"Large data" workflows using pandas
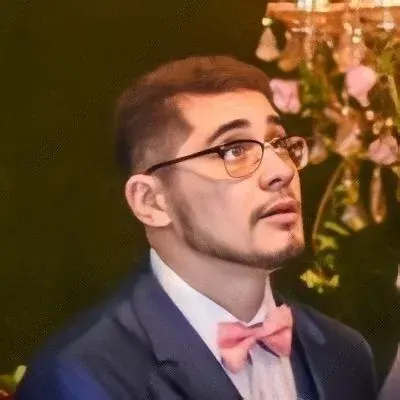
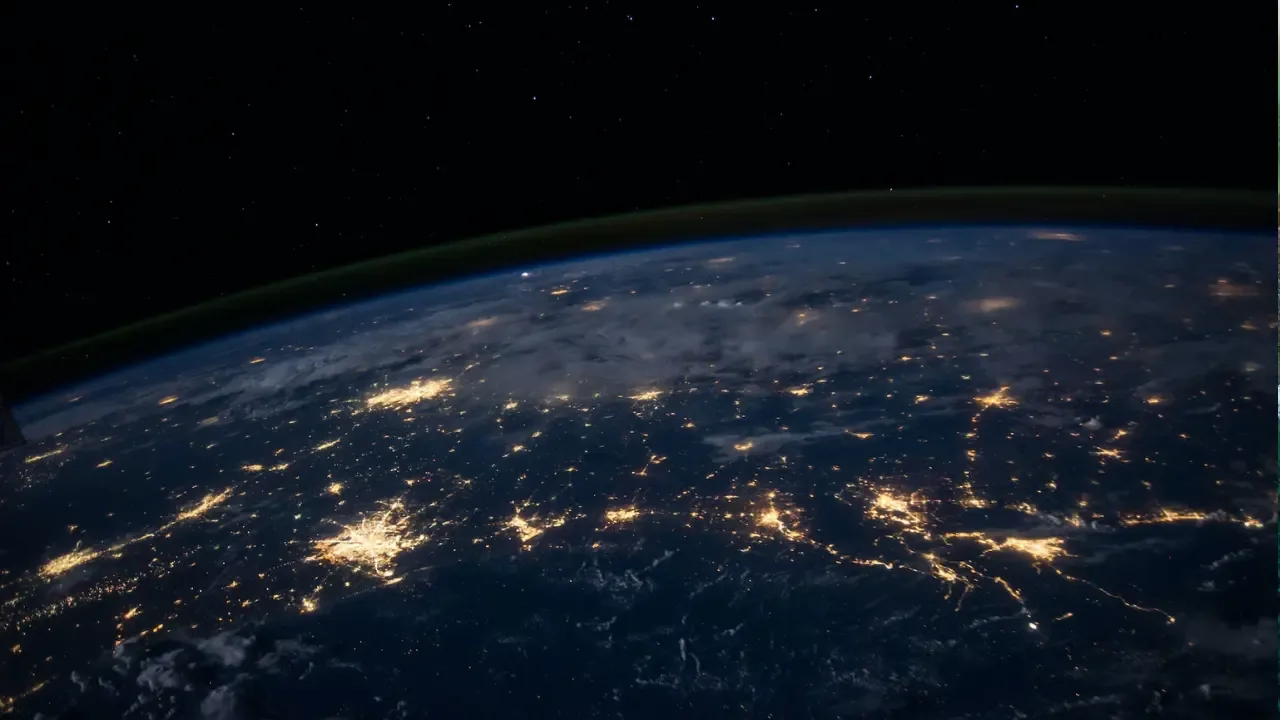
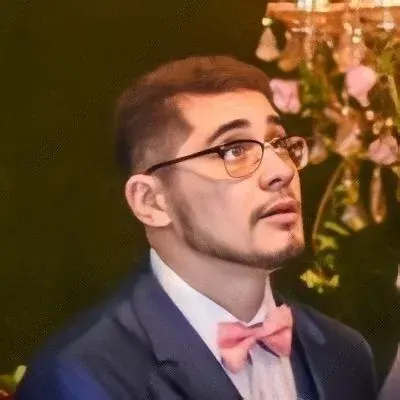
🐼 Large Data Workflows using Pandas: Best Practices and Solutions
Are you tired of struggling with large datasets that are too big to fit in memory but too small to require a distributed network? Do you want to replace your use of SAS with Python and Pandas, but lack an out-of-core workflow for large datasets? We've got you covered! In this blog post, we'll dive into best-practice workflows for loading, querying, and updating large datasets using Pandas.
The Challenge: Large Datasets that Don't Fit in Memory
One approach to handling large datasets in Pandas is to use the HDFStore
to hold the data on disk and pull only the pieces you need into dataframes for analysis. Another alternative that has been mentioned is MongoDB. However, the question remains: what are the best-practice workflows for accomplishing the following tasks with large datasets?
Loading flat files into a permanent, on-disk database structure
Querying the database to retrieve data for analysis in Pandas
Updating the database after manipulating data in Pandas
To help us understand the problem better, let's look at an example provided by the original post:
Importing Large Flat Files: Iteratively import a large flat-file and store it in a permanent, on-disk database structure. These files are typically too large to fit in memory.
Selecting Subset of Data: Read subsets of the data, usually a few columns at a time, that can fit in memory for analysis in Pandas.
Creating New Columns: Perform various operations on the selected columns to create new columns.
Appending New Columns: Append the new columns into the on-disk database structure.
Best-Practice Workflows for Large Data in Pandas
Now let's explore some best-practice solutions for each of these steps:
1. Loading Flat Files into a Database
To load flat files into a permanent, on-disk database structure, consider using the read_csv()
function from Pandas. This function allows you to read large flat files in chunks, avoiding memory constraints. You can then store the data in a database using libraries such as SQLAlchemy or the built-in SQLite support in Pandas.
Here's an example of how to read and store a large flat file in chunks:
import pandas as pd
# Read the large file in chunks
chunk_size = 100000
df_chunks = pd.read_csv('large_file.csv', chunksize=chunk_size)
# Store the chunks in a SQLite database
database_name = 'large_data.db'
conn = sqlite3.connect(database_name)
for df_chunk in df_chunks:
df_chunk.to_sql('my_table', conn, if_exists='append', index=False)
conn.close()
2. Querying the Database for Analysis
After loading the data into the database, you can query it to retrieve subsets of data for analysis in Pandas. You can utilize SQL queries or Pandas' own querying capabilities to extract the desired data.
Here's an example of how to query the database using SQL and convert the result into a Pandas dataframe:
import pandas as pd
import sqlite3
# Connect to the database
database_name = 'large_data.db'
conn = sqlite3.connect(database_name)
# Query the database using SQL
query = "SELECT column1, column2 FROM my_table WHERE condition"
df_query = pd.read_sql_query(query, conn)
# Close the connection
conn.close()
3. Updating the Database After Manipulating Data
After performing data manipulation and analysis in Pandas, you may want to update the database with the changes you made. To achieve this, you can create new columns in a dataframe and then append them to the existing table in the database.
Here's an example of how to append new columns to the existing table in the database:
import pandas as pd
import sqlite3
# Connect to the database
database_name = 'large_data.db'
conn = sqlite3.connect(database_name)
# Read the existing table into a dataframe
df_existing = pd.read_sql_query("SELECT * FROM my_table", conn)
# Perform operations to create new columns in Pandas
df_existing['new_column'] = ...
# Append the new columns to the table in the database
df_existing.to_sql('my_table', conn, if_exists='replace', index=False)
# Close the connection
conn.close()
Real-World Examples: Tackling "Large Data" with Pandas
To fully understand how these best practices can be applied in real-world scenarios, let's take a look at some examples provided by the original post:
Consumer Credit Risk Models: In this scenario, the datasets have nearly 1,000 to 2,000 fields on average of mixed data types. Instead of appending rows, various operations are performed to create new columns that describe relationships with an outcome variable.
Exploring Data in Small Sets: To create candidate variables, the user explores columns in small sets. For example, focusing on properties values and observing their relationship to loan defaulting, followed by exploring another group of columns (e.g., college education).
By following the best practices outlined above, you can effectively handle large datasets in Pandas, perform operations to create new columns, and update your database as needed.
Join the Large Data Revolution with Pandas!
Don't let large datasets intimidate you anymore! Make the switch from SAS to Python and Pandas, and discover the power of handling "large data" with ease. Start using the best-practice workflows we've covered in this blog post and take control of your data analysis journey today!
🚀 Share your experiences with large datasets in Pandas in the comments below. Let's revolutionize "large data" together! 💪