NumPy array is not JSON serializable
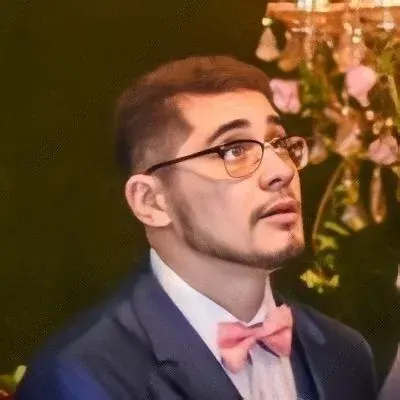
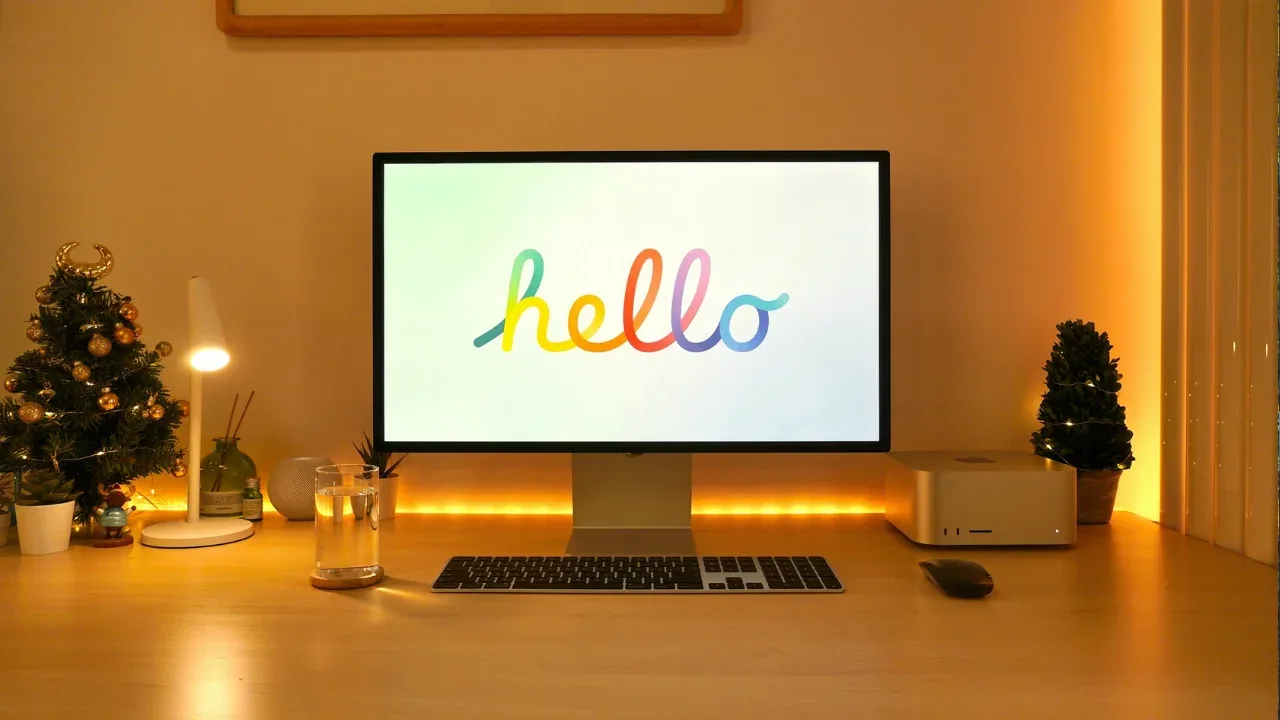
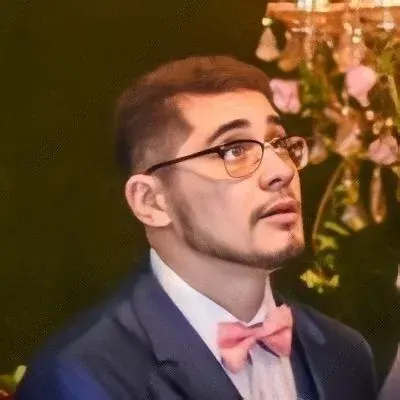
Title: 🧩 Decoding the Mystery: Why is your NumPy Array not JSON Serializable? 📦
Introduction: Are you facing the frustrating error message 🚫 "array([ 0, 239, 479, 717, 952, 1192, 1432, 1667], dtype=int64) is not JSON serializable" 🤔 when trying to save a NumPy array as a Django context variable? Don't worry; we've got you covered! In this blog post, we will explore the common issues behind this problem, provide you with easy solutions, and ultimately, help you conquer this challenge! Let's dive in! 💪
Understanding the Error:
To begin, let's unravel the meaning behind the error message 🛠️. When you encounter the phrase "is not JSON serializable," it implies that the Python object you are attempting to convert (in this case, a NumPy array) cannot be directly converted into a JSON format, which is required for serialization and transmission. Python's built-in json
module is unable to handle NumPy arrays inherently.
Common Causes: There are a few reasons why your NumPy array might not be JSON serializable. Let's address the most common ones:
NumPy-specific Array: If your array has NumPy-specific attributes, such as a specific data type or shape, it becomes non-serializable out of the box. JSON can only handle basic data types like integers, strings, dictionaries, etc.
Circular References: Circular references between NumPy arrays or instances referencing each other will lead to serialization failure. JSON allows only flat data structures and cannot manage recursive references.
Easy Solutions: Now, let's unravel some simple yet effective solutions to tackle this conundrum! 🎉
Solution 1: Convert NumPy Array to a Python List
One way to make your NumPy array serializable is by converting it into a Python list using the .tolist()
method. This will strip away any NumPy-specific attributes, converting it into a basic Python data structure that can be easily serialized.
import numpy as np
numpy_array = np.array([0, 239, 479, 717, 952, 1192, 1432, 1667], dtype=np.int64)
serializable_list = numpy_array.tolist()
Solution 2: Custom JSON Encoder
Another approach is to create a custom JSON encoder class that extends the json.JSONEncoder
class and overrides the default()
method. This way, you can handle the NumPy array serialization yourself.
import json
import numpy as np
class NumpyArrayEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, np.ndarray):
return obj.tolist() # Convert NumPy arrays to lists
return super().default(obj)
numpy_array = np.array([0, 239, 479, 717, 952, 1192, 1432, 1667], dtype=np.int64)
json_serializable = json.dumps(numpy_array, cls=NumpyArrayEncoder)
Conclusion and Call-to-Action: Congratulations! You've just decoded the mystery behind the "NumPy array is not JSON serializable" dilemma! Now, armed with practical solutions, you can effortlessly transform your NumPy arrays into JSON serializable objects. 💡
In our tech-savvy world, knowledge is best shared! If you found this blog post helpful, don't hesitate to share it with your programmer friends 🔀. Let us know your thoughts and experiences in the comments section below ⬇️. Together, we can conquer any coding obstacle that comes our way!
Stay tuned for more exciting Python tricks and tips, and happy coding! 🐍✨