Multiple aggregations of the same column using pandas GroupBy.agg()
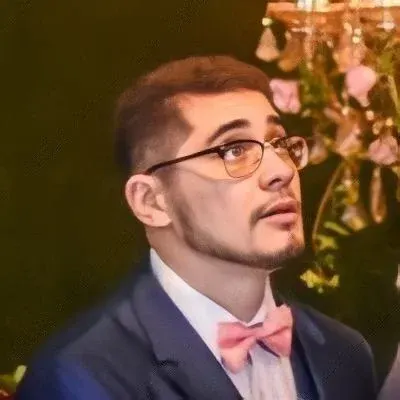
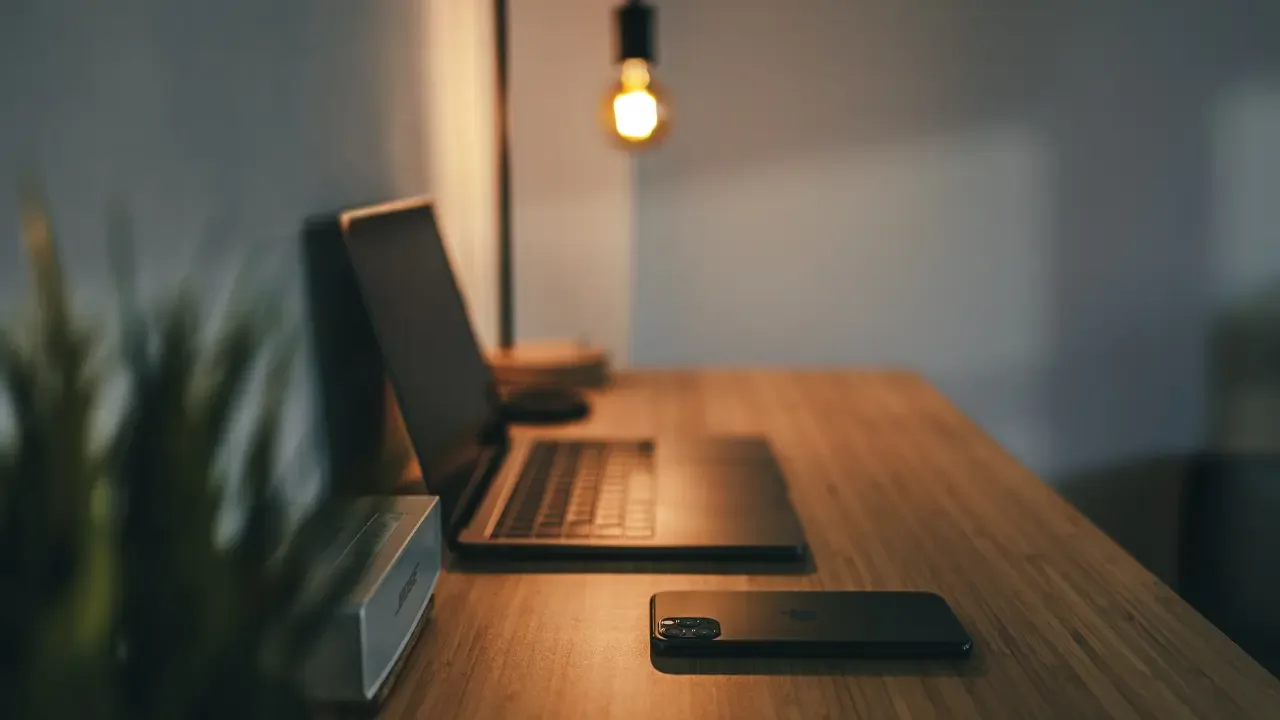
๐ Multiple Aggregations of the Same Column using pandas GroupBy.agg()
Have you ever found yourself in a situation where you need to apply multiple aggregating functions to the same column in your pandas DataFrame, but you don't want to call agg()
multiple times? If so, you're in the right place! In this blog post, we'll explore a common issue related to this question and provide you with easy solutions. So let's dive in and find out the best way to tackle this problem! ๐ช
The Problem
Let's begin by discussing the problem at hand. Assume we have a DataFrame called df
with columns date
, returns
, and dummy
. We want to apply two different aggregating functions, f1
and f2
, to the returns
column. However, the straightforward approach seems syntactically wrong:
df.groupby("dummy").agg({"returns": f1, "returns": f2})
This code snippet won't work as expected because Python doesn't allow duplicate keys in dictionaries. ๐
A Possible Solution
Now you might be wondering, "Is there any other way to express the input to agg()
that allows multiple functions to be applied to the same column?" And the answer is yes! Although agg()
seems to only accept a dictionary, we can use a clever workaround using a list of tuples. ๐
Here's how you can do it:
df.groupby("dummy").agg([("returns", f1), ("returns", f2)])
By using a list of tuples instead of a dictionary, we can specify the column name and the corresponding aggregating function for each tuple. This solution allows us to apply both f1
and f2
to the returns
column without any issues. ๐
Bonus Tip: The Power of Auxiliary Functions
But hey, what if you were wondering about how this would work with aggregation in general? Good question! When dealing with more complex aggregations, defining an auxiliary function can be a powerful tool. ๐ ๏ธ
Let's say you had multiple columns and multiple aggregating functions to apply. You can create an auxiliary function that takes care of applying all the functions as needed. Here's an example:
def aggregate_func(column):
return column.agg([("f1_result", f1), ("f2_result", f2)])
df.groupby("dummy").apply(aggregate_func)
In this example, the aggregate_func()
function applies f1
and f2
to the specified column. You can then use groupby().apply()
to apply this function to all groups in your DataFrame. This approach gives you the flexibility to handle more complex scenarios while keeping your code clean and maintainable. ๐งน
Conclusion
In this blog post, we explored the question of applying multiple aggregating functions to the same column using pandas' GroupBy.agg()
method. We learned about the syntactical limitation of duplicate keys in a dictionary and discovered a clever solution using a list of tuples. We also discussed the power of auxiliary functions when dealing with more complex aggregations. ๐
Now that you know how to overcome this common issue, go ahead and apply multiple aggregating functions to your columns like a pro! If you have any questions or suggestions, feel free to leave a comment below. Happy coding! ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
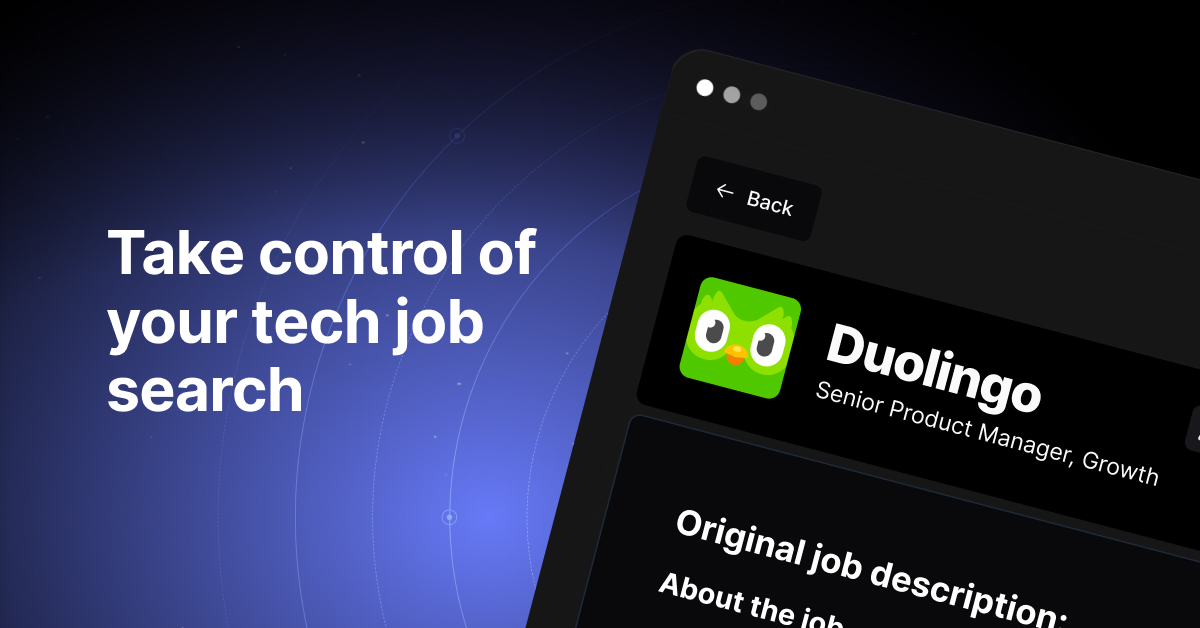