List attributes of an object
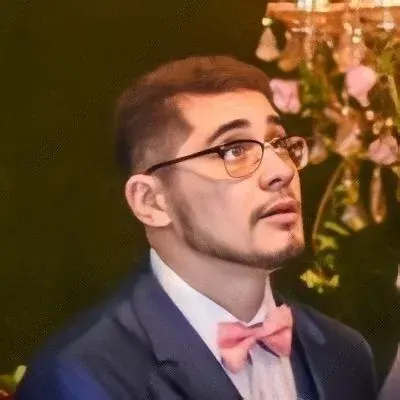
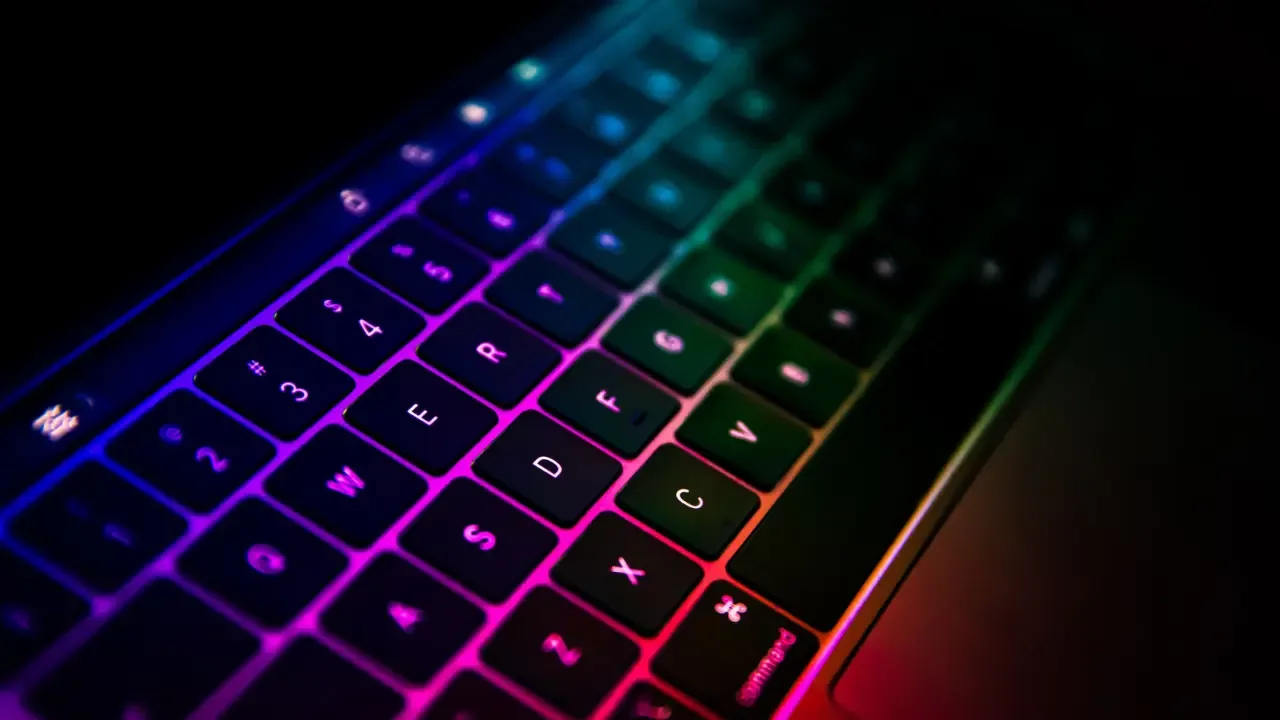
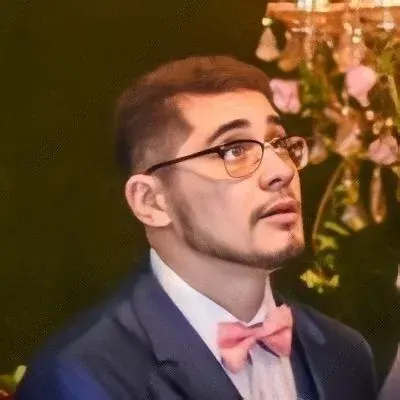
🕵️♀️ The Quest for Object Attributes
So, you find yourself on a mission to grab a list of attributes that exist on instances of a class, huh? 🤔 No worries, fellow explorer! We're here to guide you through this perplexing puzzle and help you uncover the desired results. 🌟
🎯 The Problem
Your mission is to obtain a list of attributes from instances of a class using Python. In other words, you want to extract the attributes "multi" and "str" from the new_class
object, "a".
📝 The Solution
To start, let's take a look at the code snippet you provided:
class new_class():
def __init__(self, number):
self.multi = int(number) * 2
self.str = str(number)
a = new_class(2)
print(', '.join(a.SOMETHING))
In order to retrieve the attributes, we'll use the
dir()
function. This handy function returns a list of valid attributes and methods for an object.
class new_class():
def __init__(self, number):
self.multi = int(number) * 2
self.str = str(number)
a = new_class(2)
attributes_list = dir(a)
print(attributes_list)
Now, if you only want to display the attributes and not the methods, you can filter the list using a simple list comprehension and the
hasattr()
function:
class new_class():
def __init__(self, number):
self.multi = int(number) * 2
self.str = str(number)
a = new_class(2)
attribute_names = [attr for attr in dir(a) if not callable(getattr(a, attr)) and not attr.startswith("__")]
print(", ".join(attribute_names))
💡 Voila! The desired result will now be printed: "multi, str".
🙌 Gettin' Engaged
Did you find this solution helpful? Let us know! We'd love to hear your thoughts and see what other Python challenges you're tackling. Share your experiences and engage with our vibrant community. Together, we can conquer the code! 🎉
Drop a comment below and join the conversation. Remember, sharing is caring. Share this blog post with your fellow code adventurers and help them solve their attribute-fetching quests too. ✨
🚀 Happy coding! 👩💻👨💻