Meaning of @classmethod and @staticmethod for beginner
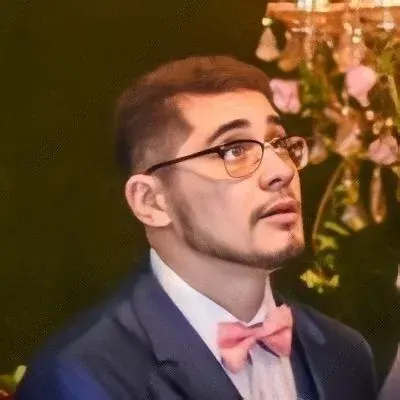
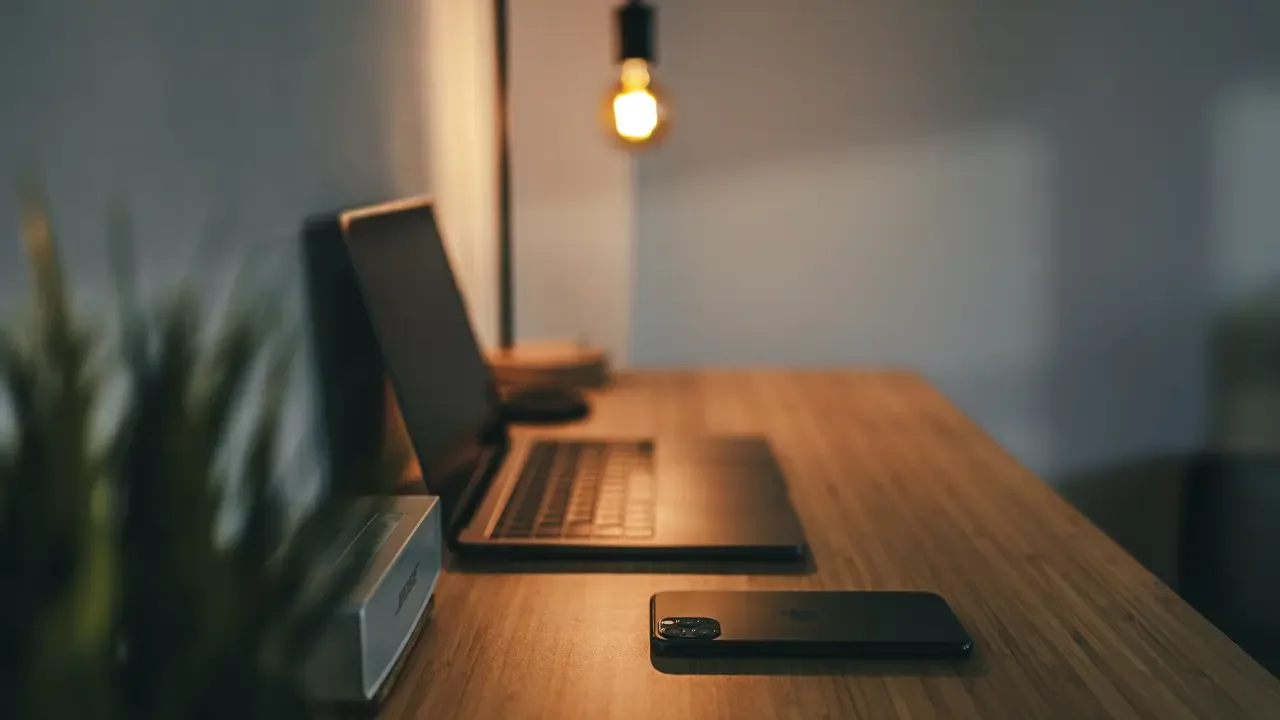
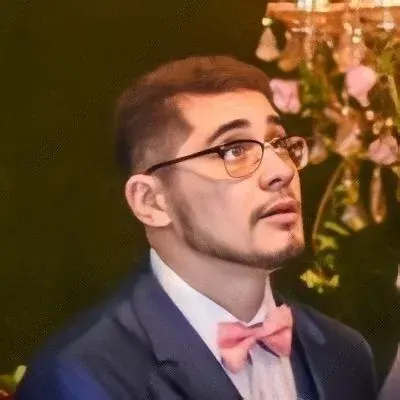
Understanding @classmethod and @staticmethod in Python 🐍
If you're new to Python, you might have come across the terms @classmethod
and @staticmethod
while reading or working on some code. 🤔 They can be a bit confusing, but don't worry! This guide will break it down for you and clarify when and why you should use them. Let's dive in! 💪
What do @classmethod and @staticmethod mean? 🤷♀️🤷♂️
@classmethod
: It is a decorator that defines a method to be a class method. A class method receives the class itself as the first argument, conventionally namedcls
, rather than an instance of the class. This allows the method to access and modify class-level variables and perform operations that are relevant to the class as a whole.@staticmethod
: It is another decorator that defines a method to be a static method. A static method does not receive the class or instance as an argument. It behaves like a regular function but is defined within the class for organization purposes.
When should I use @classmethod and @staticmethod? 🤔
@classmethod
Use @classmethod
when you want a method to be associated with the entire class and any subclass that inherits it. This is useful when the method needs to work with class-level variables or when you need a constructor-like method for the class. For example:
class Car:
fuel_capacity = 50
@classmethod
def get_fuel_capacity(cls):
return cls.fuel_capacity
Here, the get_fuel_capacity
method is defined using @classmethod
to access the fuel_capacity
variable of any subclass of Car
.
@staticmethod
Use @staticmethod
when you have a utility function that doesn't need to access any class-level variables or instance-specific data. It can be called directly from the class without creating an instance. For example:
class MathUtils:
@staticmethod
def multiply(a, b):
return a * b
In this case, the multiply
method is defined using @staticmethod
as it doesn't require any instance-specific data.
💡 Keep in mind that static methods are often used for helper functions or utility methods that are related to the class but don't need access to its internals.
Why should I use @classmethod and @staticmethod? 🤔🌟
Using @classmethod
and @staticmethod
provides some key benefits:
Improved organization: By defining methods as class methods or static methods, you clearly indicate their purpose and relationship to the class.
Code reusability: Class methods can be inherited and overridden by subclasses, ensuring that common behavior is shared across multiple classes.
Encapsulation: Static methods can be used to group related utility functions within a class, making the codebase more organized and easier to maintain.
How should I use @classmethod and @staticmethod? 🛠️👨💻
To use @classmethod
and @staticmethod
, you simply need to define them above the method you want to decorate. Here's an example:
class MyClass:
@classmethod
def my_class_method(cls):
# Do something with cls (class-level variable or operation)
pass
@staticmethod
def my_static_method():
# Do something (no access to cls or self)
pass
Remember that @classmethod
methods receive the class itself as the first argument (cls
convention), while @staticmethod
methods don't receive any special arguments.
Conclusion and Your Turn! ✍️🚀
Now that you understand the meaning of @classmethod
and @staticmethod
in Python, it's time to put this knowledge into action! Start using them in your code to improve organization, increase code reusability, and encapsulate related utility functions. Have fun exploring their possibilities! 🎉
🤝 What other Python concepts or techniques would you like us to cover? Let us know in the comments section below and keep learning together! Happy coding! 💻✨