Pandas convert dataframe to array of tuples
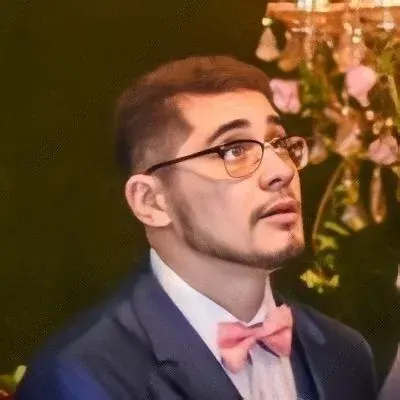
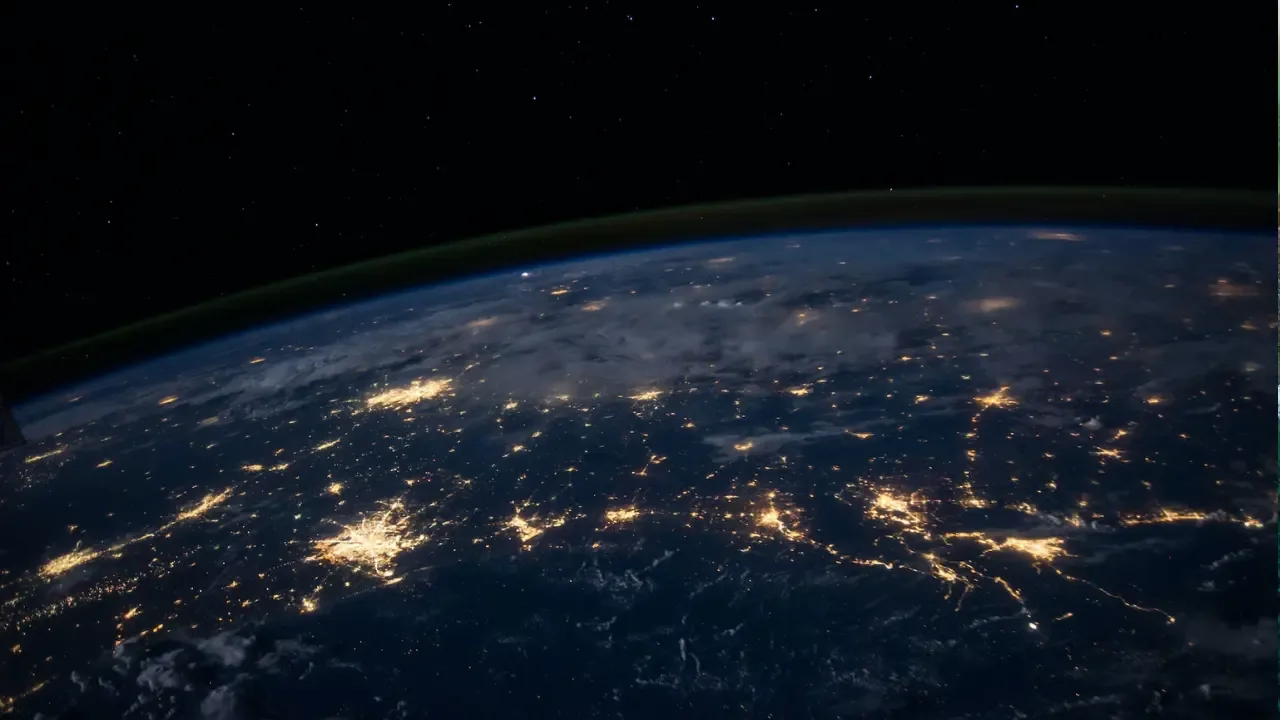
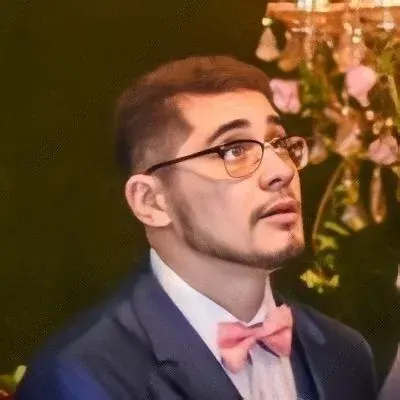
πΌπ» How to Convert a Pandas DataFrame to an Array of Tuples ππ
So you've been working with pandas and now you need to convert your dataframe into an array of tuples. Don't worry, I've got you covered! Let's dive into the solution step-by-step. π
First, let's look at the example dataframe you provided:
index data_date data_1 data_2
0 14303 2012-02-17 24.75 25.03
1 12009 2012-02-16 25.00 25.07
2 11830 2012-02-15 24.99 25.15
3 6274 2012-02-14 24.68 25.05
4 2302 2012-02-13 24.62 24.77
5 14085 2012-02-10 24.38 24.61
To convert this dataframe into an array of tuples, you can use the to_records()
method provided by pandas. This method returns a numpy structured array, which can then be easily converted into a list of tuples.
Here's how you can do it:
import pandas as pd
# Assuming your dataframe is called "data_set"
array_of_tuples = list(data_set.to_records(index=False))
That's it! The to_records()
method converts the dataframe into a structured array, and by passing index=False
as an argument, we exclude the index from the results. Then, we simply convert the structured array into a list of tuples.
In the case of your example dataframe, the resulting array_of_tuples
would be:
[(datetime.date(2012, 2, 17), 24.75, 25.03),
(datetime.date(2012, 2, 16), 25.0, 25.07),
(datetime.date(2012, 2, 15), 24.99, 25.15),
(datetime.date(2012, 2, 14), 24.68, 25.05),
(datetime.date(2012, 2, 13), 24.62, 24.77),
(datetime.date(2012, 2, 10), 24.38, 24.61)]
Pretty neat, right? π
Now that you have successfully converted your dataframe into an array of tuples, you can use this data for your batch save back to the database as you intended.
If you have any questions or face any issues while applying this solution, feel free to leave a comment below. I'm here to help you! π©βπ»π¬
Happy coding! π
Psst! Did you find this guide helpful? Share it with your fellow pandas enthusiasts and let's spread the pandas love! β€οΈπΌβ¨