Load data from txt with pandas
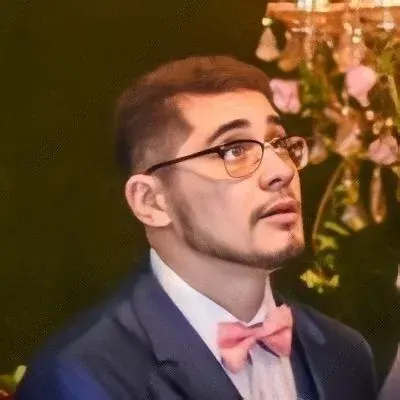
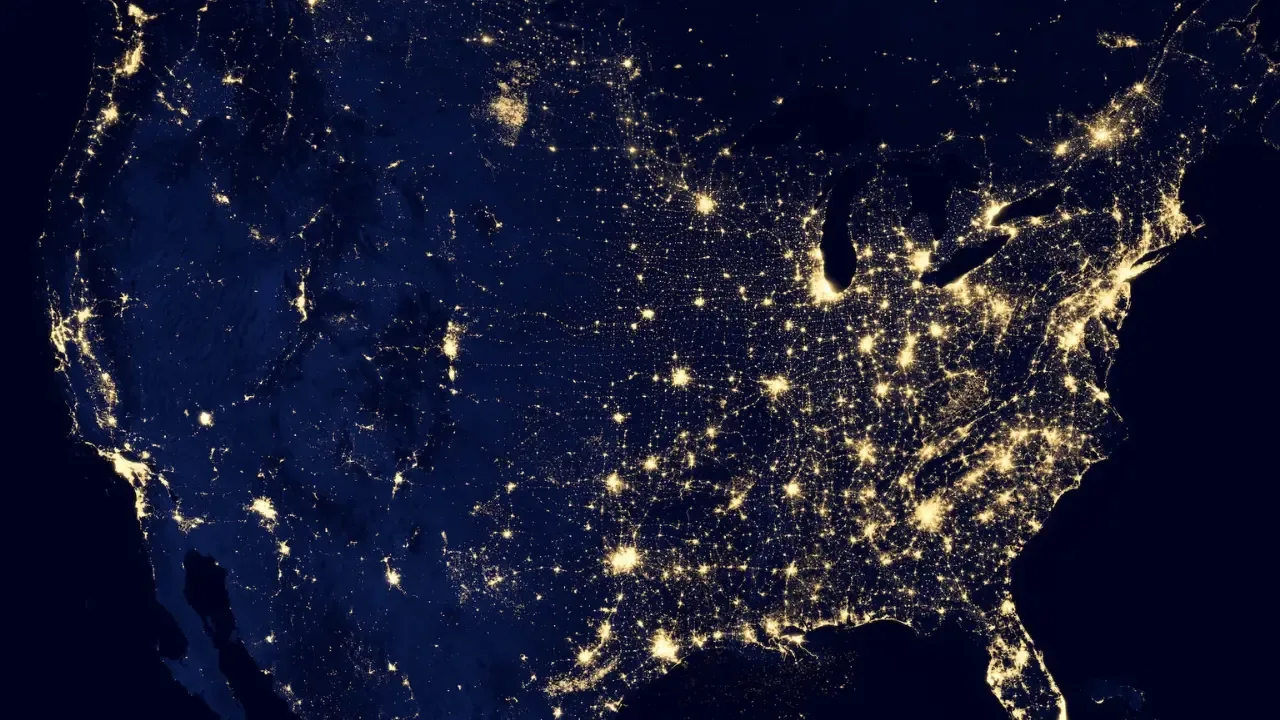
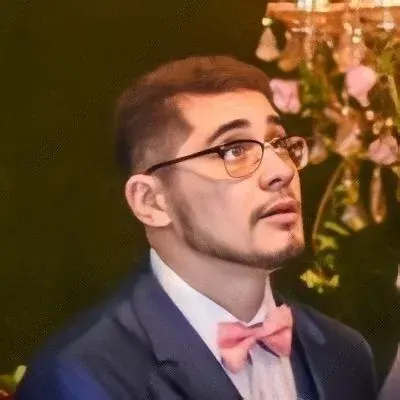
How to Load Data from a TXT File into Pandas
Are you struggling with loading data from a TXT file into pandas? Don't worry, we've got you covered! In this guide, we will address common issues and provide easy solutions to help you efficiently load and access your data.
The Problem
Let's start by understanding the problem at hand. You have a TXT file that contains a mixture of floating-point and string data. Your goal is to store these elements in an array where you can easily access each individual element.
The Initial Solution
Initially, you tried using the pd.read_csv()
function from the pandas library to load the data. Here's what your code looks like:
import pandas as pd
data = pd.read_csv('output_list.txt', header=None)
print(data)
However, this approach resulted in the data being imported as a single column. This is not ideal because you want to store different elements separately so that you can access them using the syntax data[i, j]
. Additionally, you also want to define a header for your data.
The Solution
To tackle this problem, we will make use of additional parameters provided by the pd.read_csv()
function. Let's go through each step of the solution one by one.
Step 1: Load the Data into Separate Columns
To load the data in separate columns, we can specify the delimiter used in the TXT file. In your case, it seems that the columns are separated by spaces. Modify your code as follows:
import pandas as pd
data = pd.read_csv('output_list.txt', header=None, delimiter=' ')
print(data)
By specifying the delimiter
parameter as a space, pandas will recognize the spaces in the TXT file as column separators, resulting in each element being loaded into its respective column.
Step 2: Define a Header
To define a header for your data, you can either provide it in the TXT file itself or specify it in the code. If your TXT file does not contain a header, you can define it using the names
parameter. Modify your code to include the header:
import pandas as pd
header = ['column_1', 'column_2', 'column_3', 'column_4', 'column_5', 'column_6']
data = pd.read_csv('output_list.txt', header=None, delimiter=' ', names=header)
print(data)
Now, each column will have a name corresponding to the headers you provided. You can access individual elements using the syntax data[column_name][row_index]
or data.loc[row_index, column_name]
.
Your Turn!
Give the modified code a try with your own TXT file and see the magic of pandas! Remember to adapt the header names to match your data.
If you found this guide helpful, make sure to share it with your friends who might be facing similar issues. Let's spread the joy of efficient data loading with pandas!
Have any other pandas-related questions or need further assistance? Leave a comment below, and our tech-savvy community will be happy to help you out! 👩💻👨💻