Limiting floats to two decimal points
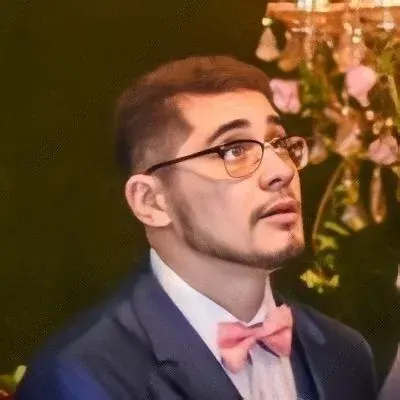
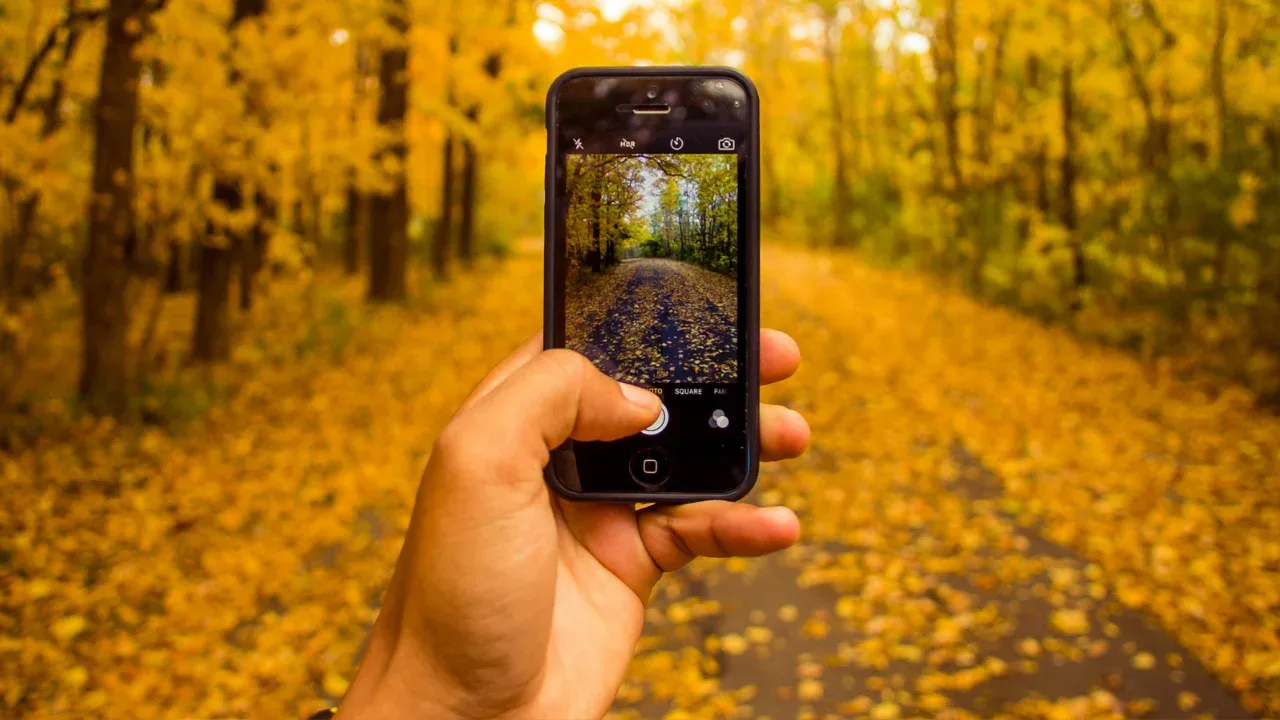
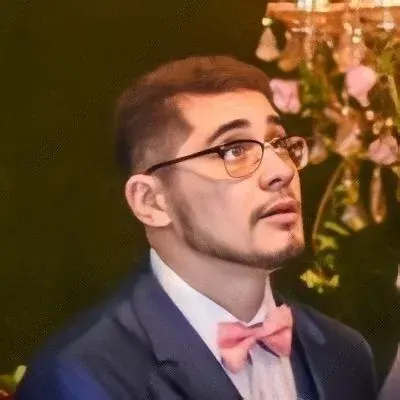
π»π’π― Limiting Floats to Two Decimal Points: Easy Solutions to a Tricky Problem
Are you tired of dealing with those pesky float values that always seem to have a decimal point that just won't quit? π Don't worry, you're not alone! Many programmers have faced this issue and found themselves scratching their heads in frustration. But fear not! π¦ΈββοΈ Today, we have some easy and effective solutions for you to round those floats to two decimal points and save yourself from unnecessary headaches. Let's dive right in! πͺ
The Problem at Hand
So you have a float value, let's call it a
, and you want it to be rounded to two decimal points, specifically to 13.95
. πββοΈ You might naturally turn to the round()
function, a tried and trusted method in Python. But alas, when you give it a shot, here's the result you get:
>>> a
13.949999999999999
>>> round(a, 2)
13.949999999999999
Wait, what just happened? Why is it still giving you all those pesky nines at the end? π
Understanding the Issue
To understand why this is happening, we need to take a quick look at how float values are stored and represented in Python. π Floats are binary fractions, and sometimes, when we convert decimal numbers to binary, there can be slight precision errors. This is exactly what you're seeing in this case.
When you assign 13.95
to a
, the binary representation of a
is inexact and ends up being a value slightly less than 13.95
. Thus, when you pass a
to the round()
function, it operates on that slightly less precise value and, unfortunately, doesn't yield the desired result. π’
Solution 1: Format it Like a Pro
One of the easiest and most straightforward solutions to limit floats to two decimal points is to leverage string formatting in Python. π‘ By using the format()
method or f-strings, you can control the precision of the output without worrying about any precision errors that might arise.
Here's how you can do it:
>>> a = 13.949999999999999
>>> formatted_a = "{:.2f}".format(a)
>>> formatted_a
'13.95'
Tada! π By using the "{:.2f}"
format specifier, we ensure that a
is displayed with exactly two decimal places. No more pesky nines bothering you!
Alternatively, you can also achieve the same result using f-strings, introduced in Python 3.6:
>>> formatted_a = f"{a:.2f}"
>>> formatted_a
'13.95'
Solution 2: Utilize the Decimal Module
If you're working with financial or monetary values, precision is critical, and rounding errors should be avoided at all costs. In such cases, the decimal
module comes to the rescue! π
This module provides the Decimal
class, which offers precise decimal arithmetic. By using the Decimal
class, you can accurately round your floats to two decimal points without any loss of precision. Here's how you can do it:
>>> from decimal import Decimal
>>> a = Decimal('13.949999999999999')
>>> rounded_a = round(a, 2)
>>> rounded_a
Decimal('13.95')
With the Decimal
class, you can rest assured that your calculations will be accurate and free from any unwanted decimal point shenanigans. π―
Time to Put an End to Those Extra Decimals!
Floats with trailing decimal points causing you frustration? π€ With the use of simple and effective solutions, we can limit those floats to two decimal points hassle-free. πͺ To recap:
Format it like a pro using the
"{:.2f}"
format specifier or f-strings.Utilize the
decimal
module and theDecimal
class for precise decimal arithmetic.
Don't let those extra decimals drive you insane! π€― Use these strategies, and you'll be rounding floats like a pro in no time. Happy coding! π
Have you faced issues with floating-point numbers? How did you tackle them? Share your experiences and let's round up the conversation! π