mongodb: insert if not exists
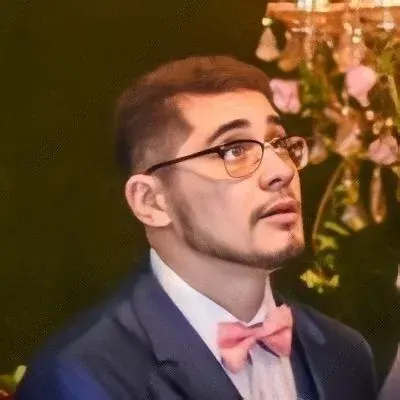
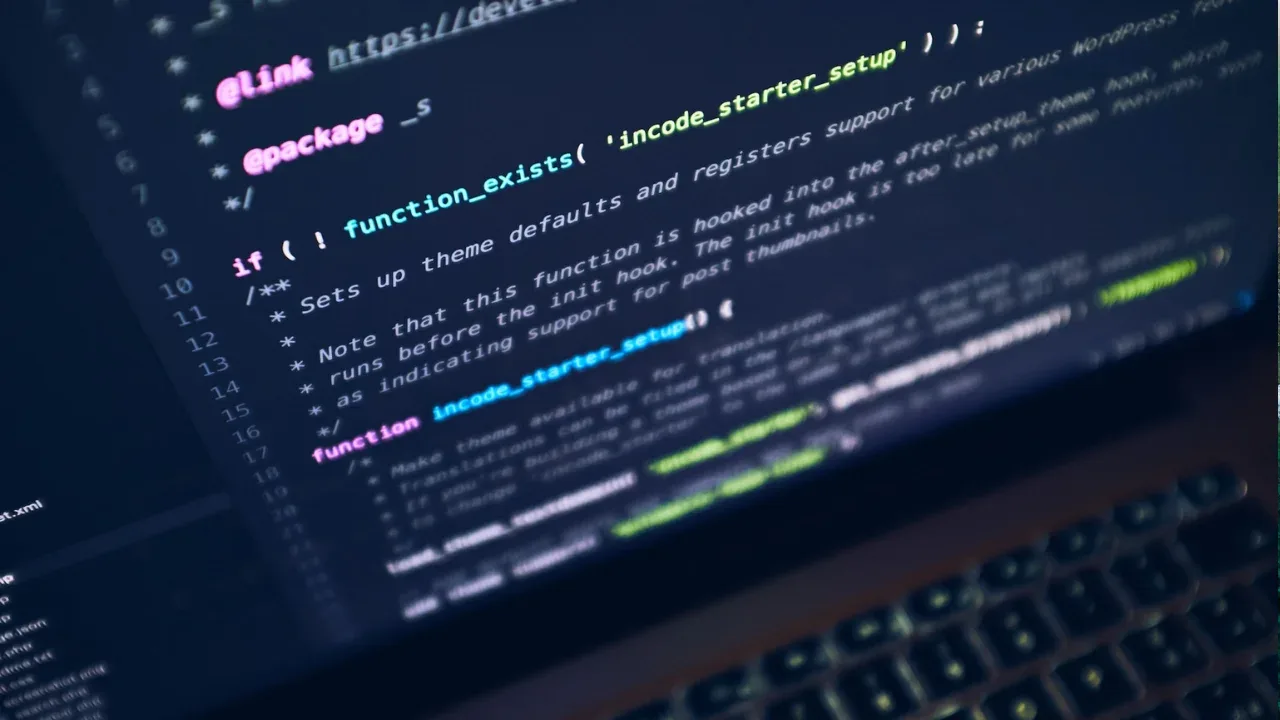
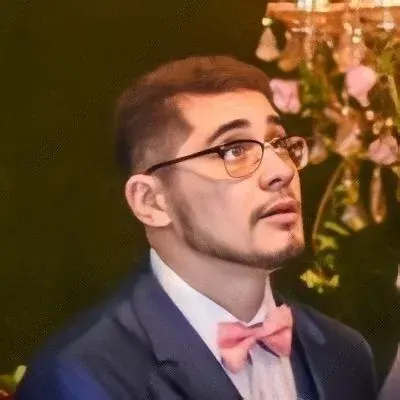
MongoDB: Insert if Not Exists - A Fast and Efficient Solution
š¢ Hey there tech enthusiasts! Are you struggling with the tedious process of inserting new documents in MongoDB while ensuring no duplicates and maintaining updated timestamps? Well, worry no more! In this blog post, we'll dive into the problem our fellow developer is facing and provide you with a super-fast and efficient solution using the Python driver (pymongo). Let's get started! š
The Problem
Our friend receives a daily stock of documents and needs to insert each item that does not already exist. Pretty straightforward, right? But here are the specific requirements:
He wants to track the first time a document is inserted and its last update time within the update.
No duplicate documents should be stored.
Existing documents, not present in the update, should remain untouched.
A significant portion of the records remains unmodified day-to-day.
The Current Approach
Here's the pseudo-code our friend is using:
for each document in update:
existing_document = collection.find_one(document)
if not existing_document:
document['insertion_date'] = now
else:
document = existing_document
document['last_update_date'] = now
my_collection.save(document)
Now, this approach gets the job done, but it's painstakingly slow. For less than 100,000 records, it takes around 40 minutes! With millions of records in the update, it becomes an unbearable process. š«
A Faster Solution
Fortunately, MongoDB provides a built-in method to handle such scenarios - update_many()
with the upsert
option, which inserts a document if it doesn't exist and updates it otherwise. Let's revamp our friend's code using this efficient approach:
for each document in update:
filter = document.copy()
filter.pop('_id', None) # Exclude '_id' field from check
update = {
'$set': {
'last_update_date': now
},
'$setOnInsert': {
'insertion_date': now
}
}
collection.update_many(filter, update, upsert=True)
š That's it! With just a few changes, our friend's insert process will go from minutes to mere seconds.
In the updated code:
We create a filter from the document by making a copy and excluding the
_id
field. This ensures that the_id
field, if present, won't affect the upsert behavior.We define the
update
object with two modifiers:$set
sets thelast_update_date
to the current timestamp.$setOnInsert
sets theinsertion_date
only during the insert operation, not during updates.
Finally, with the
update_many()
method andupsert=True
, MongoDB handles the insert/update operation for each document efficiently.
Time to Fly āļø
And there you have it folks - a lightning-fast solution to the problem of inserting documents in MongoDB without duplicates, keeping track of timestamps, and handling updates with ease! Say goodbye to lengthy waiting times and start leveraging the power of update_many()
with upsert
.
If you found this guide helpful, we'd love to hear your thoughts! Have you faced similar challenges with MongoDB? Do you have any other cool tips and tricks for optimizing database operations? Don't hesitate to share your experiences and suggestions in the comments below. Let's dive into the conversation! š¬
Keep coding! šØāš»š©āš»