Merge two dataframes by index
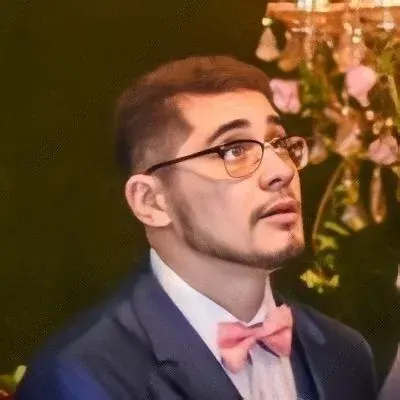
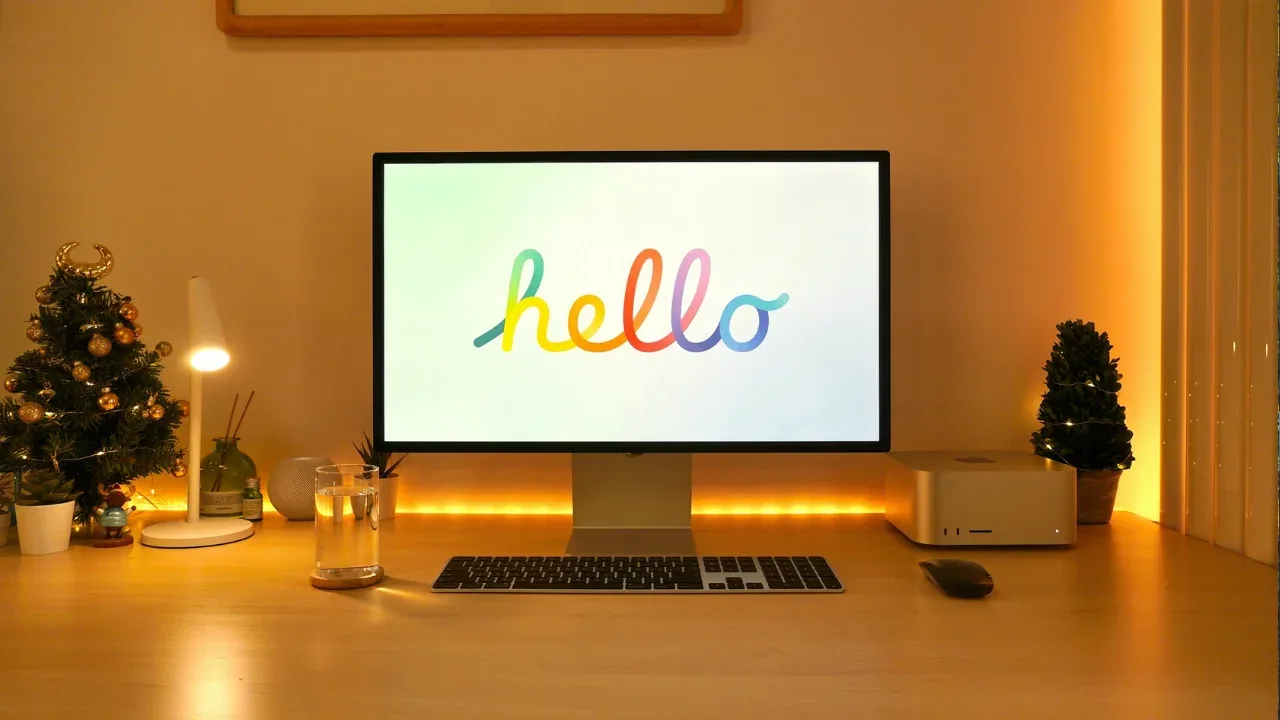
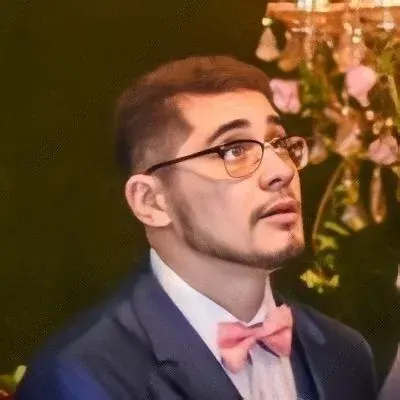
Merging Dataframes by Index: A Simple Guide 👥📊
So, you have two dataframes that you want to merge, but the problem is that you want to merge them based on their indices. You might be thinking, "Can I even do that?" 🤔 Well, worry not! In this guide, we will address this common concern and provide you with easy solutions to achieve your goal.
But first, let's set the stage 🎭. Imagine you have the following dataframes:
df1 = pd.DataFrame({
'id': [278, 421],
'begin': [56, 18],
'conditional': [False, False],
'confidence': [0.0, 0.0],
'discoveryTechnique': [1, 1]
})
df2 = pd.DataFrame({
'concept': ['A', 'B']
})
You want to merge these dataframes on their indices, so the output should look like this:
id begin conditional confidence discoveryTechnique concept
0 278 56 False 0.0 1 A
1 421 18 False 0.0 1 B
The Issue: Merge on Indices 💔
If you're familiar with the merge()
function in pandas, you might be tempted to try something like df1.merge(df2)
to achieve the desired result. However, doing this will throw an error that might look something like this:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/usr/local/lib/python2.7/dist-packages/pandas/core/frame.py", line 4618, in merge
copy=copy, indicator=indicator)
File "/usr/local/lib/python2.7/dist-packages/pandas/tools/merge.py", line 58, in merge
copy=copy, indicator=indicator)
File "/usr/local/lib/python2.7/dist-packages/pandas/tools/merge.py", line 491, in __init__
self._validate_specification()
File "/usr/local/lib/python2.7/dist-packages/pandas/tools/merge.py", line 812, in _validate_specification
raise MergeError('No common columns to perform merge on')
pandas.tools.merge.MergeError: No common columns to perform merge on
The Solution: Matching Indices Like a Pro! 💡
Now that we've identified the problem, let's dive into the solutions! Here are three ways you can merge dataframes based on their indices:
1. Using the join()
Method 🤝
Pandas provides a handy join()
method that allows you to merge dataframes on their indices. You can achieve the desired output by simply using:
result = df1.join(df2)
Easy, right? 😎
2. Resetting and Merging the Indices 🔀
In some cases, you might want to include the indices as a separate column in your merged dataframe. To achieve this, you can reset the indices of both dataframes, merge them, and then assign the merged index column back to the dataframe. Here's how it's done:
df1_reset = df1.reset_index()
df2_reset = df2.reset_index()
# Merge the dataframes
result = df1_reset.merge(df2_reset, on='index')
# Clean up the resulting dataframe
result = result.drop(columns='index')
That's it! You now have the merged dataframe with the indices included as a separate column.
3. Using the concat()
Function 🔄
If you're dealing with multiple dataframes that need to be merged on their indices, the concat()
function can be helpful. This function allows you to concatenate dataframes along a particular axis. In our case, we want to concatenate them vertically, so we specify axis=0
. Here's how you can use it:
result = pd.concat([df1, df2], axis=0)
Wrapping Up and Taking Action 🎁
And there you have it! You now know how to merge dataframes based on their indices using simple and effective techniques. No more MergeError messages! 🎉
Feel free to experiment with these methods and apply them to your specific use cases. If you have any questions or other pandas-related topics you'd like us to cover, let us know in the comments below! 👇
Now, it's your turn! Try merging some dataframes by their indices and share your experience with us. We'd love to see what you come up with! 💪
Happy coding! 💻✨
References and Further Reading: