Let JSON object accept bytes or let urlopen output strings
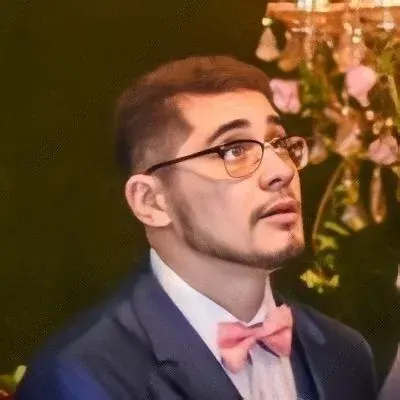
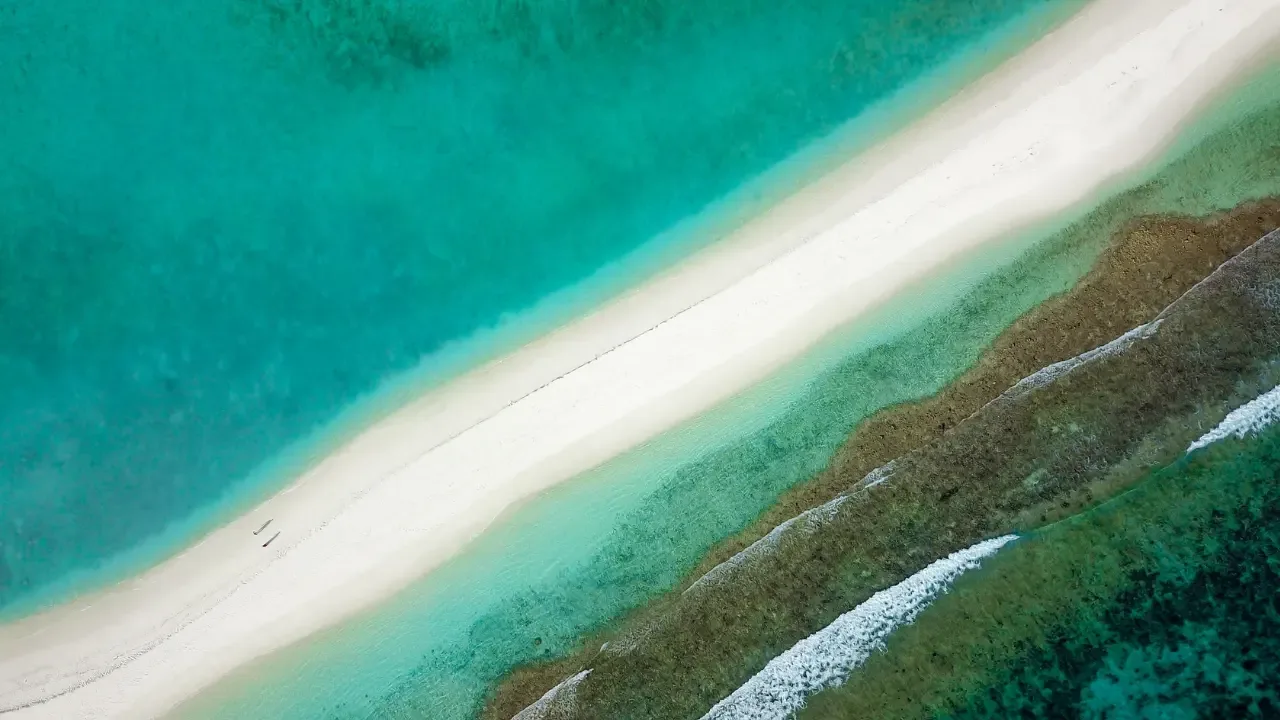
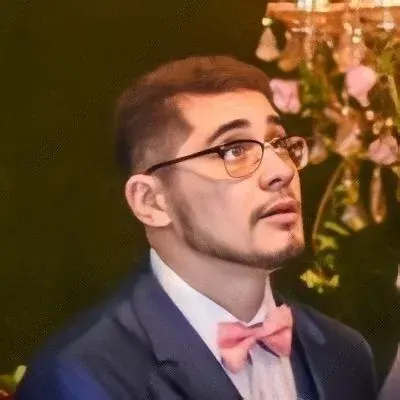
Title: JSON and Bytes: A Simple Solution 💡
Welcome to my tech blog! Today, we're going to tackle a common issue that Python developers face when working with JSON and bytes. 🐍
The Problem 🤔
With Python 3, requesting a JSON document from a URL is as easy as:
response = urllib.request.urlopen(request)
However, things start to get tricky when we want to directly load the response into a JSON object using json.load()
. Normally, to create a JSON object, we can use a file opened in text mode:
obj = json.load(fp)
But in this case, the response
object returned by urlopen
is a file-like object in binary mode, which can't be directly passed to json.load()
.
The Workaround 🛠️
No worries! We've got a simple workaround that will help you transform the bytes file object into a string file object. 💪
str_response = response.read().decode('utf-8')
obj = json.loads(str_response)
By using response.read().decode('utf-8')
, we convert the bytes returned by response.read()
into a string. Then, we can use json.loads()
to load the string as a JSON object.
A Better Way? 🚀
You might be wondering if there's a better solution or if you're missing any parameters for urlopen
or json.load
to handle the encoding automatically. Unfortunately, there isn't a direct option to handle this scenario.
The workaround we provided earlier is the most commonly used solution. While it might feel a bit clunky, it gets the job done without any major roadblocks.
Conclusion and Call-to-Action 📝
Handling JSON and bytes in Python doesn't have to be a headache! With a simple workaround, you can seamlessly transform bytes file objects into string file objects and load them as JSON. Remember, though it may feel a bit odd, it's a proven solution that many developers rely on.
If you found this blog post helpful, be sure to share it with your fellow Pythonistas! And feel free to leave a comment if you have any other tips or tricks to handle similar situations.
Happy coding! 👩💻👨💻