Manually raising (throwing) an exception in Python
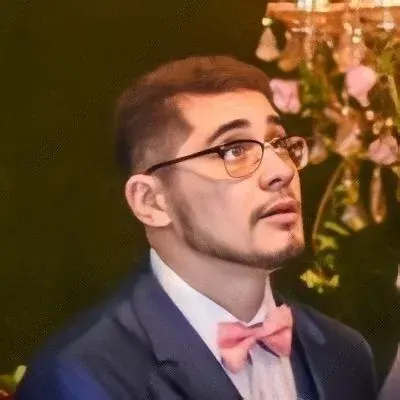
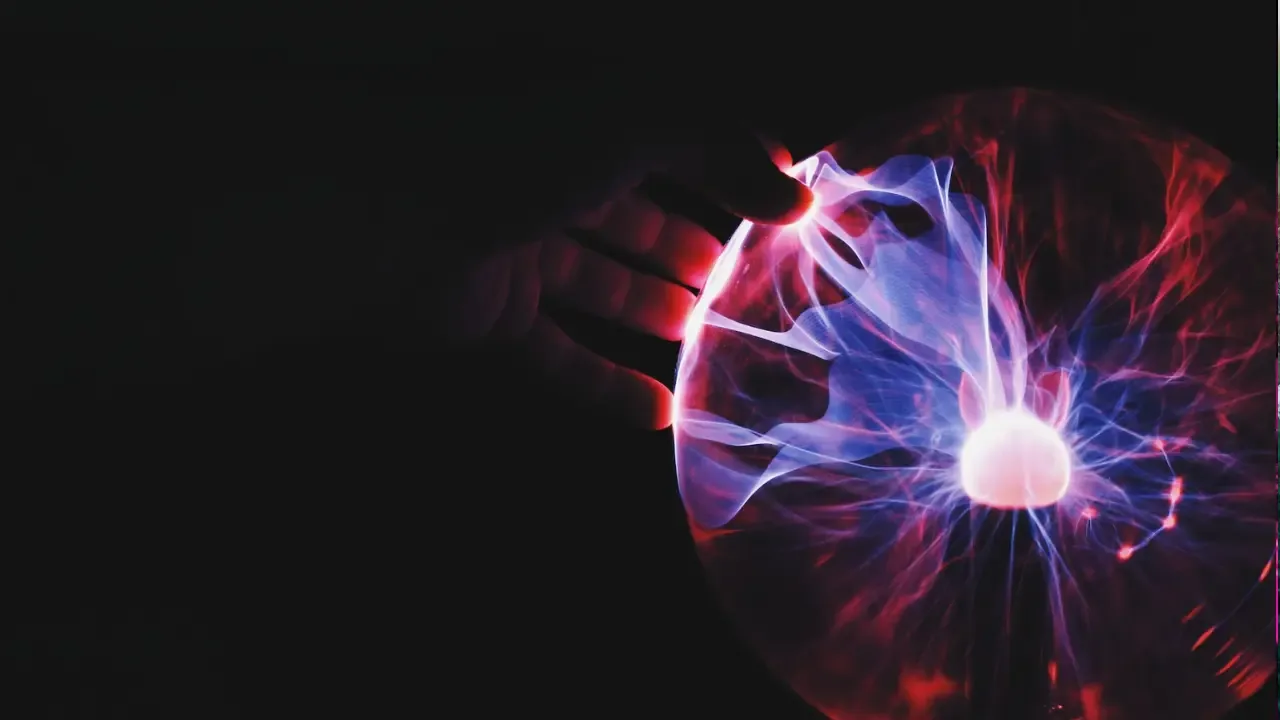
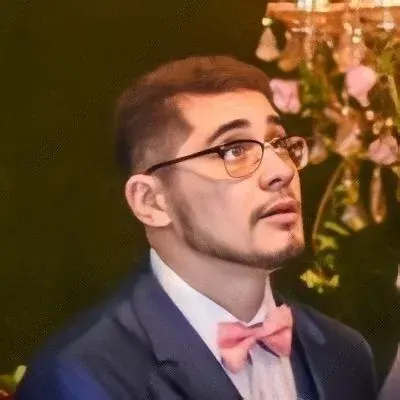
🐍 Manually Raising Exceptions in Python: Mastering the Art 🚀
Have you ever found yourself in a situation where you need to raise an exception in Python and catch it later using an except
block? Fear not, fellow Pythonista! In this guide, we'll explore the ins and outs of manually raising exceptions in Python. Buckle up, because we're about to dive into this exciting topic! 💥
The Problem: Raising Exceptions
Imagine this scenario: You're creating a Python program and you encounter an error condition that requires the program to stop its execution and notify the user about the issue. This is where raising an exception comes in handy.
But how do you go about raising an exception manually in Python? Let's find out! 😎
The Solution: Raising Exceptions like a Pro
In Python, you can raise exceptions using the raise
statement. This statement is followed by an exception type, which indicates the specific kind of error you want to raise. Here's a simple example:
raise ValueError("Oops! Something went wrong.")
In this example, we're raising a ValueError
exception and providing a custom error message. This allows us to communicate to the user what went wrong in a more meaningful way.
But what if you want to raise an exception without an error message? Easy peasy! Just omit the message like this:
raise ValueError
Now that you know the basics, let's explore some common issues and their solutions when manually raising exceptions in Python.
Common Issues and Their Solutions
Issue 1: When to Raise an Exception?
It's important to raise exceptions when you encounter exceptional conditions that prevent normal program execution. Ask yourself, "Is this situation truly abnormal?" If the answer is yes, then go ahead and raise an exception.
Issue 2: Choosing the Right Exception Type
Python provides a wide range of built-in exception types that you can use. It's crucial to choose the appropriate exception type that best fits the error you encountered. For example, if a function receives invalid arguments, you might raise a ValueError
. If a file is not found, you could raise a FileNotFoundError
. It's all about picking the most suitable exception for the job!
Issue 3: Providing Useful Error Messages
When raising an exception, always strive to provide informative error messages. Clearly describe the error condition and include any relevant details that might be useful to the user or programmer who will be handling the exception. A good error message can save hours of debugging time!
Finally, the Call-to-Action: Engage with Us! 🙌
We hope this guide has shed some light on manually raising exceptions in Python. Now it's your turn! Start implementing exception handling in your programs and share your experiences with us in the comments below. Did you encounter any interesting challenges? How did you overcome them? Let's learn and grow together! 🌱
If you found this guide helpful, don't forget to share it with your fellow Python enthusiasts. Spread the knowledge and empower others to become exceptional exception handlers! 😄
Happy coding! 💻