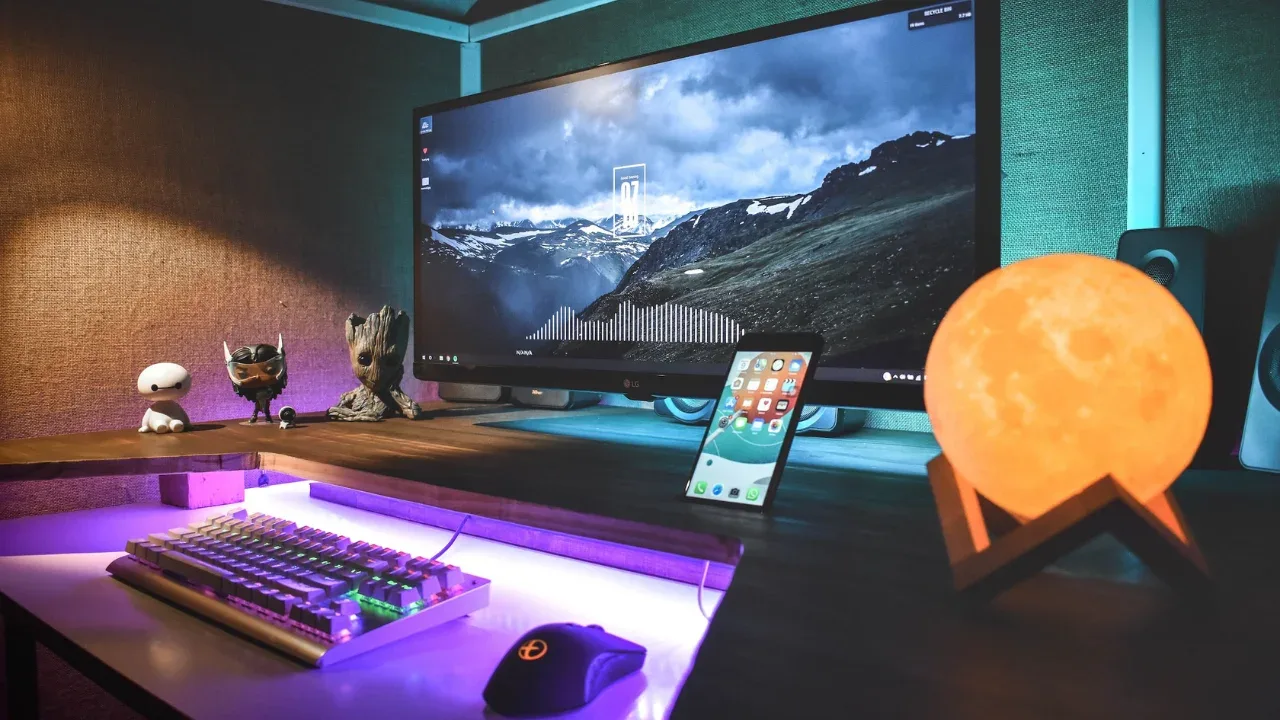
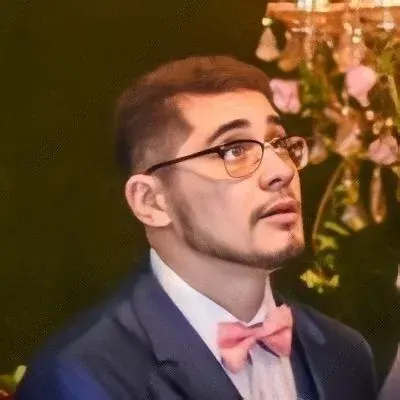
πΌπ‘ Pandas DataFrame fillna() Only Some Columns In Place ππ
Have you ever faced the challenge of filling NaN (None) values in a Pandas DataFrame, but only for specific columns? π€π This can be a common issue for data analysts and scientists working with large datasets. But fear not, as we have some easy solutions for you! Let's dive in and discover how we can accomplish this task effortlessly. πͺπ‘
First, let's take a look at the code provided by our dear fellow programmer:
import pandas as pd
df = pd.DataFrame(data={'a':[1,2,3,None],'b':[4,5,None,6],'c':[None,None,7,8]})
print(df)
df.fillna(value=0, inplace=True)
print(df)
This code demonstrates how to replace all NaN values with 0's in a DataFrame using the fillna()
method. However, it replaces every single NaN value, including those in column 'c', which might not be the behavior we desire. π
Now, let's uncover the best way to only replace NaN values in columns 'a' and 'b', but leave column 'c' untouched. πβ¨
One approach to achieve this is by using the fillna()
method in combination with the subset
parameter. The subset
parameter allows us to specify the columns we want to target for filling the NaN values. π―
Here's how you can modify the code to only replace NaN values in columns 'a' and 'b':
import pandas as pd
df = pd.DataFrame(data={'a':[1,2,3,None],'b':[4,5,None,6],'c':[None,None,7,8]})
print(df)
df[['a', 'b']] = df[['a', 'b']].fillna(value=0)
print(df)
By using double square brackets ([['a', 'b']]
), we access a subset of columns in the DataFrame: 'a' and 'b'. Then, we apply the fillna()
method specifically to this subset and assign the updated values back to the original DataFrame. ππΌ
Executing this revised code will yield the desired outcome: only NaN values in columns 'a' and 'b' get replaced with 0's, while column 'c' remains intact. ππ
Here's the final output you can expect to see:
a b c
0 1.0 4.0 NaN
1 2.0 5.0 NaN
2 3.0 0.0 7.0
3 0.0 6.0 8.0
Remember, using the subset
parameter with fillna()
allows you to easily target specific columns and only modify the NaN values within them. This technique will help you propel your data analysis and manipulation tasks forward without unnecessary headaches. π₯π‘
Now it's time for you to take action! Try implementing this method in your own code and see the magic happen. Share your experiences with us in the comments below! Let's learn and grow together. π©βπ»π¨βπ»
We hope this guide has been helpful to you. If you have any other questions or want to explore more about Pandas and data manipulation, check out our other blog posts or join our vibrant community. Together, we can conquer any data-related challenge! πͺπ
Happy coding! ππ»