Logical operators for Boolean indexing in Pandas
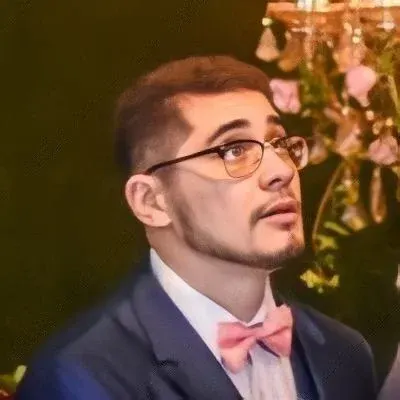
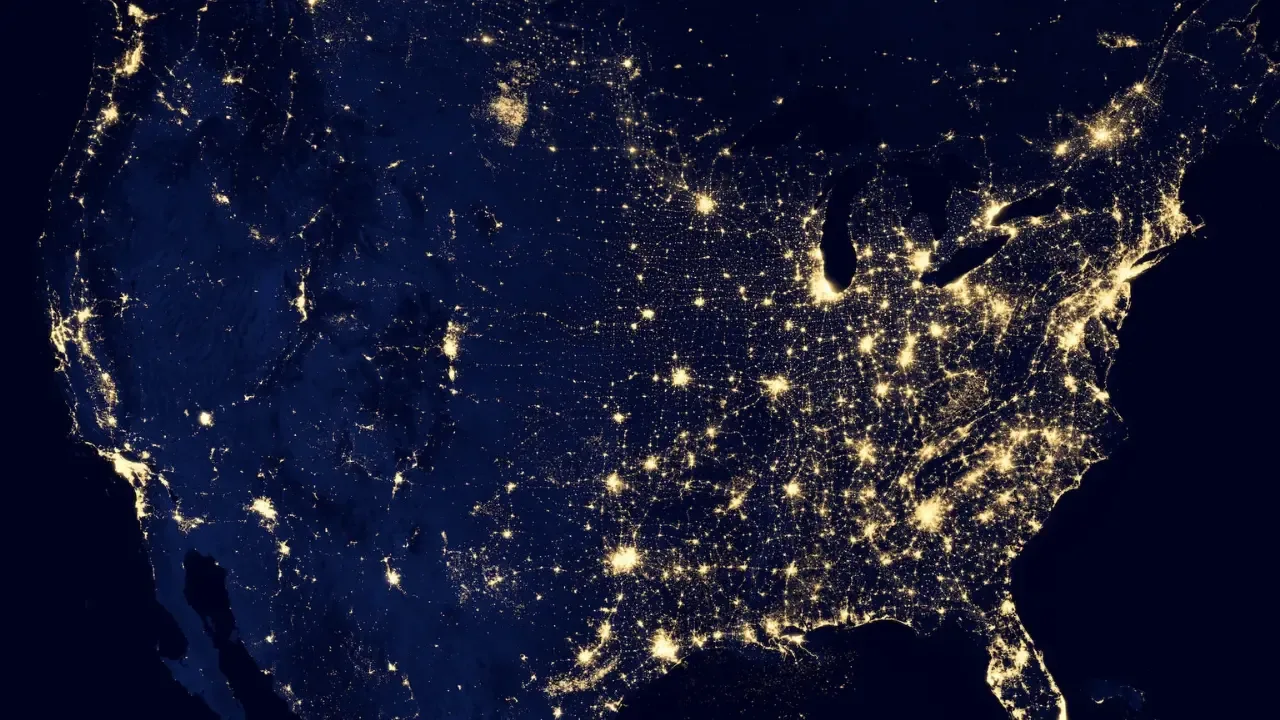
Logical Operators for Boolean Indexing in Pandas: Easy Solutions to Common Issues
If you're working with Boolean indexing in Pandas, you may have come across an error when combining conditions using logical operators. This blog post will address a specific problem and provide easy solutions for it, so you can breeze through your data filtering tasks with confidence. 😎
The Problem
Let's start by taking a look at the problem itself. Consider the following code snippet:
a = pd.DataFrame({'x': [1, 1], 'y': [10, 20]})
You might think that both of the following statements would give you the same result:
a[(a['x'] == 1) & (a['y'] == 10)]
a[(a['x'] == 1) and (a['y'] == 10)] # Throws an error
However, to your surprise, the second statement throws a ValueError
:
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
Understanding the Issue
The reason for this error lies in the usage of the logical operators and
and &
. While they may seem interchangeable, they behave differently in this context.
In the first statement, we use the bitwise logical operator &
to combine the conditions, resulting in a Boolean array. This array is then used to filter the DataFrame a
, giving us the expected output. ✅
In the second statement, we use the and
operator, which expects scalar truth values rather than arrays. Hence, Pandas throws an error because it can't determine the truth value of an array with more than one element. ❌
Easy Solutions
Now that we understand the issue, let's discuss some easy solutions to tackle it. There are two possible approaches:
Using the
&
Operator: Simply replace theand
operator with the&
operator to combine the conditions, just like in the first statement. This will ensure that the conditions are evaluated element-wise and produce the desired Boolean array.a[(a['x'] == 1) & (a['y'] == 10)]
Using Parentheses: If you prefer using the
and
operator or want to avoid confusion, you can wrap each condition within parentheses. By doing this, you'll create separate Boolean arrays for each condition, and then use the&
operator to combine them.a[(a['x'] == 1) and (a['y'] == 10)] # Updated statement
becomes
a[(a['x'] == 1) and (a['y'] == 10)] # Updated statement
This way, each condition is evaluated separately, producing the desired Boolean arrays, which are then combined using the
&
operator.
Call-to-Action
Don't let small syntax errors slow you down! Keep these tips in mind to avoid and resolve similar issues when working with Boolean indexing in Pandas.
If you found this guide helpful, share it with your friends and colleagues who might also benefit from these easy solutions. Comment below if you have any questions or encountered other common issues related to logical operators in Pandas. Let's learn and grow together! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
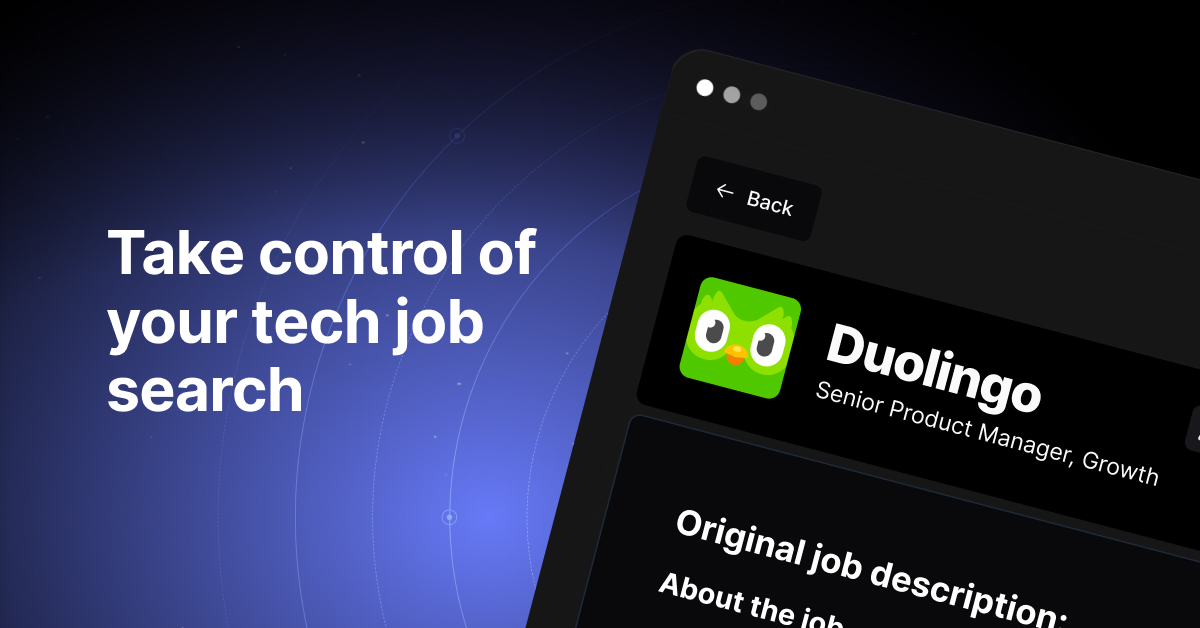