Pandas create empty DataFrame with only column names
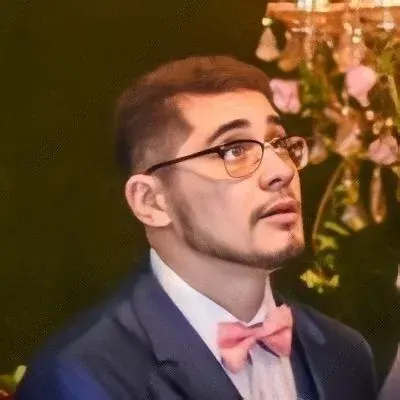
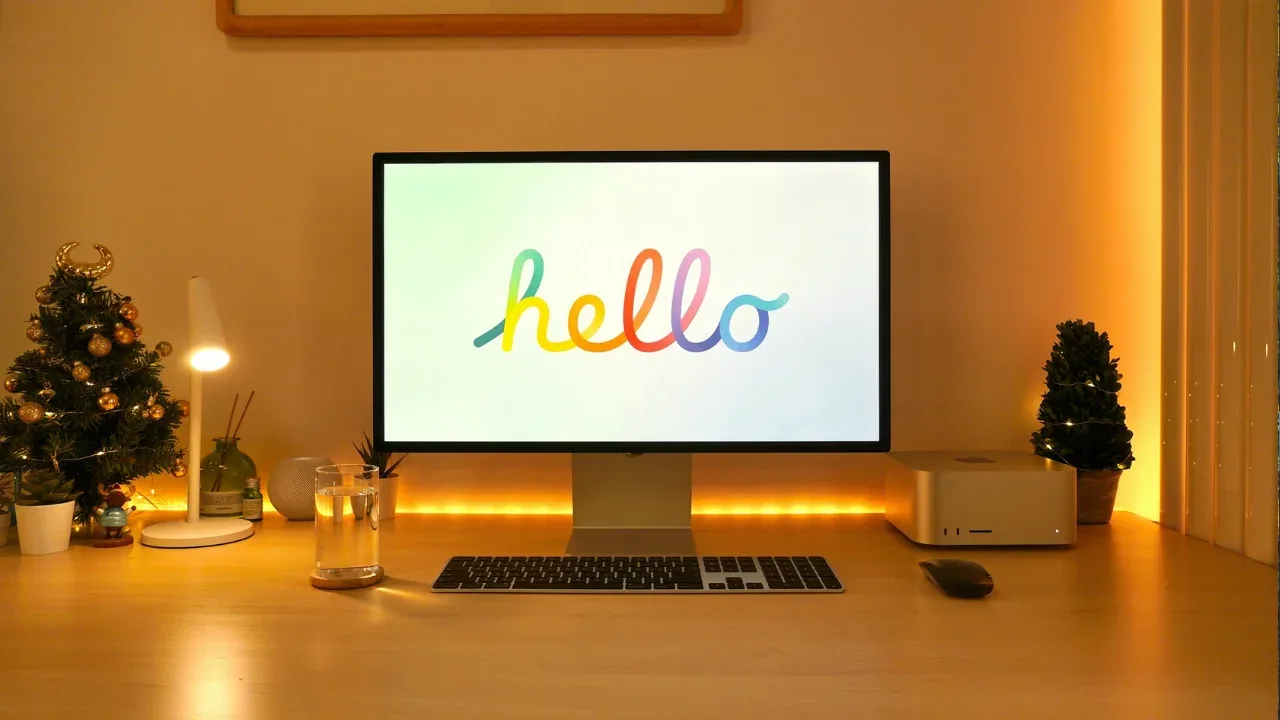
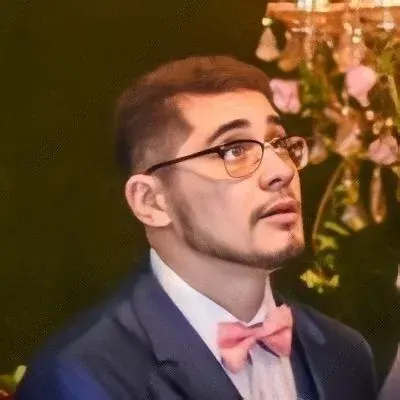
🐼 Pandas Tutorial: Creating an Empty DataFrame with Only Column Names
Are you encountering issues when trying to create an empty DataFrame in Pandas, with only column names? Don't worry, we've got you covered! In this tutorial, we will address the common problem of creating an empty DataFrame without losing the column names. We'll provide you with easy solutions and show you how to retain the column names, even when there are no row data to be inserted.
The Problem 😔
You might have a dynamic DataFrame that works perfectly fine, but you encounter an error when there are no data points to be added. So, how can you create an empty DataFrame in Pandas with only column names?
Currently, you might be using the following syntax:
df = pd.DataFrame(columns=COLUMN_NAMES) # Note that there is no row data inserted.
But the result you get looks like this:
Index([], dtype='object')
Empty DataFrame
The "Empty DataFrame" part is what you want, but instead of the "Index" thing, you need the column names to still be displayed.
The Solution 💡
To address this issue and retain the column names even in an empty DataFrame, you can make use of the following solution:
import pandas as pd
data = {}
for col in COLUMN_NAMES:
data[col] = []
df = pd.DataFrame(data)
By creating an empty dictionary data
and then dynamically assigning empty lists to each column name, you can create a DataFrame with only column names. This solution ensures that the column names are retained without any index displayed.
Let's break down the solution:
Import the Pandas library:
import pandas as pd
Create an empty dictionary,
data
:
data = {}
Iterate over the column names and assign empty lists to each column name:
for col in COLUMN_NAMES:
data[col] = []
Finally, create a DataFrame from the
data
dictionary:
df = pd.DataFrame(data)
Now, when you print or display the df
DataFrame, you will see:
Empty DataFrame
Columns: [column1, column2, column3, ...]
Index: []
Please note that the ..
in the column representation signifies more columns exist if you have a longer list of column names. Feel free to modify the solution to suit your specific needs.
Potential Cause: Converting to a PDF using Jinja2 📚
If you are converting the DataFrame to a PDF using Jinja2, you may encounter a situation where the column names get lost. This can happen when you call the df.to_html()
method before rendering it to a PDF.
To retain the column names during the conversion to PDF, you need to modify the way you render the DataFrame. Here's a solution that will preserve the column names:
from jinja2 import Environment, FileSystemLoader
from weasyprint import HTML
env = Environment(loader=FileSystemLoader('.'))
template = env.get_template("pdf_report_template.html")
template_vars = {"my_dataframe": df.to_html()}
html_out = template.render(template_vars)
HTML(string=html_out).write_pdf("my_pdf.pdf", stylesheets=["pdf_report_style.css"])
By passing the DataFrame in the template_vars
dictionary and rendering it using the Jinja2 template, you can ensure that the column names are retained in the final PDF output.
Conclusion and Call-to-Action 🚀
Creating an empty DataFrame with only column names in Pandas might seem tricky at first, but with the provided solutions, you can easily tackle this problem. By dynamically assigning empty lists to each column name and using the appropriate rendering methods for PDF conversion, you can retain the column names even when there are no row data to be added.
Next time you encounter this issue, remember these solutions and enjoy hassle-free DataFrame creation!
Feel free to share your thoughts and any other tricky Pandas problems you've encountered. Let's engage in the comments section below! 🎉