open() in Python does not create a file if it doesn"t exist
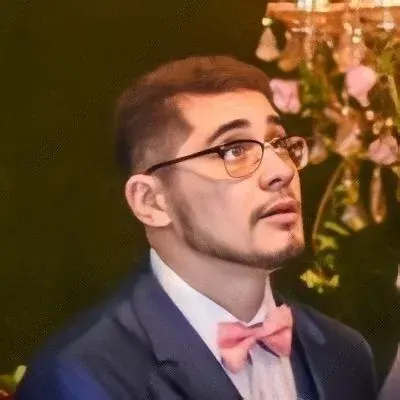
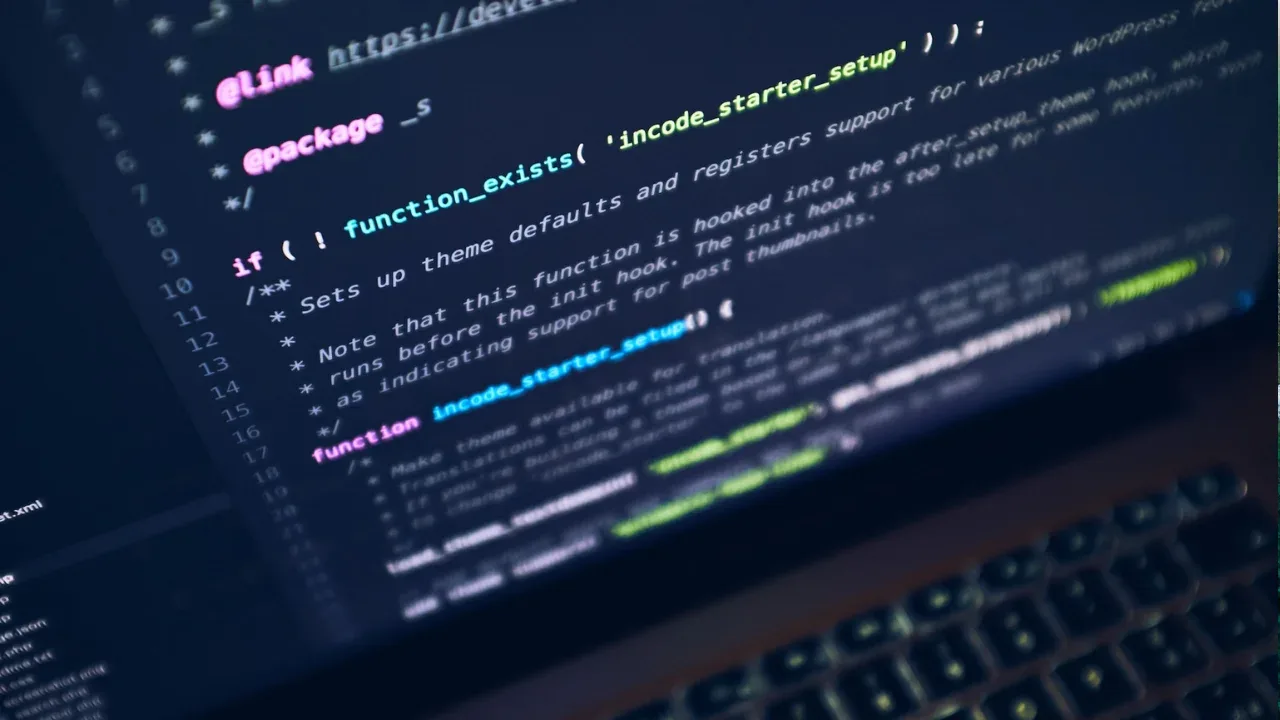
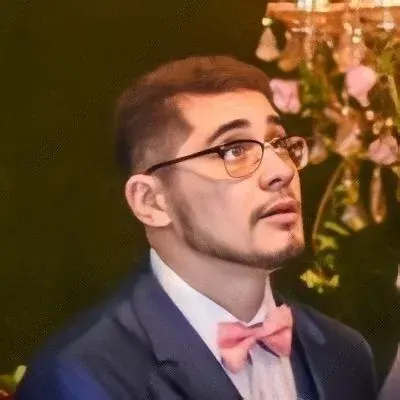
💡How to Use "open()" in Python to Create a File If It Doesn't Exist?
Have you ever encountered the frustrating "IOError: no such file or directory" when trying to open a file in Python? 😩 Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions to make your code work seamlessly. So let's dive right in! 🚀
The Problem 🕵️♀️
Imagine you want to open a file in Python for both reading and writing. If the file already exists, you want to open it; otherwise, you want to create a new file and open it. For this purpose, you might be tempted to use the following code:
file = open('myfile.dat', 'rw')
However, you quickly realize that this code snippet doesn't work as expected, resulting in the dreaded "IOError: no such file or directory" ⚠️. So what went wrong? 🤔
The Explanation 📝
The issue lies in the mode argument passed to the open()
function. In Python, there is no direct mode option like 'rw'
to specify read-write mode while creating a file if it doesn't exist. Instead, you need to use two separate options: 'r+'
for read-write mode and 'w+'
for write-read mode (which creates a new file if it doesn't exist).
The Solution ✅
To open a file in both read and write modes, and create it if it doesn't exist, use the following code:
try:
file = open('myfile.dat', 'r+')
except IOError:
file = open('myfile.dat', 'w+')
Here's what the code does:
It first tries to open the file in read-write mode (
'r+'
).If the file doesn't exist, an
IOError
is raised.In the
except
block, the code opens the file in write-read mode ('w+'
), which creates the file if it doesn't exist.Now you can continue using the
file
object for reading and writing.
A Note on Python Versions 🐍
The code snippet above works for Python 2.6.2 and above. However, starting from Python 3.0, the open()
function in write mode ('w'
and 'w+'
) can create files if they don't exist. So if you're using Python 3.0 or later, the initial code you tried (file = open('myfile.dat', 'rw')
) would actually work correctly.
Time to Take Action! ⏰
Now that you know how to solve the issue of opening a file in Python, it's time to put your new knowledge into practice! Update your code by using the provided solution and say goodbye to the "no such file or directory" error once and for all. 🎉
If you found this blog post helpful, please do share it with your fellow Python enthusiasts. Likewise, if you have any further questions or alternative solutions, feel free to leave a comment below. Let's keep the Python community buzzing with knowledge sharing! 🐍💬
Happy coding! 💻