Null object in Python
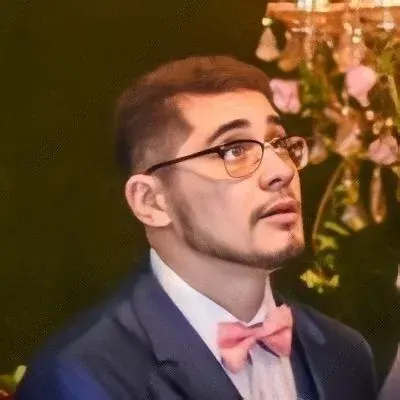
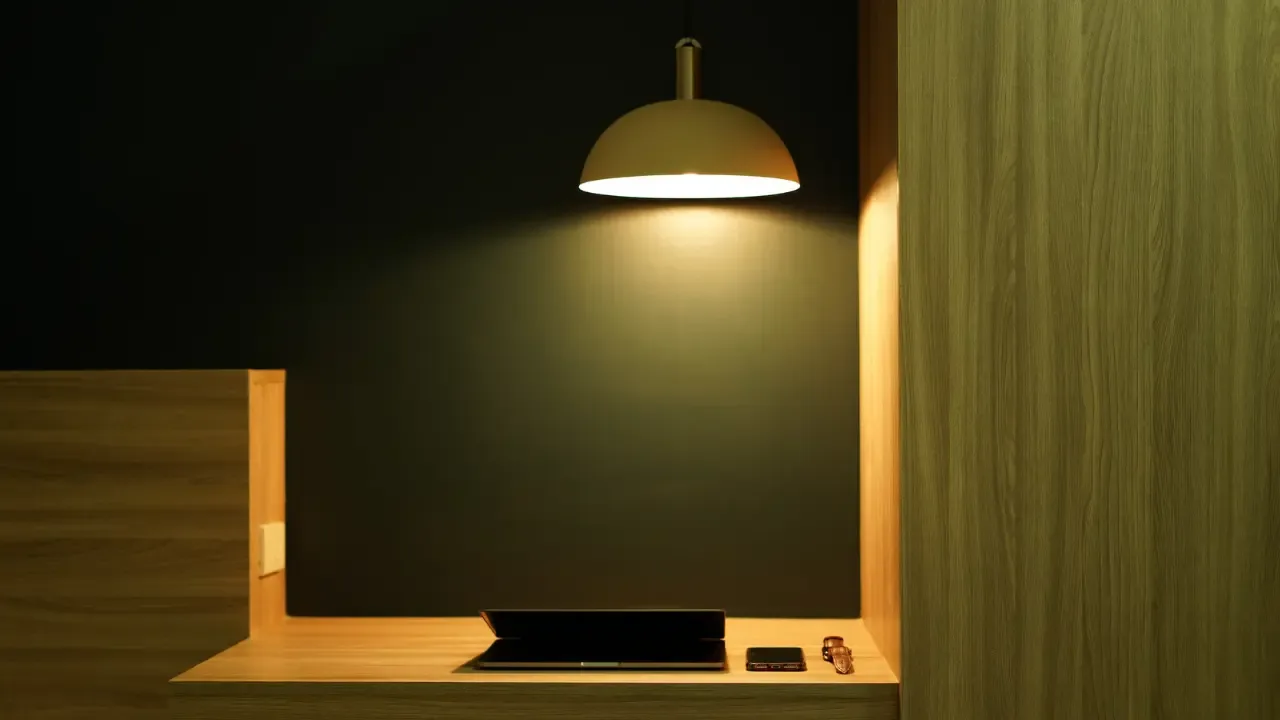
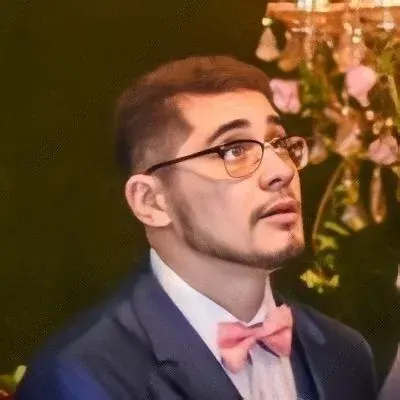
The Null Object in Python: Unlocking the Mystery! 🎩🔎
Have you ever encountered a situation where you needed to refer to a non-existent object in Python? 🤔 Don't worry, you're not alone! Many developers have faced this challenge before. In Python, the concept of a "null object" is known as None
. 🐍
What is the Null Object? 🤷♀️
The null object, also referred to as a null pointer, represents the absence of a value. It is a way to indicate that something does not exist or is unknown. In Python, None
serves as the null object, and it is a unique data type of its own. 📛
Common Issues and Challenges with the Null Object 😩
1. Comparing with None
✅❌
One common challenge developers face is mistakenly using the equality operator (==
) when comparing a variable with None
. Remember that None
is an object itself, so you should use the identity operator (is
) to check if a variable is indeed referring to None
. For example:
x = None
if x is None:
print("x is the null object!")
2. Unintended Side Effects 🔄
Assigning None
to a variable can lead to unintended side effects if you're not careful. For instance, if you accidentally pass None
as a parameter to a function that expects a valid argument, it may result in unexpected behavior or even errors. Make sure to double-check your code to avoid these surprises. 🔍
3. Null Object in Data Structures 🗂️
When working with data structures like lists or dictionaries, understanding how to handle the null object becomes even more crucial. For example, if you want to check if a key exists in a dictionary, you can utilize the get()
method, which returns None
by default if the key is not found.
my_dict = {"name": "John", "age": 25}
# Using get() method to access a key
salary = my_dict.get("salary")
if salary is None:
print("The salary is unknown.")
How to Handle the Null Object 💪🔧
1. Default Values with or
Operator 👍
You can utilize the or
operator to set a default value if a variable evaluates to None
. This technique is concise and can simplify your code. Take a look:
name = None
final_name = name or "Default"
print(final_name) # Output: "Default"
2. Conditional Statements 🔄🔍
Another approach is to use conditional statements to handle the null object case separately. By explicitly checking if a variable is None
, you can define logic specifically for that scenario. Here's an example:
x = None
if x is None:
# Handle the null object case
print("x is the null object!")
else:
# Handle the other cases
print("x is not the null object.")
Join the Null Object Revolution! 💪🚀
Now that you have a better understanding of the null object in Python, it's time to put your knowledge into action! Embrace the null object and make better, more robust code. 🙌
If you found this blog post helpful, don't forget to share it with your fellow developers. Let's spread the word and make programming a null-object-friendly experience for everyone! 💻❤️
Got any questions or additional tips to share? Leave a comment below and let's spark a discussion! 👇🎉