"Least Astonishment" and the Mutable Default Argument
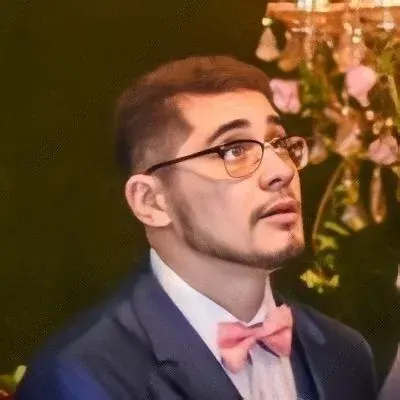
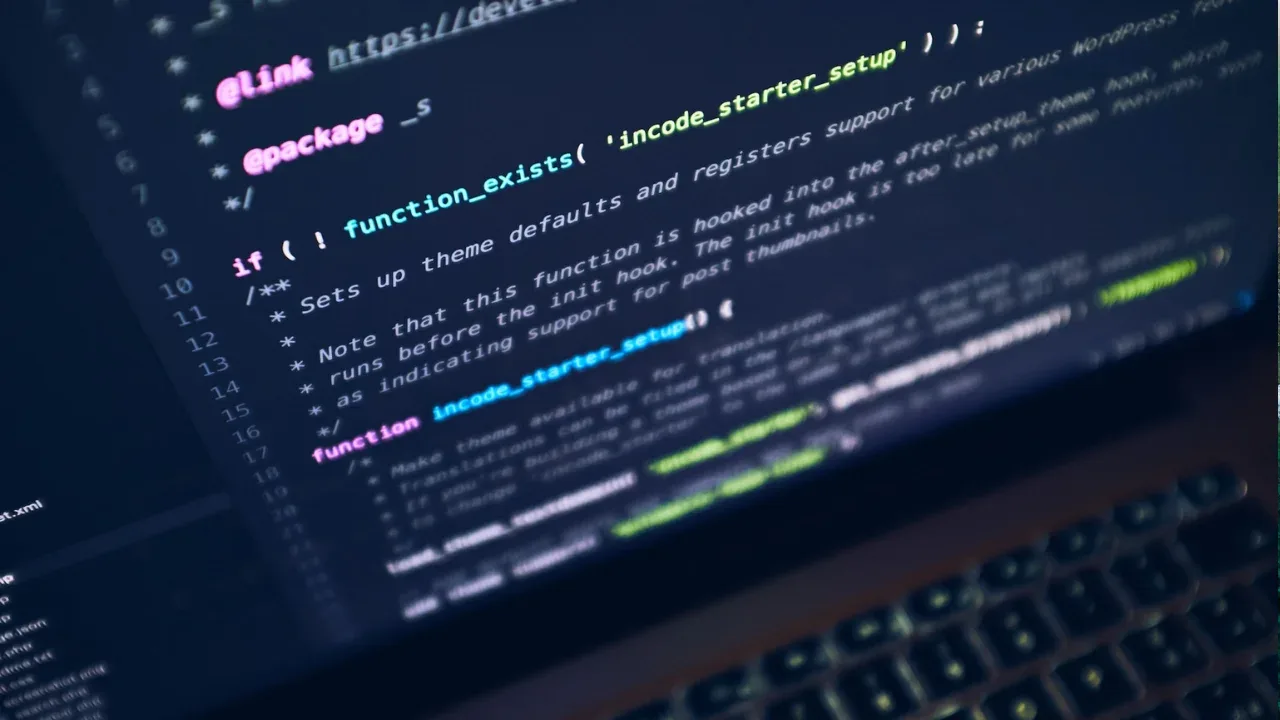
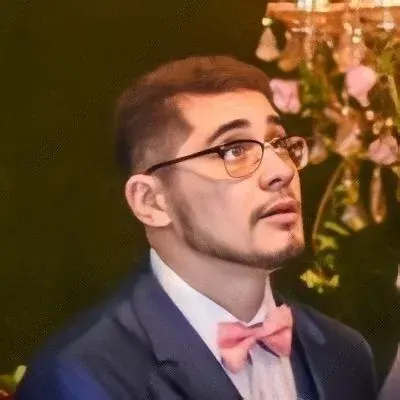
The Astonishing Mystery of Mutable Default Arguments in Python 🤔🐍
Anyone who has dabbled in Python for long enough has probably encountered the perplexing issue of mutable default arguments. It can leave novices scratching their heads and questioning the integrity of the language.
Consider the following code snippet:
def foo(a=[]):
a.append(5)
return a
At first glance, one might expect this function to always return a list with just one element: [5]
. However, the actual result is quite different and surprising:
>>> foo()
[5]
>>> foo()
[5, 5]
>>> foo()
[5, 5, 5]
>>> foo()
[5, 5, 5, 5]
🔍 The Curious Case of Mutable Default Arguments
This behavior can be coined as "least astonishment", a principle in software design that aims to minimize surprise or confusion among programmers. But in this case, it seems that Python's implementation deviates from this principle.
To shed some light on this issue, let's answer the burning question: why are default arguments bound at function definition rather than at function execution? 🤔
🕵️♀️ Delving into the Depths of Default Arguments
The reason lies in how Python handles function invocation and object references. When a function is defined, all its default values are evaluated and assigned. In the example above, the default value a=[]
is evaluated only once during function definition.
This means that subsequent invocations of the foo()
function will continue to use the same mutable list object, a
, from the previous invocation. Hence, the list keeps growing with each call, resulting in the unexpected behavior we observed.
🔧 Solutions to the Mutable Default Argument Pitfall
Now that we understand the root cause of this issue, we can explore some strategies to avoid falling into this mutable default argument trap.
Solution 1: Use None
as the Default Argument
def foo(a=None):
if a is None:
a = []
a.append(5)
return a
By using None
as the default argument and checking for None
within the function, we ensure that a new list is created for each call to foo()
. This prevents the unwanted accumulation of elements in the list.
Solution 2: Utilize Keyword Arguments
def foo(a=[]):
a.append(5)
return a
>>> foo()
[5]
>>> foo(a=[])
[5]
Explicitly passing an empty list as a keyword argument will result in a fresh list being used, instead of the mutable default argument. This approach provides a clear, visual indication that a new list is being used.
📣 Spread the Word and Engage!
Now that you know the secrets behind mutable default arguments in Python, don't keep it to yourself! Share this knowledge with your fellow Pythonistas and save them from potential surprises.
💬 What other Python features have left you puzzled? Share your thoughts in the comments below and let's unravel those mysteries together! 💡💭
👉 If you enjoyed this article, don't forget to subscribe to our newsletter for more fascinating insights and helpful tips on Python and other tech topics. Happy coding! 🎉🐍
Disclaimer: Python is full of surprises, but that's what makes it fun! Embrace the quirks and learn from them. 😉🤓